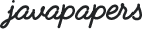
Lambda expression in Java 8, enables to pass a functionality as a method argument. Lambda expressions is one of the hot topics in Java 8, which is about to be released. Few months back, I wrote article for introduction to Lambda expressions. In this article I will be presenting few examples using which we can understand Lamda expressions better. Needless to say, we need JDK 8 to work with lambda expressions and I used JDK 1.8 b115 Early Access Release.
This is a basic usage of lambda expression. HelloWorld is in an interface and on the fly at main method, we are providing a in implementation for that interface. After implementation, we use that instance and invoke its single methods. There will be always only one method and so the lambda expression need not specify the method name.
package com.javapapers.java8; public class LambdaHelloWorld { interface HelloWorld { String hello(String name); } public static void main(String[] args) { HelloWorld helloWorld = (String name) -> { return "Hello " + name; }; System.out.println(helloWorld.hello("Joe")); } }
This is another basic level code showing how we can access the local and class level variables in a lambda expression. Also note how we have passed reference for the Runnable.
package com.javapapers.java8; public class LambdaVariableAccess { public String wildAnimal = "Lion"; public static void main(String[] arg) { new LambdaVariableAccess().lambdaExpression(); } public void lambdaExpression(){ String domesticAnimal = "Dog"; new Thread (() -> { System.out.println("Class Level: " + this.wildAnimal); System.out.println("Method Level: " + domesticAnimal); }).start(); } }
Slightly getting advanced with this example. We have got couple of implementations for the circle interface and they two different operations with respect to context. Those anonymous class implementations itself are passed as argument to another generic method, thus achieving a level of generic function.
package com.javapapers.java8; public class LambdaFunctionArgument { interface Circle { double get(double radius); } public double circleOperation(double radius, Circle c) { return c.get(radius); } public static void main(String args[]){ LambdaFunctionArgument reference = new LambdaFunctionArgument(); Circle circleArea = (r) -> Math.PI * r * r; Circle circleCircumference = (r) -> 2 * Math.PI * r; double area = reference.circleOperation(10, circleArea); double circumference = reference.circleOperation(10, circleCircumference); System.out.println("Area: "+area+" . Circumference: "+circumference); } }
package com.javapapers.java8; import java.util.concurrent.Callable; public class LambdaInitialization { public static void main(String args[]) throws Exception{ Callable[] animals = new Callable[]{()->"Lion", ()->"Crocodile"}; System.out.println(animals[0].call()); } }
In this example the key is we are passing a method reference to another method call. The comparator implementation is passed to Arrays.sort as argument.
package com.javapapers.java8; import java.util.Arrays; public class LambdaExpressionSort { public static void main (String[] ar){ Animal[] animalArr = { new Animal("Lion"), new Animal("Crocodile"), new Animal("Tiger"), new Animal("Elephant")}; System.out.println("Before Sort: "+Arrays.toString(animalArr)); Arrays.sort(animalArr, Animal::animalCompare); System.out.println("After Sort: "+Arrays.toString(animalArr)); } } class Animal { String name; Animal(String name) { this.name = name; } public static int animalCompare(Animal a1, Animal a2) { return a1.name.compareTo(a2.name); } public String toString() { return name; } }
Predicates mixes well with the lambda expressions. We can have a generic implementation and pass predicate as argument in lambda expression. Based on the condition, operation is performed inside the lambda implementation.
package com.javapapers.java8; import java.util.ArrayList; import java.util.List; import java.util.function.Predicate; public class LambdaPredicate { public static int add(ListnumList, Predicate predicate) { int sum = 0; for (int number : numList) { if (predicate.test(number)) { sum += number; } } return sum; } public static void main(String args[]){ List numList = new ArrayList (); numList.add(new Integer(10)); numList.add(new Integer(20)); numList.add(new Integer(30)); numList.add(new Integer(40)); numList.add(new Integer(50)); System.out.println("Add Everything: "+add(numList, n -> true)); System.out.println("Add Nothing: "+add(numList, n -> false)); System.out.println("Add Less Than 25: "+add(numList, n -> n < 25)); System.out.println("Add 3 Multiples: "+add(numList, n -> n % 3 == 0)); } }
Comments are closed for "Java Lambda Expression Examples".
[…] Lambda Expression Examples – Long list of examples, sure you will enjoy! […]
Sir, please link the previous paper on lambda expressions. Even for every topic plz give the link to previous post on same topic
sry sir my brwsr mstke… you provided the link
thanks a lots for sharing this one to all.
It would have been better to just make a point on default methods as all these are included in the same JSR 335.
It would be helpful, if you add the IDE that you use. I think right now, only Intellij and Netbeans support JDK8. Eclipse CDT is not available for JDK8.
Nevertheless, good and clean article to introduce to Lambda.
Thanks
[…] the last tutorial, I gave a list of examples for Java lambda expressions. Which will give detail on different ways of using lambda expressions and I recommend you to go […]
Hi
I have tried last example mentioned under section 6.Predicates and Lambda Expressions but i had reversed the condition of statement System.out.println(“Add Less Than 25: “+add(numList, n -> n n > 25)); and it results 0 which is bit surprising.
Can you please explain what would be reason for it ?
What is hello in first example.
We have used helloworld.hello(‘joe’);
It is looking like method name.
Got it :)
[…] click to a functionality, then lambda expressions will make the code look neat and compact. Refer Java Lambda expression examples to learn […]
Good Work Joe.. keep it up.
Thanks Gootam.
Kindly change it to n -> n> 25
Quick question on the code snippet on example “5. Lambda Expression Sorting”
I see that you are passing animalCompare function as argument. But the Animal Class is not implementing comparator interface, is it a miss or lambda magic?
Hi Joe,
First i want to say thank you for sharing this post.can you explain about the purpose of lambda expression’s.?
Thanks a lot sir, really it is a very nice post….