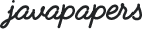
All you need is just 10 minutes to build an Artificial Intelligence (AI) powered Chatbot. AI is the future – like it or not! Will AI save or destroy humanity, let us leave that discussion to Mark and Elon for now. As a developer let us embrace it. Chatbots are taking over customer service jobs all over. It is already happening and at a rate with which we cannot imagine.
I have good experience in web hosting. I have been using web hosting for around 20 years and I have used right from the cheapest provider from my backyard to the premium so called International “cloud” providers. When it comes to customer service they are all the same. If you ask a couple of questions away from the standard set of Q&A they have, then you will know that bots are way better than them.
Let alone the web hosting support, there are too trivial support jobs and they are easily replaced by a chatbot. In this article, I will help you create a an Android AI chatbot powered by IBM Watson. Watson is an AI platform by IBM. It was introduced in 2010 as a question answering system capable of answering questions in natural language. It was named after the IBM’s first CEO.
Watson is one of the popular AI platform in use. It is available in the cloud as a SAAS and anybody can create an account and start using it in minutes. In this tutorial, I will just be introducing you to the IBM console and help you get started with it. Just a “Hello World” kinda thing. It provides great functionality which you can explore and build great AI systems that can pass Turing test.
We will be using only tools and api that are free. As of now, IBM Watson provides the Lite Plan of “Conversation Service” free with 10,000 API Calls per Month. We will be using this to build this Android Chatbot.
First let us start with creating an account in IBM cloud. Sign up with your email and it’s just free. Then login into the bluemix console.
Now you will be forwarded to the Watson page. Click “Create Watson service” button to create a service.
Then click “Conversation”.
Check the Pricing detail. It is free for 10,000 API calls per Month with some added conditions. That should be more than sufficient for our educational purposes. Even for a starter APP that should be cool enough.
The in the Watson Conversation page, create a new Workspace (up to 5 workspaces free as per this Lite plan).
Now let us start with Intent. “An Intent is the goal or purpose of the user’s input…”
Plan for our Chatbot is, it will be a simple hello world kind of bot.
We will have three intents. The first intent is greeting.
The intent will map to the phrases we enter, in our example case, “good morning”, “hello”, “hi”, “welcome”. Here you can add your imagination and make your Chatbot as intelligent as possible. Then similarly create one more intent as “#Name” and supply it with phrases like “what is your name?”. Then the final intent #Joke.
Now we are done with Intents and let us move to Dialog.
“A dialog uses intents, entities, and content from your application to return a response to each user’s input…”
Click the “Dialog” tab in the top. The “Add node”,
Note the “If bot recognizes” in the right side. It should map to your already created intent. Then, you can add the responses below. Similarly add nodes for Name and Joke also. Already there will be a node “Anything else” which is the fall back node which will handle the request when none of the node matches the input.
We can do a trial run in the console itself. There is a bubble icon in the top right corner, click that and you will get the “Try it out” window shown in the above image. You can chat and get response from the Chatbot.
Now let us get ready to use the Watson Chatbot we can created from an Android APP. Click the “Deploy” icon on the left side bar and get the workspace details. We will need,
Let us rock on to building the Android APP now.
We do not have much to do in the Android APP front. It is just an user interface for the Chatbot. So, we will keep it as simple as possible. Our objective is to learn the overall outlay of building an AI powered Chatbot and let’s stick to it.
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
Give permission for accessing Internet as we have to call the IBM Watson API. The complete manifest will be as follows,
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.javapapers.android.aichatbot"> <uses-permission android:name="android.permission.INTERNET" /> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Gradle build dependencies
compile 'com.ibm.watson.developer_cloud:java-sdk:3.7.2' compile 'com.github.kittinunf.fuel:fuel-android:1.9.0'
We will use the IBM Watson SDK to interact with the Conversation API. Fuel just makes our HTTP calls easier.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:padding="16dp" tools:context="com.javapapers.android.aichatbot.MainActivity"> <ScrollView android:layout_width="match_parent" android:layout_height="match_parent" android:layout_above="@+id/user_statement_container"> <TextView android:id="@+id/tv_chat_display" android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="16sp" /> </ScrollView> <android.support.design.widget.TextInputLayout android:id="@+id/user_statement_container" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentBottom="true"> <EditText android:id="@+id/et_user_statement" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Your Message:" android:imeOptions="actionDone" android:inputType="textShortMessage" /> </android.support.design.widget.TextInputLayout> </RelativeLayout>
The layout is simple, just displays the chat conversation and has an input field for the user to chat with the bot.
