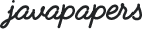
Google cloud messaging (GCM) is an Android platform API provided by Google for sending and receiving push notifications to and from an Android application. This is one of the most important arsenal in an Android developer’s armory.
Let us consider an email android application, it is not efficient to have the application ping the server to check for updates regularly. Mobile devices’ resources should to be used wisely as they are scarce.
Push and pull are two types of notifications using which messages are transferred. In this android tutorial, I will walk you through setting up push notification setup between an application server and an Android mobile application using Google cloud messaging (GCM) API.
This tutorial will have a complete working setup having,
Interacting with Google cloud messaging server (GCM) is not difficult, all we need to do is get couple of configurations perfect and code wise just few number of lines. Overall setup involves IP Address, network proxy/firewall, API key and json objects which sounds like it will be difficult. Get a cup of green tea, start this on a positive note and we will get this done in half an hour.
Custom web application will connect to Google cloud messaging API url and send notification message to GCM server to push it to the registered Android device.
RegId uniquely identifies the Android device to communicate. From the Android app (client), we will make the registration and get the RegId from the Google GCM server. Then, we should communicate that RegId to the web application via some defined process.
In this tutorial, let us provide a callback url in the web application and the Android device should invoke that url to share GCM RegId. In the web application, get the RegId and persist it to a file.
The example presented with this tutorial demonstrates minimal features and in future tutorials we will see about advanced capabilities of GCM.
I have written server application for Google cloud messaging in both Java and PHP. You can use either Java or PHP.
In PHP its just a single file web application, just put it in your Apache.
<?php //generic php function to send GCM push notification function sendPushNotificationToGCM($registatoin_ids, $message) { //Google cloud messaging GCM-API url $url = 'https://android.googleapis.com/gcm/send'; $fields = array( 'registration_ids' => $registatoin_ids, 'data' => $message, ); // Google Cloud Messaging GCM API Key define("GOOGLE_API_KEY", "AIzaSyDA5dlLInMWVsJEUTIHV0u7maB82MCsZbU"); $headers = array( 'Authorization: key=' . GOOGLE_API_KEY, 'Content-Type: application/json' ); $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_POST, true); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt ($ch, CURLOPT_SSL_VERIFYHOST, 0); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($fields)); $result = curl_exec($ch); if ($result === FALSE) { die('Curl failed: ' . curl_error($ch)); } curl_close($ch); return $result; } ?> <?php //this block is to post message to GCM on-click $pushStatus = ""; if(!empty($_GET["push"])) { $gcmRegID = file_get_contents("GCMRegId.txt"); $pushMessage = $_POST["message"]; if (isset($gcmRegID) && isset($pushMessage)) { $gcmRegIds = array($gcmRegID); $message = array("m" => $pushMessage); $pushStatus = sendPushNotificationToGCM($gcmRegIds, $message); } } //this block is to receive the GCM regId from external (mobile apps) if(!empty($_GET["shareRegId"])) { $gcmRegID = $_POST["regId"]; file_put_contents("GCMRegId.txt",$gcmRegID); echo "Ok!"; exit; } ?> <html> <head> <title>Google Cloud Messaging (GCM) Server in PHP</title> </head> <body> <h1>Google Cloud Messaging (GCM) Server in PHP</h1> <form method="post" action="gcm.php/?push=1"> <div> <textarea rows="2" name="message" cols="23" placeholder="Message to transmit via GCM"></textarea> </div> <div><input type="submit" value="Send Push Notification via GCM" /></div> </form> <p><h3><?php echo $pushStatus; ?></h3></p> </body> </html>
/gcm/gcm.php is the home page using which we can send a push notification to Google cloud messaging server. /gcm/gcm.php?shareRegId=1 is the callback url and can be invoked from an external application (like the client Android app), which wants to share the GCM Registration id (RegId). On invocation, RegId will be written to a file.
Note: PHP is optional. If you prefer Java, use the following as server.
For this tutorial, I have implemented a HTTP based App Server and have used gcm-server a Helper library for GCM HTTP server operations. It has only two files. Deploy and run using Tomcat. You can download this as a web application project bundle below.
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <% String pushStatus = ""; Object pushStatusObj = request.getAttribute("pushStatus"); if (pushStatusObj != null) { pushStatus = pushStatusObj.toString(); } %> <head> <title>Google Cloud Messaging (GCM) Server in PHP</title> </head> <body> <h1>Google Cloud Messaging (GCM) Server in Java</h1> <form action="GCMNotification" method="post"> <div> <textarea rows="2" name="message" cols="23" placeholder="Message to transmit via GCM"></textarea> </div> <div> <input type="submit" value="Send Push Notification via GCM" /> </div> </form> <p> <h3> <%=pushStatus%> </h3> </p> </body> </html>
package com.javapapers.java.gcm; import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import com.google.android.gcm.server.Message; import com.google.android.gcm.server.Result; import com.google.android.gcm.server.Sender; @WebServlet("/GCMNotification") public class GCMNotification extends HttpServlet { private static final long serialVersionUID = 1L; // Put your Google API Server Key here private static final String GOOGLE_SERVER_KEY = "AIzaSyDA5dlLInMWVsJEUTIHV0u7maB82MCsZbU"; static final String MESSAGE_KEY = "message"; public GCMNotification() { super(); } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doPost(request, response); } protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { Result result = null; String share = request.getParameter("shareRegId"); // GCM RedgId of Android device to send push notification String regId = ""; if (share != null && !share.isEmpty()) { regId = request.getParameter("regId"); PrintWriter writer = new PrintWriter("GCMRegId.txt"); writer.println(regId); writer.close(); request.setAttribute("pushStatus", "GCM RegId Received."); request.getRequestDispatcher("index.jsp") .forward(request, response); } else { try { BufferedReader br = new BufferedReader(new FileReader( "GCMRegId.txt")); regId = br.readLine(); br.close(); String userMessage = request.getParameter("message"); Sender sender = new Sender(GOOGLE_SERVER_KEY); Message message = new Message.Builder().timeToLive(30) .delayWhileIdle(true).addData(MESSAGE_KEY, userMessage).build(); System.out.println("regId: " + regId); result = sender.send(message, regId, 1); request.setAttribute("pushStatus", result.toString()); } catch (IOException ioe) { ioe.printStackTrace(); request.setAttribute("pushStatus", "RegId required: " + ioe.toString()); } catch (Exception e) { e.printStackTrace(); request.setAttribute("pushStatus", e.toString()); } request.getRequestDispatcher("index.jsp") .forward(request, response); } } }
Following zip file contains the GCM Java server web application eclipse project.
