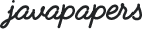
Have you ever seen what is inside a serialized object? I will explain to you what is java serialization, then provide you with a sample for serialization. Finally, most importantly, let us explore what is inside a serialized object and what it means. That is internals of Java serialization and how does it works. If you want to have your own implementation of Java serialization, this article will provide you with a good platform to launch.
Primary purpose of java serialization is to write an object into a stream, so that it can be transported through a network and that object can be rebuilt again. When there are two different parties involved, you need a protocol to rebuild the exact same object again. Java serialization API just provides you that. Other ways you can leverage the feature of serialization is, you can use it to perform a deep copy.
Why I used ‘primary purpose’ in the above definition is, sometimes people use java serialization as a replacement for database. Just a placeholder where you can persist an object across sessions. This is not the primary purpose of java serialization. Sometimes, when I interview candidates for Java I hear them saying java serialization is used for storing (to preserve the state) an object and retrieving it. They use it synonymously with database. This is a wrong perception for serialization.
When you want to serialize an object, that respective class should implement the marker interface serializable. It just informs the compiler that this java class can be serialized. You can tag properties that should not be serialized as transient. You open a stream and write the object into it. Java API takes care of the serialization protocol and persists the java object in a file in conformance with the protocol. De-serialization is the process of getting the object back from the file to its original form.
Here protocol means, understanding between serializing person and de-serializing person. What will be the contents of file containing the serialized object? This serves as a guideline to de-serialize. Have a look at the following sample and how its serialized file looks.
package com.javapapers.sample; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; import java.io.Serializable; class SerializationBox implements Serializable { private byte serializableProp = 10; public byte getSerializableProp() { return serializableProp; } } public class SerializationSample { public static void main(String args[]) throws IOException, FileNotFoundException, ClassNotFoundException { SerializationBox serialB = new SerializationBox(); serialize("serial.out", serialB); SerializationBox sb = (SerializationBox) deSerialize("serial.out"); System.out.println(sb.getSerializableProp()); } public static void serialize(String outFile, Object serializableObject) throws IOException { FileOutputStream fos = new FileOutputStream(outFile); ObjectOutputStream oos = new ObjectOutputStream(fos); oos.writeObject(serializableObject); } public static Object deSerialize(String serilizedObject) throws FileNotFoundException, IOException, ClassNotFoundException { FileInputStream fis = new FileInputStream(serilizedObject); ObjectInputStream ois = new ObjectInputStream(fis); return ois.readObject(); } }
Look at following image. After serializing ‘SerializationBox’ in the above sample code, I opened the output in a hex editor. You can use Notepad++ and hex plugin to open the serialized file.
Let us look at contents byte by byte and find out what they are. It starts with “ac ed”. It is is called STREAM_MAGIC. It is a magic number (java API guys says) that is written to the stream header. It denotes that is start of serialzed content.
Similarly every character has a meaning. Actually the serialized file is more bulkier than you would expect, as it has a huge header the meta information of the classes involved and finally the content. Object Serialization Stream Protocol have a look at chapter 6.4.2 Terminal Symbols and Constants. It gives you list of symbols and constants used in serialization.
In the image, I have underline a unit of information in a separate color for you to easily identify.
ac ed – STREAM_MAGIC – denotes start of serialzed content
00 05 – STREAM_VERSION – serialization version
73 – TC_OBJECT – new Object
72 – TC_CLASSDESC – new Class Descriptor
00 26 – length of the class name
63 6f 6d 2e 6a 61 76 61 70 61 70 65 72 73 2e 73 61 6d 70 6c 65 2e 53 65 72 69 61 6c 69 7a 61 74 69 6f 6e 42 6f 78 – class name
57 fc 83 ca 02 85 f0 18 – SerialVersionUID
02 – this object is serializable
00 01 – count of properties in the serialzed class – one property in our example
42 00 10 – private byte
73 65 72 69 61 6c 69 7a 61 62 6c 65 50 72 6f 70 78 70 – property name – serializableProp in our example
0a – 10 the value – This is the persisted value of the property in our sample
Comments are closed for "Java Serialization".
Thanks for the insight on serialization
Serialization Concept explained nicely. Thanks for Details.
Simple and easy-to-understand explanation. I liked the use of a broad use tool like notepad++ and the Constant mapping. By the way, in wich class this constans are defined?
Thanks so much for the good explanation on serialization
Thanks for such a precise and clear understanding.
