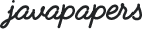
In this Twitter+Java tutorial we will see about how to Tweet / post using Java via Twitter API. Twitter provides a rich REST API to create Twitter enabled applications. It provides the complete possibilities, right from reading a timeline to posting the status.
There are two options,
Before going to the code, we should generate Twitter API keys which enables us to access the APIs. These
We need to get “Consumer Key” and “Consumer Secret” from Twitter. Using these two, we should generate, “Access Token” and “Access Token Secret”.
Go to the URL https://dev.twitter.com/apps and create a new application. Give access level in ‘Settings’ as ‘Read, Write and Access direct messages’. You will get both consumer key and secret.
We can generate access tokens using the consumer keys. It can be done using the API or through the interface as well. I am going with generating using the Twitter website itself as shown below. Ensure that the access level comes the same as in consumer keys or regenerate it.
Now we are all set to post to Twitter using Java. In this section, let us see how we can use the REST APIs directly in our Java application. Let us use the status update post Twitter API
All we need to do is,
To make these REST call lets use oauth-signpost and Apache Commons HTTP. Following are the dependent jars to be used,
package com.javapapers.java; import oauth.signpost.OAuthConsumer; import oauth.signpost.commonshttp.CommonsHttpOAuthConsumer; import org.apache.commons.io.IOUtils; import org.apache.http.HttpResponse; import org.apache.http.client.HttpClient; import org.apache.http.client.methods.HttpPost; import org.apache.http.impl.client.DefaultHttpClient; public class JavaRestTweet { static String consumerKeyStr = "XXXXXXXXXXXXXXXXXXXXXXXXXXXX"; static String consumerSecretStr = "XXXXXXXXXXXXXXXXXXXXXXXXXXXX"; static String accessTokenStr = "XXXXXXXXXXXXXXXXXXXXXXXXXXXX"; static String accessTokenSecretStr = "XXXXXXXXXXXXXXXXXXXXXXXXXXXX"; public static void main(String[] args) throws Exception { OAuthConsumer oAuthConsumer = new CommonsHttpOAuthConsumer(consumerKeyStr, consumerSecretStr); oAuthConsumer.setTokenWithSecret(accessTokenStr, accessTokenSecretStr); HttpPost httpPost = new HttpPost( "http://api.twitter.com/1.1/statuses/update.json?status=Hello%20Twitter%20World."); oAuthConsumer.sign(httpPost); HttpClient httpClient = new DefaultHttpClient(); HttpResponse httpResponse = httpClient.execute(httpPost); int statusCode = httpResponse.getStatusLine().getStatusCode(); System.out.println(statusCode + ':' + httpResponse.getStatusLine().getReasonPhrase()); System.out.println(IOUtils.toString(httpResponse.getEntity().getContent())); } }
Twitter4J is one of the most popular APIs for handling Twitter in Java. If we don’t want to handle the Twitter REST API calls directly as we have shown above and we want go simple then Twitter4J is the way. All we need to do is add only one jar file from Twitter4J twitter4j-core-3.0.5.jar to the dependency and use the respective classes to make the calls.
package com.javapapers.java; import twitter4j.Twitter; import twitter4j.TwitterException; import twitter4j.TwitterFactory; import twitter4j.auth.AccessToken; public class JavaTweet { static String consumerKeyStr = "XXXXXXXXXXXXXXXXXXXXXXXXXXXX"; static String consumerSecretStr = "XXXXXXXXXXXXXXXXXXXXXXXXXXXX"; static String accessTokenStr = "XXXXXXXXXXXXXXXXXXXXXXXXXXXX"; static String accessTokenSecretStr = "XXXXXXXXXXXXXXXXXXXXXXXXXXXX"; public static void main(String[] args) { try { Twitter twitter = new TwitterFactory().getInstance(); twitter.setOAuthConsumer(consumerKeyStr, consumerSecretStr); AccessToken accessToken = new AccessToken(accessTokenStr, accessTokenSecretStr); twitter.setOAuthAccessToken(accessToken); twitter.updateStatus("Post using Twitter4J Again"); System.out.println("Successfully updated the status in Twitter."); } catch (TwitterException te) { te.printStackTrace(); } } }
Comments are closed for "Post to Twitter using Java".
