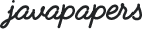
Spring 2.5 introduced support for annotation based MVC controllers. @RequestMapping, @RequestParam, @ModelAttribute are some of the annotations provided for this implementation. We have seen about these controller annotations at high level in a previous tutorial on spring MVC. I will be using the same code available in that tutorial to explain the annotations.
An important note, SimpleFormController is deprecated so do not use it. Spring may remove it from the next major version from the API. Go with annotation based controllers and it is easy to use. Using annotation based controllers means we need not extend some defined base class and need not implement some specific interfaces.
To brush up the basics of annotations I recommend you to go through my tutorial on java annotations.
In spring-context first we need to declare a bean. This is just a stand spring bean definition in dispatcher’s context.
Latest way of doing this is, enabling autodetection. For the @Controller annotation spring gives a feature of autodetection. We can add “component-scan” in spring-context and provide the base-package.
<context:component-scan base-package="com.javapapers.spring.mvc" /> <mvc:annotation-driven />
Then add @Controller annotation to controllers. The dispatcher will start from the base-package and scan for beans that are annotated with @Controller annotation and look for @RequestMapping. @Controller annotation just tells the container that this bean is a designated controller class.
@RequestMapping annotation is used to map a particular HTTP request method (GET/POST) to a specific class/method in controller which will handle the respective request.
@RequestMapping annotation can be applied both at class and method level. In class level we can map the URL of the request and in method we can map the url as well as HTTP request method (GET/POST).
We can use wildcard characters like * for path pattern matching.
In the following example, @RequestMapping(“/hi”) annotated at class level maps the request url and at again at lower level method level mapping is used for HTTP request mapping.
package com.javapapers.spring.mvc; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RequestParam; @Controller @RequestMapping("/hi") public class HelloWorldController { @RequestMapping(method = RequestMethod.GET) public String hello() { return "hello"; } @RequestMapping(method = RequestMethod.POST) public String hi(@RequestParam("name") String name, Model model) { String message = "Hi " + name + "!"; model.addAttribute("message", message); return "hi"; } }
In a multi-action controller urls are mapped at method level since the controller services multiple urls. In below example two urls are serviced by the controller and they are mapped to separate methods.
package com.javapapers.spring.mvc; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RequestParam; @Controller public class HelloWorldController { @RequestMapping("/") public String hello() { return "hello"; } @RequestMapping(value = "/hi", method = RequestMethod.GET) public String hi(@RequestParam("name") String name, Model model) { String message = "Hi " + name + "!"; model.addAttribute("message", message); return "hi"; } }
When using multi-action form controller there is a possibility of creating ambiguity in mapping the urls to methods. In those cases, if we have specified a
org.springframework.web.servlet.mvc.multiaction.MethodNameResolver it will be used to map a method. If a method name resolver is not specified then by default org.springframework.web.servlet.mvc.multiaction.InternalPathMethodNameResolver is used. This default implementation doesnot support wildcard characters.
When a matching method is not found, action will be mapped to a default method in the controller which does not have any @RequestMapping specified. If there are more such methods in the controller, then method name will be taken into consideration.
<beans ...> <bean class="org.springframework.web.servlet.mvc.support.ControllerClassNameHandlerMapping" /> <bean class="com.javapapers.spring.mvc.controller.HelloWorldController"> <property name="methodNameResolver"> <bean class="org.springframework.web.servlet.mvc.multiaction.PropertiesMethodNameResolver"> <property name="mappings"> <props> <prop key="/">hello</prop> <prop key="/hi">hi</prop> </props> </property> </bean> </property> </bean> </beans>
To bind the request parameter a variable in method scope this @RequestParam annotation is used.
In below code “name” is bound to the request parameter.
public String hi(@RequestParam("name") String name, Model model) { String message = "Hi " + name + "!"; model.addAttribute("message", message); return "hi"; }
An annotated method parameter can be mapped to an attribute in a model using @Modelttribute in controller. It can also be used to provide reference data for the model when used at method level.
We can declare session attributes used by a method handler using @SessionAttributes annotation in controller.
Similarly @CookieValue annotation is used to bind a method parameter to a HTTP cookie. In below example, a cookie with key “username” value will be bound to method variable name.
@RequestMapping("/hi") public void userInfo(@CookieValue("username") String name) { //... }
Very similar to cookie binding, @RequestHeader is used to bind a header value to a method parameter.
Assume we have the following header value, and the following annotation in controller will bind the host variable to the value.
Host: localhost:8080
@RequestMapping("/hi") public void hostInfo(@RequestHeader("Host") String host) { //... }
Comments are closed for "Spring Annotation Based Controllers".
Thanx for sharing you knowledge with us. Please keep sharing.I am a regular follower of your blog.
i am new to this blog so i want to know what is the benefit of compiler and interpreter as java use both of them is there any major difference between compiler and interpreter i need detail explanation. i have to know i am waiting for my answer from past 3 years but i am not getting satisfied answer the main question rises in my mind like:-
1. compiler and interpreter both converts a language from one level to another then witch one we have to use and why?
2. is there any thing which can be achieved with interpreter but not with compiler or vice verse.
3. And why java uses both if interpreter and compiler can achieve which is required by java then java designers can use double compiler or interpreter why both .
Please tell me i am confused i don’t know whether my question is right or not.
very nice… Thanks Joe for sharing …
Now look this… one more in a good collection!! Good one Joe, Keep up posting!!
