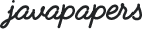
This Java tutorial is to introduce the Java SE 8 Date and Time API. For long Java developers were not happy about the date and time support in the API. Main reason were the API was not consistent in its design, required numerous casting and conversions to achieve basic use case, not thread safe, etc. These were carefully looked at and mitigated in this new Java 8 API design.
In the list of Java 8 features, Date and Time API is going to be the most used by Java developers. JSR 301 is the Java Specification Request of Date and Time API release with Java SE 8. Stephen Colebourne (author of Joda Time) is one of the specification leads.
Constructing date and time objects have become easy and usable.
package com.javapapers.java8.dateandtime; import java.time.LocalDate; import java.time.LocalDateTime; import java.time.LocalTime; import java.time.Month; public class DateAndTime { public static void main(String args[]) { // current date LocalDateTime currentDateTime = LocalDateTime.now(); System.out.println(currentDateTime); // create date from passed parameters LocalDate date1 = LocalDate.of(2012, Month.DECEMBER, 12); System.out.println(date1); // create time from passed parameters LocalTime time1 = LocalTime.of(18, 30); System.out.println(time1); // parse time from a string LocalTime time2 = LocalTime.parse("10:15:30"); System.out.println(time2); } }
Output is:
2014-08-04T17:28:30.003 2012-12-12 18:30 10:15:30
Before Java 8, to get the current month in an integer variable, we need to cross seven mountains and seas. Not its just in the calling distance.
package com.javapapers.java8.dateandtime; import java.time.LocalDateTime; import java.time.Month; public class GetDateAndTime { public static void main(String... args) { LocalDateTime currentDateTime = LocalDateTime.now(); System.out.println("Current local date and time: " + currentDateTime); Month month = currentDateTime.toLocalDate().getMonth(); System.out.println("Month: "+month); int day = currentDateTime.toLocalDate().getDayOfMonth(); System.out.println("Day: "+day); int secs = currentDateTime.getSecond(); System.out.println("Current second: "+secs); } }
Output:
Current local date and time: 2014-08-04T17:36:10.814 Month: AUGUST Day: 4 Current second: 10
This is a real killer. Think about how we will add some X number of days to the current month before Java 8. All classes in Java 8 Date and Time API are immutable. For any operation a new instance will be returned.
package com.javapapers.java8.dateandtime; import java.time.LocalDateTime; public class CalculateDateAndTime { public static void main(String... args) { LocalDateTime currentDateTime = LocalDateTime.now(); LocalDateTime lastYear = currentDateTime.withYear(2013); System.out.println("Same day last year: " + lastYear); LocalDateTime lastYearPlusOneWeek = lastYear.plusWeeks(1); System.out.println("Same day last year with one week added: " + lastYearPlusOneWeek); LocalDateTime tenYrFromNow = currentDateTime.plusYears(10); System.out.println("Ten years from now: " + tenYrFromNow); } }
Output:
Same day last year: 2013-08-04T17:46:41.371 Same day last year with one week added: 2013-08-11T17:46:41.371 Ten years from now: 2024-08-04T17:46:41.371
Comments are closed for "Java 8 Date and Time: Construction, Get and Set".
Really a good tutorial
Very nice tutorial on the intro of java8 date time api.
Nice tutorial ! Thanks.
Very nice and helpful information.
It was really simple and helpful. Thanks!
New Date Time Features are really awesome!!!