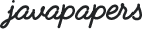
reCAPTCHA is the popular CAPTCHA tool that allows to embed a CAPTCHA in UI and validate the input to block automated spammers. reCAPTCHA provides a Java library and a easy way to implement the CAPTCHA. CAPTCHA stands for “Completely Automated Public Turing test to tell Computers and Humans Apart”.
reCAPTCHA uses a vast library of words for CAPTCHA, it will connect to its API at runtime. This is not a standalone tool and it requires Internet access to work. There are many attempts to develop a fool proof CAPTCHA system and reCAPTCHA has proven to be one the best solution that is difficult to break. The best known use for CAPTCHA is to guard against spams and in some cases we can protect lower layers (example: DB layer) from denial of service (DOS) attacks.
Hackers have claimed and proved success of breaking the CAPTCHA algorithm many times. One side CAPTCHA tools are getting better day by day and other side hackers also breaking the CAPTCHA frequently. We can generate CAPTCHA using Java 2D API with custom generated words, but that has not proven to be strong enough.
In this how to tutorial, let us see how we can use reCAPTCHA to safeguard our Java application. This is the simplest it can get.
This is the first page where we display the generated CAPTCHA image and get input from the user. We can also do this using JavaScript directly.
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <%@ page import="net.tanesha.recaptcha.ReCaptcha" %> <%@ page import="net.tanesha.recaptcha.ReCaptchaFactory" %> <html> <head> <title>CAPTCHA in Java using reCAPTCHA</title> </head> <body> <h2>CAPTCHA in Java Application using reCAPTCHA</h2> <form action="./validate.jsp" method="post"> <p>Username: <input type="text" name="user"></p> <p>Password: <input type="password" name="password"></p> <p> <% ReCaptcha c = ReCaptchaFactory.newReCaptcha( "6LdlHOsSAAAAAM8ypy8W2KXvgMtY2dFsiQT3HVq-", "6LdlHOsSAAAAACe2WYaGCjU2sc95EZqCI9wLcLXY", false); out.print(c.createRecaptchaHtml(null, null)); %> <input type="submit" value="submit" /> </p> </form> </body> </html>
Second page where we get the user input, user’s IP address and other required parameters, to pass on to the reCAPTCHA server for input validation. reCAPTCHA server validates the input and returns true or false.
<%@ page import="net.tanesha.recaptcha.ReCaptchaImpl"%> <%@ page import="net.tanesha.recaptcha.ReCaptchaResponse"%> <html> <head> <title>CAPTCHA in Java using reCAPTCHA</title> </head> <body> <h2>CAPTCHA in Java Application using reCAPTCHA</h2> <p> <% String remoteAddr = request.getRemoteAddr(); ReCaptchaImpl reCaptcha = new ReCaptchaImpl(); reCaptcha.setPrivateKey("6LdlHOsSAAAAACe2WYaGCjU2sc95EZqCI9wLcLXY"); String challenge = request .getParameter("recaptcha_challenge_field"); String uresponse = request.getParameter("recaptcha_response_field"); ReCaptchaResponse reCaptchaResponse = reCaptcha.checkAnswer( remoteAddr, challenge, uresponse); if (reCaptchaResponse.isValid()) { String user = request .getParameter("user"); out.print("CAPTCHA Validation Success! User "+user+" registered."); } else { out.print("CAPTCHA Validation Failed! Try Again."); } %> </p> <a href=".">Home</a> </body> </html>
Download Example: Java Web Application using ReCAPTCHA
Comments are closed for "CAPTCHA in Java using reCAPTCHA".
Good :)
This is what i am searching, it’s simply blast.
Nice article… esp the snippet on digitizing books.
Good article..
hi joe,
I have posted a comment few days ago about this captcha and thanks for explaining in detail.Nice one!!!
Nice one.It will help me out in project
Nice Article
Nice sir…
Please provide example to access recaptcha via proxy and also how to fetch the recaptcha image via proxy validation.
how to use captcha in android
I will write a tutorial for reading captcha in Android soon.
i want to make simple captcha without using recaptcha
Dear sir,
you didn’t mansion the name of the second page.
Second how to use this with java script ?
I have a signup.jsp form in which I am validating all the fields onsubmit using a signup.js javascript file, now I want to use this CAPTCHA at the end of my jsp page- signup.jsp.
So how to use this with javascript.
Thank you
application us working absolutely fine but .. the image box appearing is very big .can we resize it?
any suggestions please
It is working excellently. Could you let me know is there any way of removing “Privacy & Terms” from the widget as needed to our business req.
very nice sir.this is what i search for
Thanx alot. I was searching for 1-2 days and found this blog.
This article helped me alot and is working for me correctly. Thnx again!!!!.