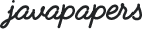
This Java tutorial is to help understand the Temporal Adjuster introduced in Java 8 Date and Time API. In a previous tutorial we saw an introduction to Java 8 Date and Time API and followed by a tutorial for Java Temporal Adjuster.
In this tutorial, let us see how to write a temporal adjuster for finding the thanks giving day in a given year.
package com.javapapers.java8.dateandtime; import java.time.DayOfWeek; import java.time.LocalDate; import java.time.Month; import java.time.temporal.Temporal; import java.time.temporal.TemporalAdjuster; import java.time.temporal.TemporalAdjusters; // Every year 4th Thursday of November is Thanks Giving Day in US public class ThanksGivingDayTemporalAdjuster implements TemporalAdjuster { @Override public Temporal adjustInto(Temporal temporalAdjusterInput) { LocalDate temporalAdjusterDate = LocalDate.from(temporalAdjusterInput); LocalDate firstNovInYear = LocalDate.of(temporalAdjusterDate.getYear(), Month.NOVEMBER, 1); // adjusting four weeks for Thursday LocalDate thanksGivingDay = firstNovInYear .with(TemporalAdjusters.nextOrSame(DayOfWeek.THURSDAY)) .with(TemporalAdjusters.next(DayOfWeek.THURSDAY)) .with(TemporalAdjusters.next(DayOfWeek.THURSDAY)) .with(TemporalAdjusters.next(DayOfWeek.THURSDAY)); return thanksGivingDay; } public static void main(String... strings) { LocalDate currentDate = LocalDate.now(); ThanksGivingDayTemporalAdjuster thanksGivingDayAdjuster = new ThanksGivingDayTemporalAdjuster(); LocalDate thanksGivingDay = currentDate.with(thanksGivingDayAdjuster); System.out.println("In Year " + currentDate.getYear() + ", Thanks Giving Day(US) is on " + thanksGivingDay); } }
In US, the Thanks Giving day is celebrated every year on 4th Thursday of November. The above TemporalAdjuster first fixes the month as November and then shifts the date four times for the day Thursday.
In Year 2014, Thanks Giving Day(US) is on 2014-11-27
Comments are closed for "Temporal Adjuster Example: Thanks Giving Day".
That’s such a helpful tutorial of JAVA which explain temporal adjuster in JAVA 8 with example.
Java 8 seems very promising Thanks for your effort in making these tutorials