Very neatly explained…!
[…] Also know some fundamentals of serialization. […]
Today only I visited this site for the first time and wow..I am in love with its content….very informative indeed. Well its now in my fav list and I have planned to read one topic per day.
Thanks a lot for creating such a useful blog!!!
Hi there,
Nice Explanation in simple and precise format.
keep writing :) .
Cheers
Nitin.V
You made serialization concept just so simple and understandable.
Thanks and keep such articles coming up!
Nice and easily understandable explanation for java serialization. I have never came across this before.
Good Insight
Very Good Explanation. Its very understandable.Thanks
Nice understanding explanation..
Thanks a lot
Hi
Thanks the detail explanation.
Regards,
Suwarna
Hi,
It is very good material on serialization. But i have one doubt.
In EJB we have ejbActivate() and ejbPassivate() methods. In CMP beans the container will serialize the object in ejbActivate() and deserialize in ejbPassivate() methods. Here we are not tranporting the objects via streams to other PCs using serialization protocol. Please explain me in the context of EJB serialization and deserialization?
Thanks
Anand Garlapati.
Thanks for giving me this type of information.
This is a very nice and easy to understande.
Hi,
Just to add while writing Serializable class , its good practice to put a Seriazlizable alert in file so that when some one else add a new field in the class he must ensure that field is either transient or Serializable , so that Serializability of class should not break.
Thanks
Javin
Nice to see detail description,it helps alot to understand internal details.
Please keep posting such a nice blogs.
-Vidhi
Explained in a very Simple Manner.
Thank You.
Its very nice artice , but please cover the Serialization UID concept indetail.
Can i serialise any type of data,means i want to serialise print preview.is it possible???????
Its very nice artice , but please cover the Serialization whole concept in detail
Can you explain the Serialization using Externalizable interface?
thanks in advance.
Serialization Concept has been explained in a simple but easily understandable manner….
Its really cool…
Thank u for ur answer….!:-)
Very Knowledge full about Serialization
very nice… at last found a good website 4 java learning
can we implement Serialization on images…
basically i want image to be converted in binary form..
pls reply
Thanks for providing such tough topic in an easiest way…..
very helpful, thanks.
Very nice. Above contents are helpful to all.
Thanks for such a great work.
Could you please add more examples like serializing images or Resultset,It will be more helpful
i could not understand
“serialization”
something good in this………
Excellent creativity.
Hi this is good
thanks to this site develpers
Can you give some explanation about SerialVersionUID in serialization
I appreciated it.It is very nice explanation.
sir I want to some easy example of file handling,java bean,and networking.I need must.
thank u sir
it is a good material for serialization….
easily understandable
[…] have seen enough about using the default protocol to implement serialization and how instances are created during serialization. In this current article we shall see about […]
Please tell me the difference between serialization, transcient and clonable?.
hey really nice blog… keep it up
very good explanation
thank u….
please tell how to serialize object in xml
thanks to make us understand.
please tell how we use xml in serialization.
and how we convert object to xml form.
Excellent Material.
[…] is an interface that enables you to define custom rules and your own mechanism for serialization. Serializable defines standard protocol and provides out of the box serialization […]
Hi Joe , can you explain why strings are immutable in java???
awesome Tutorial for Serialization
@rahul have a look at java string
Very neat and clear explanation which never read.
its very nice def….
Hi,
This is very helpful example….
Thanks
Sunil Kumar
Good
Nice way of presentation.
I have a question regarding de-serialization.
First you serialize a object SerializationBox and write it to a file outfile.ser. On a different JVM i take the outfile.ser file and try to deserialize it using readObject() but i get java.lang.ClassNotFoundException as there is no class file sent.
How does it reconstruct the object and type cast to the SerializationBox object?
I know the file contains Class information as shown in the hex-format. but how do you write code to use readObject and typecast to the serialized object.
SerializationBox reconstructedSBX = SerializationBox ois.readObject();
Excellent work Joe!!
– Shyam :)
Thanks..Very Helpfull document..
Thank you, it was good and very helpfull…
i have a small doubt:
1)difference between creating a thread by extending Thread class and by implementing Runnable interface? Which one should prefer?
please help me
Hi Ganesh,
I as know we should prefer implementing Runnable always as you don’t need to create multiple abject of your class to create multiple threads when required.
There may be other uses/advantages of it.
