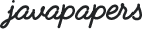
Have you ever encountered a surprise while using toLowerCase()? This is a widely used method when it comes to strings and case conversion. There is a nice little thing you should be aware of.
toLowerCase() respects internationalization (i18n). It performs the case conversion with respect to your Locale. When you call toLowerCase(), internally toLowerCase(Locale.getDefault()) is getting called. It is locale sensitive and you should not write a logic around it interpreting locale independently.
import java.util.Locale; public class ToLocaleTest { public static void main(String[] args) throws Exception { Locale.setDefault(new Locale("lt")); //setting Lithuanian as locale String str = "\u00cc"; System.out.println("Before case conversion is "+str+" and length is "+str.length());// Ì String lowerCaseStr = str.toLowerCase(); System.out.println("Lower case is "+lowerCaseStr+" and length is "+lowerCaseStr.length());// i?` } }
In the above program, look at the string length before and after conversion. It will be 1 and 3. Yes the length of the string before and after case conversion is different. Your logic will go for a toss when you depend on string length on this scenario. When your program gets executed in a different environment, it may fail. This will be a nice catch in code review.
To make it safer, you may use another method toLowerCase(Locale.English) and override the locale to English always. But then you are not internationalized.
So the crux is, toLowerCase() is locale specific.
Comments are closed for "Java’s toLowerCase() has got a surprise for you!".
Very new information I have never heard before. Because, it is common method that had been used by everybody. This post is very useful.
Thank you.
That’s great, i have never heard of it before.
I agree with Vincy, useful and new information.
Keep posting.
Is the behavior standard across all conditions?
Instead of using \u00cc during string initialization, i just changed string to be cc and it gave me same length regardless of whether i set locale or not.
Am i doing anything wrong?
Very good information.
Excellent article. Good to know such deep secrets of Java…Plz keep posting more such things…
Thanks for this new information.
By reading the title I was guessing that the article will be about String immutability but I was wrong.
New Concept. Thanks
Very interesting.
Keep posting.
interesting…
This is really interesting and new to me. Keep it up. thanks
Really a good work. With your blogs I come to know about little internal things of JAVA.
Intersting concept. Thanks for the information
I am learning java…in this location i found more useful articles ..this is best …thank you
Thanks………
Thanks
Thanks for this hidden information…
Same is true with toUpperCase aswell
Never heard of it before..very well
Yes it is nice catch
Intresting. Good job Joe.
cool…this is informative
This blog is really cool buddy.
Interesting never heard of it before..very well
interesting fact..
I don’t know if somebody else has already said what I’m going to say, but: interesting…
Never heard before,, good one!!!