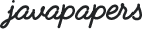
Template method design pattern is to define an algorithm as skeleton of operations and leave the details to be implemented by the child classes. The overall structure and sequence of the algorithm is preserved by the parent class.
This behavioral design pattern is one of the easiest to understand and implement. This design pattern is used popularly in framework development. This helps to avoid code duplication also.
Template method design pattern has two components. An abstract parent class and one or more concrete child classes extending that parent class. The abstract parent class is the template class used to define the algorithmic steps and preserve it across implementations. Child class contains details of the abstract methods.
In the above UML diagram an example is shown. It deals with order processing flow. The OrderProcessTemplate class is an abstract class containing the algorithm skeleton. As shown on note, processOrder() is the method that contains the process steps. We have two subclasses NetOrder and StoreOrder which has the same order processing steps.
So the overall algorithm used to process an order is defined in the base class and used by the subclasses. But the way individual operations are performed vary depending on the subclass.
package com.javapapers.designpatterns.template; public abstract class OrderProcessTemplate { public boolean isGift; public abstract void doSelect(); public abstract void doPayment(); public final void giftWrap() { System.out.println("Gift wrap done."); } public abstract void doDelivery(); public final void processOrder() { doSelect(); doPayment(); if (isGift) { giftWrap(); } doDelivery(); } }
package com.javapapers.designpatterns.template; public class NetOrder extends OrderProcessTemplate { @Override public void doSelect() { System.out.println("Item added to online shopping cart,"); System.out.println("Get gift wrap preference,"); System.out.println("Get delivery address."); } @Override public void doPayment() { System.out.println("Online Payment through Netbanking/Cards."); } @Override public void doDelivery() { System.out.println("Ship the item through post to delivery address"); } }
package com.javapapers.designpatterns.template; public class StoreOrder extends OrderProcessTemplate { @Override public void doSelect() { System.out.println("Customer chooses the item from shelf."); } @Override public void doPayment() { System.out.println("Pays at counter through cash/POS"); } @Override public void doDelivery() { System.out.println("Item deliverd to in delivery counter."); } }
package com.javapapers.designpatterns.template; public class TemplateMethodPatternClient { public static void main(String... args) { System.out.println("$$$$$$$ NetOrder instance of the Template: $$$$$$$"); OrderProcessTemplate netOrder = new NetOrder(); netOrder.processOrder(); System.out.println("$$$$$$$ StoreOrder instance of the Template: $$$$$$$"); OrderProcessTemplate storeOrder = new StoreOrder(); storeOrder.processOrder(); } }
$$$$$$$ NetOrder instance of the Template: $$$$$$$ Item added to online shopping cart, Get gift wrap preference, Get delivery address. Online Payment through Netbanking/Cards. Ship the item through post to delivery address $$$$$$$ StoreOrder instance of the Template: $$$$$$$ Customer chooses the item from shelf. Pays at counter through cash/POS Item deliverd to in delivery counter.
Class HttpServlet and it doGet, doPost, doPut,… methods are example of template method pattern usage in Java API.
Comments are closed for "Template Method Design Pattern".
Is there any end to design patterns? ..IF they keep on inventing or discovering new patterns , how many should we remember?.
Sorry for being offtopic , but Are these design patterns worth studying in the current world?
Thats a nice question !! .. The author has explained the Template design pattern in very easy terms.
You may not be able to remember or memorize all the design patterns out there cos its like remembering ideas of human beings in general which is crazy to do !!
But the reason of why these are termed accordingly is just to related a specific approach with a common name… whatever that works for your project / implementation is completely left to your imagination !!
Awesome Details in simple language . I like your post always. I am your one of Fan.
Thanks Hari for the question and Raghav for the nice response.
Just trying to memorize the design patterns will never work. You need to relate these to the project you work and try to apply them or find out if they are already applied. If you learn these in such a way, then it can stand longer.
There is no question about do we need design patterns! We absolutely need them. Design patterns are defined solution to commonly occurring problems. We need not find a solution to a repeating problem. Its better to reuse already existing solution patterns as it is already used, tested and verified.
All we need to do is identify problem and match it with available design patterns. Do not worry about it, you will get that knack over experience. Work closely with a software architect and he may be able to highlight and show you how a design pattern is used in your project.
Thanks Siddhartha.
Awesome notes and explanation with such an ease.
Include me in your fan list.
A great resource!
oh.. great tutorial.
I want to learn design pattern but I don’t know where I should start to learn it. Can you tell me where I should start?
And Web app-programing mostly use it or not?
Lovely Article and explained in very simple language. Can you please also include Visitor and Strategy Pattern, I could not find these two.
Thanks in Advance. :)
Hi Joe,
Thanks for the nice explanation!
Can you let us know the difference between Streategy and Template design pattern?
-Vaibhav
Nice Article with very clear explanation.
Thanks.
In UML graph, Should a realization relationship be presented by a dash arrow?
Good explanation !!
The pattern I see in all these patterns is tht it always revolves around making combinations of inheritance polymorphism or interface polymorphism !
Will you please explain me what is HOOK METHOD and how it is useful in Template Method Design Pattern?
Hi Joe,
Very good explanation in simple terms. Thanks.
Joe,the explanation is really clear and extract the idea behind this design pattern. Thanks