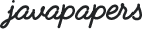
In this article we will learn to add intro slider to an Android App. We will also learn to make a splash screen in android. Intro Slider is the first screen in an APP that is used to display the highlights of application during the first launch of the app.
We will start by adding splash screen in our APP first and then followed by Intro Slider. Splash screen is the first thing that the user sees when app is launched. It is timed and user is directed to the home activity after that. During the splash screen display we can do background processing like loading data for that user etc.
We will make an Intro slider and splash screen for a demo camera app. We will use Viewpager Class and a Viewpager adapter to display Slides of Intro slider.
We will also use PreferenceManager class which is used to help create preference hierarchies from activities or XML. We will use run() method and will call it a thread to time our splash screen.
This example Android application is made to be simple as possible and you can use this as a base framework and can be enhanced and edited according to the need. I have used three screens. You may add more if required.
I have added an icon using ImageView and app title using TextView.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#e78129"> <ImageView android:id="@+id/imageView2" android:layout_width="150dp" android:layout_height="150dp" android:layout_centerHorizontal="true" android:layout_centerVertical="true" android:src="@drawable/javapapers" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/imageView2" android:layout_centerHorizontal="true" android:layout_marginTop="48dp" android:text="Demo App" android:textColor="#000000" android:textSize="40dp" /> </RelativeLayout>
Here, run() method is used and is passed in a thread. Sleep(time in milliseconds) is used to set time for which splash screen will be displayed. After the defined time Intent used here will direct us MainScreen activity.
package com.javapapers.android.introslider; import android.content.Intent; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; public class Splash_screen extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_splash_screen); Thread myThread = new Thread() { @Override public void run() { try { sleep(1200); Intent intent = new Intent(getApplicationContext(),MainScreen.class); startActivity(intent); finish(); } catch (InterruptedException e) { e.printStackTrace(); } } }; myThread.start(); } }
MainScreen activity holds the ViewPager. It has built-in swipe gestures to flip left and right through pages, and display screen slide animations. It is contained in android.support.v4.view.ViewPager library.
Next Button added is used to direct us to next screen. Skip button is used to skip Intro slides and will launch home acivity.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <android.support.v4.view.ViewPager android:id="@+id/view_pager" android:layout_width="match_parent" android:layout_height="match_parent" /> <LinearLayout android:id="@+id/layoutBars" android:layout_width="match_parent" android:layout_height="60dp" android:layout_alignParentBottom="true" android:layout_marginBottom="51dp" android:gravity="center" android:orientation="horizontal"> </LinearLayout> <Button android:id="@+id/next" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="@null" android:text="next" android:textSize="20dp" android:textColor="@android:color/white" android:layout_alignParentBottom="true" android:layout_centerHorizontal="true" /> <View android:layout_width="match_parent" android:layout_height="1dp" android:alpha=".4" android:background="@android:color/white" android:layout_above="@+id/next" android:layout_alignParentStart="true" /> <Button android:id="@+id/skip" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="@null" android:text="skip" android:textSize="20dp" android:textColor="@android:color/white" android:layout_alignParentTop="true" android:layout_alignParentEnd="true" android:layout_marginTop="34dp" /> </RelativeLayout>
Here before execution, it is checked if it is first time launch. If not it will direct us to main activity.
bottomBars() method is used to add bars. It tells which screen is active during run time.
package com.javapapers.android.introslider; import android.content.Context; import android.content.Intent; import android.view.LayoutInflater; import android.view.ViewGroup; import android.view.View; import android.support.v7.app.AppCompatActivity; import android.text.Html; import android.widget.Button; import android.os.Bundle; import android.support.v4.view.PagerAdapter; import android.support.v4.view.ViewPager; import android.widget.LinearLayout; import android.widget.TextView; public class MainScreen extends AppCompatActivity { PreferenceManager preferenceManager; LinearLayout Layout_bars; TextView[] bottomBars; int[] screens; Button Skip, Next; ViewPager vp; MyViewPagerAdapter myvpAdapter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main_screen); vp = (ViewPager) findViewById(R.id.view_pager); Layout_bars = (LinearLayout) findViewById(R.id.layoutBars); Skip = (Button) findViewById(R.id.skip); Next = (Button) findViewById(R.id.next); myvpAdapter = new MyViewPagerAdapter(); vp.setAdapter(myvpAdapter); preferenceManager = new PreferenceManager(this); vp.addOnPageChangeListener(viewPagerPageChangeListener); if (!preferenceManager.FirstLaunch()) { launchMain(); finish(); } screens = new int[]{ R.layout.intro_screen1, R.layout.intro_screen2, R.layout.intro_screen3 }; ColoredBars(0); } public void next(View v) { int i = getItem(+1); if (i < screens.length) { vp.setCurrentItem(i); } else { launchMain(); } } public void skip(View view) { launchMain(); } private void ColoredBars(int thisScreen) { int[] colorsInactive = getResources().getIntArray(R.array.dot_on_page_not_active); int[] colorsActive = getResources().getIntArray(R.array.dot_on_page_active); bottomBars = new TextView[screens.length]; Layout_bars.removeAllViews(); for (int i = 0; i < bottomBars.length; i++) { bottomBars[i] = new TextView(this); bottomBars[i].setTextSize(100); bottomBars[i].setText(Html.fromHtml("¯")); Layout_bars.addView(bottomBars[i]); bottomBars[i].setTextColor(colorsInactive[thisScreen]); } if (bottomBars.length > 0) bottomBars[thisScreen].setTextColor(colorsActive[thisScreen]); } private int getItem(int i) { return vp.getCurrentItem() + i; } private void launchMain() { preferenceManager.setFirstTimeLaunch(false); startActivity(new Intent(MainScreen.this, MainActivity.class)); finish(); } ViewPager.OnPageChangeListener viewPagerPageChangeListener = new ViewPager.OnPageChangeListener() { @Override public void onPageSelected(int position) { ColoredBars(position); if (position == screens.length - 1) { Next.setText("start"); Skip.setVisibility(View.GONE); } else { Next.setText(getString(R.string.next)); Skip.setVisibility(View.VISIBLE); } } @Override public void onPageScrolled(int arg0, float arg1, int arg2) { } @Override public void onPageScrollStateChanged(int arg0) { } }; public class MyViewPagerAdapter extends PagerAdapter { private LayoutInflater inflater; public MyViewPagerAdapter() { } @Override public Object instantiateItem(ViewGroup container, int position) { inflater = (LayoutInflater) getSystemService(Context.LAYOUT_INFLATER_SERVICE); View view = inflater.inflate(screens[position], container, false); container.addView(view); return view; } @Override public int getCount() { return screens.length; } @Override public void destroyItem(ViewGroup container, int position, Object object) { View v = (View) object; container.removeView(v); } @Override public boolean isViewFromObject(View v, Object object) { return v == object; } } }
Now we will add screen layouts. Here, i have added 3 screens.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@color/screen1"> <ImageView android:layout_width="150dp" android:layout_height="150dp" android:src="@drawable/video2" android:layout_centerVertical="true" android:layout_centerHorizontal="true" android:id="@+id/imageView" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="VIDEO" android:textColor="@android:color/white" android:textSize="50dp" android:textStyle="bold" android:layout_below="@+id/imageView" android:layout_centerHorizontal="true" android:layout_marginTop="32dp" > </RelativeLayout>
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@color/screen2"> <ImageView android:layout_width="150dp" android:layout_height="150dp" android:src="@drawable/camera2" android:layout_centerVertical="true" android:layout_centerHorizontal="true" android:id="@+id/imageView" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="CAMERA" android:textColor="@android:color/white" android:textSize="50dp" android:textStyle="bold" android:layout_below="@+id/imageView" android:layout_centerHorizontal="true" android:layout_marginTop="32dp" /> </RelativeLayout>
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@color/screen3"> <ImageView android:layout_width="150dp" android:layout_height="150dp" android:src="@drawable/gallery" android:layout_centerVertical="true" android:layout_centerHorizontal="true" android:id="@+id/imageView" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="GALLERY" android:textColor="@android:color/white" android:textSize="50dp" android:textStyle="bold" android:layout_below="@+id/imageView" android:layout_centerHorizontal="true" android:layout_marginTop="32dp" /> </RelativeLayout>
We need to add PreferenceManager class to manage the screens in viewpager.
package com.javapapers.android.introslider; import android.content.Context; import android.content.SharedPreferences; public class PreferenceManager { SharedPreferences sharedPreferences; SharedPreferences.Editor spEditor; Context context; private static final String FIRST_LAUNCH = "firstLaunch"; int MODE = 0; private static final String PREFERENCE = "Javapapers"; public PreferenceManager(Context context) { this.context = context; sharedPreferences = context.getSharedPreferences(PREFERENCE, MODE); spEditor = sharedPreferences.edit(); } public void setFirstTimeLaunch(boolean isFirstTime) { spEditor.putBoolean(FIRST_LAUNCH, isFirstTime); spEditor.commit(); } public boolean FirstLaunch() { return sharedPreferences.getBoolean(FIRST_LAUNCH, true); } }
I added a Replay button in main activity so that we can view Intro slides again.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#25edea" android:gravity="center" android:orientation="vertical"> <Button android:id="@+id/b1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="#ffffff" android:text="REPLAY" /> </LinearLayout>
package com.javapapers.android.introslider; import android.content.Intent; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.Button; public class MainActivity extends AppCompatActivity { Button b1; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); b1 = (Button) findViewById(R.id.b1); } public void replay(View view) { PreferenceManager preferenceManager = new PreferenceManager(getApplicationContext()); preferenceManager.setFirstTimeLaunch(true); startActivity(new Intent(MainActivity.this, MainScreen.class)); finish(); } }
Download Intro Slider Splash Android App