If we save the object state in a file in one system, then how we will get back the same state in another system to rebuild the object from the same state
please answer this properly with detail
if we declare a type as transient how it is not serialized ..pls explain..
Wonderful.. thank you Sir please keep on writing.
I am waiting for your more blogs.
thanks a lot!!
I was little confused before but as per your explaination it is now clear for me.
Thanks!!!!!
Nice representation of serialized object will look like. Thanks.
Hi Joe,
Awesome Tutorial on Serialization.
I never see the easiest tutorial like yours.
Thanks
Chintan
Good Explaination.
thanks for a deep explanation .it really necessary for all java developers.
thanks a lot.
Thanx for the good article. It helped me a lot. But there are some errors in bytecode:
42 is `B` which means byte (not a private),
00 10 73 65 72 69 61 6c 69 7a 61 62 6c 65 50 72 6f 70 – property name `serializableProp` in modified UTF-8
78 – TC_ENDBLOCKDATA – classAnnotation (empty, no annotations)
70 – TC_NULL – superClassDesc (superclass is Object)
Great
Thanks
thanks
thanku so much
great…………..
.
insert java values(‘1′,’
Java Interview Questions
With the notable increase in jobs that involve extensive use of Java it certainly is worth devoting an entire section to this programming language. Of course, this is not a complete and thorough guide to Java, so some basic knowledge of Java is assumed. What’s being presented here are some salient questions that cover a broad range of topics in Java.
Unless you are interviewing for a position that involves extensive use of Java, you generally won’t be asked Java-related questions. But if you know that the position requires at least some Java skills, you will definitely want to read this section.
‘)
sorry joe i was trying to make how to create comment box ..so i have done this non sense
good explaination
Just Superb
Simple, understandable and clear.
Thanks
Hi Joe,
I have some doubt…
suppose I have a class that implements Serializable interface. Inside the class I don’t writes the code to persisting the object state like in your code
public static void serialize(String outFile, Object serializableObject)
throws IOException {
FileOutputStream fos = new FileOutputStream(outFile);
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(serializableObject);
}
public static Object deSerialize(String serilizedObject)
throws FileNotFoundException, IOException, ClassNotFoundException {
FileInputStream fis = new FileInputStream(serilizedObject);
ObjectInputStream ois = new ObjectInputStream(fis);
return ois.readObject();
}
So, my questions is, with the above scenario, is this a valid implementation of serialization? if Yes, then how JVM persist the state of object?
nice blog…very helpful….thnx..
will u plz enlighten us on 1 more point..
y serializable interface is used though it dont hav ny methods?????
Thanks for giving the detail of Serialization.i have better understand the serialization through your tutorial.but what is marker interface?Explain briefly.
Please reply me………….
very well explained
Great!!!!!!! Explanations are clear and simple
are all the functions of java implicitly virtual????
wow…now,its very easy for me to explain in my exam.. thanxx Joe……
-Pramit(9723916656)
very nice explanation so far i have surfed over. Thanks a lot.
-Rajasekhar
sir i have one doubt
which action java platform restrict on applet?
hi
without implements Serializable i can able to write and read the object in standalone as well as in network using ObjectOutputStream and ObjectInputStream. Please explain, is it must to implements Serializable interface. waiting for your reply.
hi,
Sorry to ask previous question.i Serializable the arrayList and vector objects.so i confused, now only saw that the both original class implements Serializable..
Hi,
Thanks & nice to see good work,
Its very much useful
Regards,
Jeevan
Hi…
I hav a doubt..can we serialize a object without any property ie A class without any property?
Ex–
class A implements Serializable{
}
Class Test{
public static void main(String ag[]){
—-code for serialzation of class A–
}}
Can it will serialize?
Hi,
Thanks a lot for providing such good technical stuff.
very very nice example
Wow.. What a presentation JOE.
Real good work. Hope to see other topics as well..!!
Amazing explanation.. Thanks
Hi Joe,
Nice articles, reading continously from 2 hours,
Your style of writing article makes it interesting.
I think ,I am in a new world of java.
Keep it up .
compact and very good artical.
1 Question:
If we serialize a object in one JDK version and try to deserialize it in different JDK version; it is get deserialized?
You should also mention that upon deserialization if the compiler finds the class missing/unreachable for the serialized object, it’ll throw an Exception. It becomes the responsibility of the deserializing code to include the appropriately required classes.
suppose there are 2 User A and B, if A impliments serialization in one java class(means writting instance variable on socket and passing it to another side) and pass it over the network and User B sends one java class instance variable using socket to another side without serializing its class
now at recevier side User C is receving data so what ADVANTAGE will get to User C when it recevies data from User A
there is no methods and no fields in serializable interface.how can store a state of an object.
please explain i am confuse.
