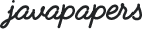
Captcha is used to distinguish human from automated bots. Google provides a captcha API named Google reCAPTCHA as a free service. It is the most popular captcha service available in the Internet. In june 2017, Google rolled out Android reCAPTCHA API. It is a part of SafteyNet services. It is effective in preventing bots from engaging in abusive activities on your app.
Android reCAPTCHA uses adaptive CAPTCHAs and an advanced risk analysis engine which automatically distinguish between human and bot. It doesn’t always require user to read distorted text or solve a simple problem.
In this tutorial i will guide you to integrate and use Android reCAPTCHA in an android APP. We will build a demo Android APP, in which user is required to click a button to proceed. Google Android reCAPTCHA service will respond to the click. You will be prompted to new activity once verification is successful. I recommend you to check the Google reCAPTCHA implementation in Java.
We need to integrate SafetyNet reCAPTCHA API using reCAPTCHA key pair. To register a key pair, follow these steps:-
You will get promoted to new page. Now save the site key and site Secret.
We also need to add safetynet api dependency and enable google play services in our project. To do that, open build.gradle module file and add the following code
compile 'com.google.android.gms:play-services-base:11.0.1' compile 'com.google.android.gms:play-services-basement:11.0.1' compile 'com.google.android.gms:play-services-tasks:11.0.1' compile 'com.google.android.gms:play-services-safetynet:11.0.1'
Added internet permission in the Android Manifest file.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.javapapers.googlerecaptatutorial"> <uses-permission android:name="android.permission.INTERNET" /> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name=".MainActivity2"></activity> </application> </manifest>
Our layout will only have a button.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_gravity="center" android:padding="10dp" tools:context="com.javapapers.googlerecaptatutorial.MainActivity"> <Button android:id="@+id/button" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_centerVertical="true" android:clickable="true" android:onClick="onClick" android:padding="20dp" android:text="Are you Human?" android:textSize="25dp" /> </RelativeLayout>
Create a new activity and name it MainActivity2.
This activity has only a textview containing a dummy text.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_gravity="center" tools:context="com.javapapers.googlerecaptatutorial.MainActivity2"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_centerVertical="true" android:gravity="center" android:padding="20dp" android:text="Verified" android:textSize="25dp" /> </RelativeLayout>
Here, GoogleApiClient is used for Google play integration. Code inside on click method is used for verify the the action with recaptcha. The onResult method return the result form the api. Inside onResult method, intent is used to start next activity once verification is successful. Otherwise it will throw an error message.
package com.javapapers.googlerecaptatutorial; import android.content.Intent; import android.support.annotation.NonNull; import android.support.annotation.Nullable; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.Toast; import com.google.android.gms.common.ConnectionResult; import com.google.android.gms.common.api.GoogleApiClient; import com.google.android.gms.common.api.ResultCallback; import com.google.android.gms.common.api.Status; import com.google.android.gms.safetynet.SafetyNet; import com.google.android.gms.safetynet.SafetyNetApi; public class MainActivity extends AppCompatActivity implements GoogleApiClient.ConnectionCallbacks, GoogleApiClient.OnConnectionFailedListener { Button btnRequest; public final String SiteKey = "6LdwxD8UAAAAAGBNbiw0GF4E_8sopV1ZtfnHDcYt"; public final String SiteSecretKey = "6LdwxD8UAAAAAPosHviHJnwwZ7lviwr6CFZ1D_MX"; private GoogleApiClient mGoogleApiClient; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); btnRequest= findViewById(R.id.button); mGoogleApiClient = new GoogleApiClient.Builder(this) .addApi(SafetyNet.API) .addConnectionCallbacks(MainActivity.this) .addOnConnectionFailedListener(MainActivity.this) .build(); mGoogleApiClient.connect(); } public void onClick(View v){ SafetyNet.SafetyNetApi.verifyWithRecaptcha(mGoogleApiClient, SiteKey) .setResultCallback(new ResultCallback() { @Override public void onResult(SafetyNetApi.RecaptchaTokenResult result) { Status status = result.getStatus(); if ((status != null) && status.isSuccess()) { Toast.makeText(MainActivity.this, "Verification Successful", Toast.LENGTH_SHORT).show();; Intent i =new Intent(MainActivity.this,MainActivity2.class); startActivity(i); } else { Log.e("MY_APP_TAG", "Error occurred " + "when communicating with the reCAPTCHA service.");} } }); }; @Override public void onConnected(@Nullable Bundle bundle) { Toast.makeText(this, "onConnected()", Toast.LENGTH_LONG).show(); } @Override public void onConnectionSuspended(int i) { Toast.makeText(this, "onConnectionSuspended: " + i, Toast.LENGTH_LONG).show(); } @Override public void onConnectionFailed(@NonNull ConnectionResult connectionResult) { Toast.makeText(this, "onConnectionFailed():\n" + connectionResult.getErrorMessage(), Toast.LENGTH_LONG).show(); } }
Now run the example Android APP.
Android reCAPTCHA Google API Integration APP
Comments are closed for "Android ReCAPTCHA Google API Integration".
very nice explain