Here is the main activity that has the logic of accessing the Watson API. Start the class by instantiating the ConversationService API. It is used to interact with the Watson chat API. We build the MessageRequest using the user input and pass that on to the service. In turn, we will get the response back. Whatever response we get will be appended to the chat conversation display in the Chatbot UI.
To add a flavor to our Chatbot, here is a small twist to it. Let us make it tell random jokes to the user and make it a Joke Bot. From the response check if the Conversation Intent is identified as “#Joke”, then let us access a third party API, get a joke and display it to the user. All this will happen behind the screens.
There are numerous free Joke API services available in the Internet and I am using icanhazdadjoke.com to get random jokes. Fuel API is used to do the HTTP request.
package com.javapapers.android.aichatbot; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.text.Html; import android.util.Log; import android.view.KeyEvent; import android.view.inputmethod.EditorInfo; import android.widget.EditText; import android.widget.TextView; import com.github.kittinunf.fuel.Fuel; import com.github.kittinunf.fuel.core.FuelError; import com.github.kittinunf.fuel.core.Handler; import com.github.kittinunf.fuel.core.Request; import com.github.kittinunf.fuel.core.Response; import com.ibm.watson.developer_cloud.conversation.v1.ConversationService; import com.ibm.watson.developer_cloud.conversation.v1.model.MessageRequest; import com.ibm.watson.developer_cloud.conversation.v1.model.MessageResponse; import com.ibm.watson.developer_cloud.http.ServiceCallback; import java.util.HashMap; import java.util.Map; public class MainActivity extends AppCompatActivity { private static final String TAG = "MainActivity"; private ConversationService myConversationService = null; private TextView chatDisplayTV; private EditText userStatementET; private final String IBM_USERNAME = "abcde-1234-1234-abcde-abcdefghijk"; private final String IBM_PASSWORD = "abcdefghijk"; private final String IBM_WORKSPACE_ID = "xyzxyzxyz-1234-1234-5678-abcdefgixyz123"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); chatDisplayTV = findViewById(R.id.tv_chat_display); userStatementET = findViewById(R.id.et_user_statement); //instantiating IBM Watson Conversation Service myConversationService = new ConversationService( "2017-12-06", IBM_USERNAME, IBM_PASSWORD ); userStatementET.setOnEditorActionListener(new TextView.OnEditorActionListener() { @Override public boolean onEditorAction(TextView tv, int action, KeyEvent keyEvent) { if (action == EditorInfo.IME_ACTION_DONE) { //show the user statement final String userStatement = userStatementET.getText().toString(); chatDisplayTV.append( Html.fromHtml("<p><b>YOU:</b> " + userStatement + "</p>") ); userStatementET.setText(""); MessageRequest request = new MessageRequest.Builder() .inputText(userStatement) .build(); // initiate chat conversation myConversationService .message(IBM_WORKSPACE_ID, request) .enqueue(new ServiceCallback<MessageResponse>() { @Override public void onResponse(MessageResponse response) { final String botStatement = response.getText().get(0); runOnUiThread(new Runnable() { @Override public void run() { chatDisplayTV.append( Html.fromHtml("<p><b>BOT:</b> " + botStatement + "</p>") ); } }); // if the intent is joke then we access the third party // service to get a random joke and respond to user if (response.getIntents().get(0).getIntent().endsWith("Joke")) { final Map<String, String> params = new HashMap<String, String>() {{ put("Accept", "text/plain"); }}; Fuel.get("https://icanhazdadjoke.com/").header(params) .responseString(new Handler<String>() { @Override public void success(Request request, Response response, String body) { Log.d(TAG, "" + response + " ; " + body); chatDisplayTV.append( Html.fromHtml("<p><b>BOT:</b> " + body + "</p>") ); } @Override public void failure(Request request, Response response, FuelError fuelError) { } }); } } @Override public void onFailure(Exception e) { Log.d(TAG, e.getMessage()); } }); } return false; } }); } }
Download Android AI Chatbot Project
Comments are closed for "Android AI Chatbot Powered by IBM Watson".
Hey man, long time no see!!
Welcome back.
Back with a bang.
Excellent article. You make it look so simple. Thanks Joe.
Wow it’s working for me. Thanks thanks
where have you been all these days??
Thanks Joe for this indepth tutorial. Can you please guide to make it an audio based bot.
[…] an Android app which uses the Watson Conversation API. I am following the tutorials mentioned here and here. However, it seems that quite a few of the Api’s functions are deprecated. For […]