Good work joe, we heartily appreciate this. :)
Nice tutorial., keep doing the good work..,
Very nice tutorial..
I used to go through your blog before my interview,. Thank you so much for explaining clearly,.. Great Work,.. Keep going
very good to have a site like this to understand the java concepts in very simple and clear way . thanks to blog owner
Aree joe anna…mast cheppivanee conceptuu…ne article kiraak unnai anna…gitlanee ee blog continue cheyi…
wow..what an explanation about serilalization..u made it easy..
can u plz tell me the exact difference between wildcard and bounded variable in collections….
nice…very usefull
nice…very usefull
Your explanations help me a lot…
Thanks and keep writing…
Hey,
I have understood the Serialization and deSerialization how does internally works in java.
Thanks for explaining the core stuff.
I have one doubt that is eating my head.
I am holding an ArrayList . I would not like to serialize the 3 rd element from an my ArrayList. How to do that?
List li = new ArrayList();
li.add(“one”);
li.add(“two”);
li.add(“three”);
Could tell me.
Thanks,
-Siva
Thanx for such a nice article.. good luck
Very good article Joe. I know what exactly serialization is. But, as a programmer, I was always interested to see the serialization file and it’s format and header and stuff. This blog is really good and informative. Keep up the good work :)
Hi Shiva,
You can declare some String literal for third element and declare it as transient.
Ex:
transient String third_ele = “three”;
Hope this works :)
very nice….thanx..!!
good explanation fro serialization .Very nice thanx.
It would be nice to have a subtopic on readObject() and writeObject() methods from ObjectInputStream and ObjectOutputStream. Without that the topic can not be complete.
Sir,
Your concepts are really super.
I have one doubt about serialization.
Why we need to serialize object?
When we use in real time?
Please answer.
Can you please Explain how can we append an objects to a file which already contains some objects… and i want to read all objects
it gives an StreamCorrupted Exception ..when i try to read an objects i recently appended……
I had never understood Serilaization this better
Nice explanation. Simple & easy to understand.
very nice explation serialization
Never came across such detailed explanation of Serialization. Thanks for sharing. Awesome blog.
I have a strange question for you. What is the need for sending the object over the network. Why we need to send. Can you please explain with an example or rough design
A classic use case would be in integration projects.
When i compile the code which you have given am getting the output as 10. I thought the object was deserialized. But if i comment the method deserialize and execute the above method , it is returning as 10. please clarify Joe for my below code,i would like to serilaze the object
SerializationBox serialB = new SerializationBox();
serialize(“serial.out”, serialB);
// SerializationBox sb = (SerializationBox) deSerialize(“serial.out”);
// System.out.println(sb.getSerializableProp());
System.out.println(serialB.getSerializableProp());
It is said that static fields cannot be serialized.
public class SerializableExample implements Serializable{
private static Integer staticInteger = new Integer(0);
public static void main(String[] args) {
SerializableExample example = new SerializableExample();
example.setStaticInteger(20);
FileOutputStream fileOutputStream;
try {
File file = new File(“/tmp/test.out”);
fileOutputStream = new FileOutputStream(“/tmp/test.out”);
ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream);
objectOutputStream.writeObject(example);
FileInputStream fileInputStream = new FileInputStream(“/tmp/test.out”);
ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream);
SerializableExample serializableExample1 = (SerializableExample) objectInputStream.readObject();
System.out.println(serializableExample1.getStaticInteger());
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (ClassNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public static Integer getStaticInteger() {
return staticInteger;
}
public static void setStaticInteger(Integer staticInteger) {
SerializableExample.staticInteger = staticInteger;
}
}
The above program should have returned 0 . But it returns 20.
you are performing both serialization and deserialization on same class.As Static variables are class variable the value is still 20.try deserialization in other class
State is nothing but the values stored in member variables of a class. Class has two things state and behavior, State is nothing but member variables and Behavior are the member functions of a class. For example consider a Employee class where states/variables will be name, age, id etc., whereas doWork(), applyLeave() will be behavior of the class.
Good teaching sir. But I have a doubt. Can you explain why we need to implement serializable interface as we are not creating any bond? Can you please explain what we achieve by implementing serializable interface.