Hi joe,
Can we post to twitter only for user for which twitter app is created?
I mean for each user we have to crete app and access tokens?
You should provide a OAuth sign in process as part of you application. Then the other user(s) has to authenticate using the provided sign-in process. After this you (your app) can post on behalf of the signed-in user.
what should i enter in
Callback URL:
For this example, you can leave it empty. Callback can be used, when we want a page to be called after successful authentication.
Excellent! Joe
Please write something about posting to facebook using java.
HI Paritosh You Can Use RestFB For Facebook ..
Thanks
Hi Joe, can you give an example for oAuth sing in? Thank you!
Hi, I like this site very much.I regular go through this site. I tried Twitter API Example. Its awesome.
Please write some post regarding Facebook API. How to connect through Facebook.
I will definitely try this
please write some tutorials for starters who want to learn RESTful web services, build applications using REST
when I try the first method I get the error 403 forbidden. How I can solve it?
Try changing http to https. It should work
i am getting below error, while creating the app, what’s the resolution? –
Error
Validation failed: Not a valid URL format
Thanks for the tutorial. I am using a different api call (user_timeline.json) but it still works great. I had to change http to https too.
https://api.twitter.com/1.1/search/tweets.json?q=infy&count=999&max_id=505211895112937472&until='2012-09-01‘
here date parameter is not working can u help me sir
Very nice tutorial, you helped me a lot
Thanks so much. :)
Hi sir. Your tutorials are very easy to understand. very kind of you. Can you help me to send Direct message in Twitter ? I tried possible ways yesterday. Still didn’t find solution. I’m using twitter4j lib.
MY CODE IS message = twitter.sendDirectMessage(friendUserId, My Messsage);
I have set Configuration. But still issues are here.
CAN YOU GIVE YOUR SUGGESTION PLEASE ?
Hi Sir,
I am trying to execute JavaRestTweet.java,getting below exception please give me the direction to execute.
Exception in thread “main” java.net.ConnectException: Connection refused: connect
at java.net.DualStackPlainSocketImpl.connect0(Native Method)
at java.net.DualStackPlainSocketImpl.socketConnect(DualStackPlainSocketImpl.java:79)
at java.net.AbstractPlainSocketImpl.doConnect(AbstractPlainSocketImpl.java:339)
at java.net.AbstractPlainSocketImpl.connectToAddress(AbstractPlainSocketImpl.java:200)
at java.net.AbstractPlainSocketImpl.connect(AbstractPlainSocketImpl.java:182)
at java.net.PlainSocketImpl.connect(PlainSocketImpl.java:172)
at java.net.SocksSocketImpl.connect(SocksSocketImpl.java:392)
at java.net.Socket.connect(Socket.java:579)
at org.apache.http.conn.scheme.PlainSocketFactory.connectSocket(PlainSocketFactory.java:117)
at org.apache.http.impl.conn.DefaultClientConnectionOperator.openConnection(DefaultClientConnectionOperator.java:178)
at org.apache.http.impl.conn.ManagedClientConnectionImpl.open(ManagedClientConnectionImpl.java:304)
at org.apache.http.impl.client.DefaultRequestDirector.tryConnect(DefaultRequestDirector.java:610)
at org.apache.http.impl.client.DefaultRequestDirector.execute(DefaultRequestDirector.java:445)
at org.apache.http.impl.client.AbstractHttpClient.doExecute(AbstractHttpClient.java:863)
at org.apache.http.impl.client.CloseableHttpClient.execute(CloseableHttpClient.java:82)
at org.apache.http.impl.client.CloseableHttpClient.execute(CloseableHttpClient.java:106)
at org.apache.http.impl.client.CloseableHttpClient.execute(CloseableHttpClient.java:57)
at JavaRestTweet.main(JavaRestTweet.java:28)
Hi Joseph,
I dont have handson on java. Just by running the code whether the post will be displayed on twitter.
Thanks,
Harsha.