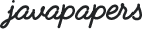
For now this is the last example in the temporal adjuster example series. This tutorial is to find second Saturday of a month. Refer the temporal adjuster tutorial and introduction to java 8 date and time API to know more about this topic.
Temporal adjuster is a powerful utility. It will be really handy in projects with date time operations. Before Java 8, it was not at all easy to work with date and time. This date and time API provides us great relief from Java 8 release.
package com.javapapers.java8.dateandtime; import java.time.DayOfWeek; import java.time.LocalDate; import java.time.temporal.Temporal; import java.time.temporal.TemporalAdjuster; import java.time.temporal.TemporalAdjusters; public class SecondSaturdayTemporalAdjuster implements TemporalAdjuster { @Override public Temporal adjustInto(Temporal temporalAdjusterInput) { LocalDate temporalAdjusterDate = LocalDate.from(temporalAdjusterInput); LocalDate firstNovInYear = LocalDate.of(temporalAdjusterDate.getYear(), temporalAdjusterDate.getMonth(), 1); // adjusting two weeks LocalDate secondSaturday = firstNovInYear.with( TemporalAdjusters.nextOrSame(DayOfWeek.SATURDAY)).with( TemporalAdjusters.next(DayOfWeek.SATURDAY)); return secondSaturday; } public static void main(String... strings) { LocalDate currentDate = LocalDate.now(); SecondSaturdayTemporalAdjuster secondSaturdayDayAdjuster = new SecondSaturdayTemporalAdjuster(); LocalDate secondSaturday = currentDate.with(secondSaturdayDayAdjuster); System.out.println("Second Saturday is on " + secondSaturday); } }
In many developing countries, Sunday is the only Off day in a week. Added to that one day weekly off, every second Saturday in a month is holiday. This is a special use case and this example program is to explain how we create a temporal adjuster to find the second Saturday in a given month. This is similar to the earlier seen example of finding Thanks Giving day in a year.
Second Saturday is on 2014-08-09