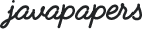
In this tutorial, we will see about temporal adjusters and truncation in Java SE8 Date and time API. This is a continuation of the Java 8 features introduction series. In the previous tutorial we saw about date construction, getter methods and calculate date and time with Java 8 API.
TemporalAdjuster is a functional interface and a key tool for modifying a temporal object. This is an implementation of the strategy design pattern using which the process of adjusting a value is externalized. This interface has a method adjustInto(Temporal) and it can be directly called by passing the Temporal object. It takes input as the temporal value and returns the adjusted value. It can also be invoked using ‘with’ method of the temporal object to be adjusted.
Java 8 API comes with a class named TemporalAdjusters and it provides multiple TemporalAdjuster implementations that can be used to adjust the Date and Time objects. Like based on the passed date, we can find the first day of that month and etc.
package com.javapapers.java8.dateandtime; import java.time.DayOfWeek; import java.time.LocalDateTime; import java.time.temporal.TemporalAdjusters; public class DateAndTimeTemporalAdjusters { public static void main(String... strings) { LocalDateTime currentDateTime = LocalDateTime.now(); System.out.println("firstDayOfMonth: " + currentDateTime.with(TemporalAdjusters.firstDayOfMonth())); System.out.println("lastDayOfMonth: " + currentDateTime.with(TemporalAdjusters.lastDayOfMonth())); System.out .println("firstDayOfNextMonth: " + currentDateTime.with(TemporalAdjusters .firstDayOfNextMonth())); System.out.println("firstDayOfNextYear: " + currentDateTime.with(TemporalAdjusters.firstDayOfNextYear())); System.out.println("firstDayOfYear: " + currentDateTime.with(TemporalAdjusters.firstDayOfYear())); System.out .println("next DayOfWeek: " + currentDateTime.with(TemporalAdjusters .next(DayOfWeek.MONDAY))); } }
Output of the Temporal Adjuster Example
firstDayOfMonth: 2014-08-01T20:44:09.359 lastDayOfMonth: 2014-08-31T20:44:09.359 firstDayOfNextMonth: 2014-09-01T20:44:09.359 firstDayOfNextYear: 2015-01-01T20:44:09.359 firstDayOfYear: 2014-01-01T20:44:09.359 next DayOfWeek: 2014-08-11T20:44:09.359
Similar to the TemporalAdjusters class provided in the Java 8 API, we can create our own custom implementation of TemporalAdjuster having our business logic and use it in the Java application. It should be useful when there are repeating patterns of adjustment in our use cases. Those can be externalized using a TemporalAdjuster implementation.
Following is an example of a TemporalAdjuster implementation where given a date we adjust and return the next odd date.
package com.javapapers.java8.dateandtime; import java.time.LocalDate; import java.time.Month; import java.time.temporal.Temporal; import java.time.temporal.TemporalAdjuster; public class CustomTemporalAdjuster implements TemporalAdjuster { public Temporal adjustInto(Temporal temporalInput) { LocalDate localDate = LocalDate.from(temporalInput); int day = localDate.getDayOfMonth(); if (day % 2 == 0) { localDate = localDate.plusDays(1); } else { localDate = localDate.plusDays(2); } return temporalInput.with(localDate); } public static void main(String... strings) { LocalDate someDate1 = LocalDate.of(2014, Month.AUGUST, 1); LocalDate someDate2 = LocalDate.of(2014, Month.AUGUST, 2); CustomTemporalAdjuster nextOddDay = new CustomTemporalAdjuster(); LocalDate nextOddDay1 = someDate1.with(nextOddDay); LocalDate nextOddDay2 = someDate2.with(nextOddDay); System.out.println("Next Odd Day for " + someDate1 + " is " + nextOddDay1); System.out.println("Next Odd Day for " + someDate2 + " is " + nextOddDay2); } }
Output:
Next Odd Day for 2014-08-01 is 2014-08-03 Next Odd Day for 2014-08-02 is 2014-08-03
Comments are closed for "Java 8 Date and Time: Temporal Adjuster".
Very helpful. Thanks!