I am a regular follower of your blog.Thanks for sharing please more share about @ModelAttribute Annotation at method level as well as perameter level
Good site to start with Basics.
nice. It helped me a lot to implement the complete annotation based controller…
Thanks, Keep the good works up….
you raise our knowledge all the time by these great posts :)
Above stuff is very helpfull in my project.
Thanks, Keep the good works up….
Can u please tell about the spring restfull web services………?
good article on spring MVC annotation.
very good Site…i like the content n info with itS look,,,too creative..*_*
very good Site…i like the content n info with itS look,,,too creative..*_*
very nice
very helpful – thank you!
written well. Very much useful . keep posting more on spring.
can you please tell me how to set the cookie using spring framework..
Am The Regular follower of your blog… please let me know how to make a single Spring3 controller to handle more than one request that are coming from different forms of same jsp… besides how to validate all the forms in that situation using annotations only?
Please ellobrate the example of @ModelAttribute
and why we use in which senerio
Nice article, It is very useful for me.
Thank you Mr. Joe
How does the Spring configurations work in general?
Let us say that an application is run. What happens from here?
Please explain step by step.
The container looks for the context-path and what happens from there on?
Thanks in advance.
why used java annotation in spring & hibernate? what is its requirement & what is its advantages? can annotation make programmer easy for spring & hibernate?
nice tutorial
Hi Arvind,
1)In java, compiler will convert .java code into byte code(class file) these byte code can be run in any operating system(i.e write once run any where)
2)compiler: to produce byte code(class file)
interpreter: to execute the byte code
compiler cant do the interpreter task and vice-verse.
3)Now we have byte code, in order to run byte code(class file) we require some thing called java interpreter(JVM)which is a specific implementation with respect to the Operating system, this byte code(class file)is picked by the interpreter to execute(run)
interpreter will have it own set of instruction set, and we come to conclusion stating that interpreter is an instance of JRE
please let me know if am wrong any where
Awaiting for your kind response
Thanks,
Daya
Thanks
hi easy to understand.
Hi, nice tutorial. Very helpful.
Thanks
Great article for beginners..
Good GUI but not as much portable.
Thank you!
Your blog is very useful,and also easy to understand. Thank you..
exlent
good
Very good tutorial, thanks for sharing….
I’ve a doubt in Multiaction Controller. I need to execute a function in the onload of all controller classes. So I created a base class which extends MultiactionController. But after that how will I define this common function so that all controllers that are extended this base class execute this function.
thank you very much. These stuff is very useful
excellent explanation…
Really this is fantastic .If possible just do it for servlets , jsp and springs completely for all concepts with easy diagram and example with explanation.
Thank you
[…] If you are a beginner, go through the Spring MVC tutorial before taking this. We will be using Spring 3 annotation based approach for the web […]
It was excellent.
Thank You so much
Thank you.
[…] For all these annotations (stereotypes), technically the core purpose is same. Spring automatically scans and identifies all these classes that are annotated with “ @Component, @Service, @Repository, @Controller” and registers BeanDefinition with ApplicationContext. We read about @Controller in a previous Spring tutorial on annotation based controllers. […]
Thankyou very much for your explaination in annotation based configuration.
once again Thankyou!…………
Hi daya,
sorry for the late response i am glad to know that you have shown some interest in my question but please read these 3 points carefully again if you have ample time and then share your answer.you are correct for which you are trying to explain, i knew that but my this is not my question’s answer.
Actually this is the point where my question starts.
Thanks for the reply daya :)
package Annotations;
import java.util.List;
import java.util.Map;
//import net.roseindia.model.LoginForm;
//import net.roseindia.service.MyService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class AnnotationController {
private MyService myService;
@Autowired
public void setMyService(MyService myService) {
this.myService = myService;
}
@RequestMapping(“/loginForm.html”)
public String loadForm(Map model) {
return “loginForm”;
}
@ModelAttribute(“loginForm”)
public LoginForm returnModel() {
return new LoginForm();
}
@ModelAttribute(“countryList”)
public List loadCounties() {
return myService.loadCountry();
}
@RequestMapping(“/display.html”)
public String responseForm(Map model, @Value() LoginForm loginForm) {
if (loginForm.getLoginID() != “” && loginForm.getPassword() != “”){
return “displayDetail”;
}
return “loginForm”;
}
}
simple and easy to understand…
Thanks
in spring container has its own init (),service() ,destroy() method.if then how it works.
please clear my doubt.
thanks
Compiler produces machine dependent code , which cannot be ported to all other machines to run. Where as in Java the interpreted code is compiled with the help of JVM and produce the machine dependent. Since JVM is specific to each platform, and due to the fact that it generates the machine dependent code Just when it is ready to run there is no problem of machine dependency. In order to achieve this write once run anywhere principle Java has both interpreter and compiler
Thaks alot this tutorial now i got clear understanding about request handler and controller
CAn you please please give a full source code example with annotation
so that i could get full idea
i am close to nothing
:(
PLease help i have found only your site helpful
Good tutorial
Hi Joe,
Can you please provide details how to implement
form wizard in spring 3 MVC.
Thanks,
Sha.
Can u please tell me how to validate request parameters pattern
@RequestMapping(value = “/get_list”,
method = RequestMethod.GET,
headers=”Accept=*/*”)
Hi,
I am getting error at headers. I have added all recent spring jars.