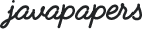
Text to speech is becoming an integral part of many content driven mobile APPs. If your APP is a tutorial or content heavy, then it is high time you think about adding the Text-To-Speech (TTS) feature to it. Voice input based command and text to speech is a trend now. In this tutorial, we will explore how “Text-To-Speech(TTS)” feature can be implemented in an Android APP.
Text to speech (TTS) makes an android device read the text and convert it to audio out via the speaker. Android TTS supports multiple languages. TTS is a simple but powerful feature. It can also be effectively used in mobile APPs dedicated to visually impaired people or in educational app for kids or can be used in pronunciation learning app, etc. These are some of the ways you can use TTS. Using TextToSpeech enhances interaction between the user and the mobile application.
Android TTS was available from version 1.6. It has features to control speed, pitch of speech as well as type of language. We will also learn to use these features in this tutorial.
In order to learn how it works, we will build a simple Andriod App with a EditText and a button and so that the user can input text. On click of a button the Android APP will convert the text to speech (audio).
Let’s start by designing the layout of our example Android App.
I have kept the layout as simple. It has a LinearLayout with vertical orientation. There are two widgets. First is a an EditText which will receive text input from the user. Second one is the button on click of which application reads the input text entered in EditText and calls the Android TTS API to convert the text to speech.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:orientation="vertical" android:paddingLeft="20dp" android:paddingRight="20dp" tools:context=".MainActivity"> <TextView android:id="@+id/textView" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="50dp" android:gravity="center_horizontal" android:text="Android Text to Speech (TTS) Demo" android:textAppearance="@style/TextAppearance.AppCompat.Headline" /> <EditText android:id="@+id/et" android:layout_width="match_parent" android:layout_height="wrap_content" android:background="@drawable/edit_text_style" android:hint="Enter the text here" android:paddingBottom="10dp" android:paddingLeft="10dp" android:paddingRight="10dp" android:paddingTop="10dp" /> <Button android:id="@+id/btn" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginVertical="10dp" android:elevation="0dp" android:paddingHorizontal="30dp" android:text="Click to convert text to speech" /> </LinearLayout>
We have to create an object of TextToSpeech class and this is the Android class that does the job of converting the text to speech and also provides parameters to set the language, speed and pitch.
Method textToSpeech.setLanguage() is used to set the language of the speech. Many languages are supported by Android TTS engine. In the current example Android app we will use english(United States). To set speed of speech or speed rate textToSpeech.setSpeedRate() method is used. It’s default value is 1. User can set the value according to his need
Setting up the pitch of speech is similar to setting up speed rate described above. Here, textToSpeech.setPitch() method is used. You can also control the speech volume by setting the parameter params.put(TextToSpeech.Engine.KEY_PARAM_VOLUME, “0.1”);
After setting up the speech parameters, we have to invoke OnClickListener when button is clicked. Inside the listener, we will convert user input into string. textToSpeech.speak() method does the main job of the text. It is good practice to shutdown the Android TTS engine once it’s task is over. To accomplish this onDestroy() method is used.
package com.javapapers.texttospeechdemo; import android.speech.tts.TextToSpeech; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.Toast; import java.util.Locale; public class MainActivity extends AppCompatActivity { private TextToSpeech textToSpeech; private Button btn; private EditText editText; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); btn = (Button) findViewById(R.id.btn); editText = (EditText) findViewById(R.id.et); textToSpeech = new TextToSpeech(getApplicationContext(), new TextToSpeech.OnInitListener() { @Override public void onInit(int status) { if (status == TextToSpeech.SUCCESS) { int ttsLang = textToSpeech.setLanguage(Locale.US); if (ttsLang == TextToSpeech.LANG_MISSING_DATA || ttsLang == TextToSpeech.LANG_NOT_SUPPORTED) { Log.e("TTS", "The Language is not supported!"); } else { Log.i("TTS", "Language Supported."); } Log.i("TTS", "Initialization success."); } else { Toast.makeText(getApplicationContext(), "TTS Initialization failed!", Toast.LENGTH_SHORT).show(); } } }); btn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View arg0) { String data = editText.getText().toString(); Log.i("TTS", "button clicked: " + data); int speechStatus = textToSpeech.speak(data, TextToSpeech.QUEUE_FLUSH, null); if (speechStatus == TextToSpeech.ERROR) { Log.e("TTS", "Error in converting Text to Speech!"); } } }); } @Override public void onDestroy() { super.onDestroy(); if (textToSpeech != null) { textToSpeech.stop(); textToSpeech.shutdown(); } } }
We are done! Now run and demo the Android app and convert your text to speech.
Download Android Text To Speech Demo Application Project
Comments are closed for "Android Text To Speech Tutorial".
Thank you so much. for me it was never that much easy before
Welcome Firoz.
Great tutorial. Will try it.