Following Android application is a GCM client and it will,
1. Google Play Services in SDK: is required for Google cloud messaging. Check your Android SDK Manager if you have installed it. It is in the bottom below Extras in the SDK Manager as shown below.
2. Google APIs: we need “Google APIs” installed. I am using Android 4.3 with API 18. Check under your Android API version for Google API.
3. Google-Play-Services_Lib project as Android dependency: Import Google play services lib project into workspace (if you use Android Studio skip this step). In Eclipse, Click File > Import, select Android > Existing Android Code into Workspace. This project should be available as part of the android-sdk download. After importing we need to add this project as dependency to the Android application we are going to create.
4. Google Project Id: In the previous part during custom web application, we created a project in the Google console for creating the Google API server key. Google will assign a project Id when doing that. We need to use that Google project Id to register this Android application with the GCM server.
5. Register/login with a Google account in the AVD: We need to create an Android virtual device (emulator) with the Target as Google API. Then start the emulator, go to list of apps, click ‘Settings’, then ‘Add account’ and login with your Google/Gmail id.
The complete project with source is given for download below.
We have two buttons in this activity, first one is to register this Android application with the Google cloud messaging server and second one is to share the RegId with the web application.
On click of the Register button, we will use the Google Project ID to register with Google cloud messaging server and get the GCM RegId.
Second button is to share this RegId with our custom web application. On click the custom application’s callback url will be invoked. I am running the web application in same machine localhost. But we should not use localhost in the url, since we are connecting from the Android virtual device, as it will loopback to the AVD itself. We should use the our local-ip.
After sharing the Google cloud messaging RegId to web application, we will forward to the next activity.
package com.javapapers.android; import java.io.IOException; import android.app.Activity; import android.content.Context; import android.content.Intent; import android.content.SharedPreferences; import android.content.pm.PackageInfo; import android.content.pm.PackageManager.NameNotFoundException; import android.os.AsyncTask; import android.os.Bundle; import android.text.TextUtils; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.Toast; import com.google.android.gms.gcm.GoogleCloudMessaging; public class RegisterActivity extends Activity { Button btnGCMRegister; Button btnAppShare; GoogleCloudMessaging gcm; Context context; String regId; public static final String REG_ID = "regId"; private static final String APP_VERSION = "appVersion"; static final String TAG = "Register Activity"; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_register); context = getApplicationContext(); btnGCMRegister = (Button) findViewById(R.id.btnGCMRegister); btnGCMRegister.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View arg0) { if (TextUtils.isEmpty(regId)) { regId = registerGCM(); Log.d("RegisterActivity", "GCM RegId: " + regId); } else { Toast.makeText(getApplicationContext(), "Already Registered with GCM Server!", Toast.LENGTH_LONG).show(); } } }); btnAppShare = (Button) findViewById(R.id.btnAppShare); btnAppShare.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View arg0) { if (TextUtils.isEmpty(regId)) { Toast.makeText(getApplicationContext(), "RegId is empty!", Toast.LENGTH_LONG).show(); } else { Intent i = new Intent(getApplicationContext(), MainActivity.class); i.putExtra("regId", regId); Log.d("RegisterActivity", "onClick of Share: Before starting main activity."); startActivity(i); finish(); Log.d("RegisterActivity", "onClick of Share: After finish."); } } }); } public String registerGCM() { gcm = GoogleCloudMessaging.getInstance(this); regId = getRegistrationId(context); if (TextUtils.isEmpty(regId)) { registerInBackground(); Log.d("RegisterActivity", "registerGCM - successfully registered with GCM server - regId: " + regId); } else { Toast.makeText(getApplicationContext(), "RegId already available. RegId: " + regId, Toast.LENGTH_LONG).show(); } return regId; } private String getRegistrationId(Context context) { final SharedPreferences prefs = getSharedPreferences( MainActivity.class.getSimpleName(), Context.MODE_PRIVATE); String registrationId = prefs.getString(REG_ID, ""); if (registrationId.isEmpty()) { Log.i(TAG, "Registration not found."); return ""; } int registeredVersion = prefs.getInt(APP_VERSION, Integer.MIN_VALUE); int currentVersion = getAppVersion(context); if (registeredVersion != currentVersion) { Log.i(TAG, "App version changed."); return ""; } return registrationId; } private static int getAppVersion(Context context) { try { PackageInfo packageInfo = context.getPackageManager() .getPackageInfo(context.getPackageName(), 0); return packageInfo.versionCode; } catch (NameNotFoundException e) { Log.d("RegisterActivity", "I never expected this! Going down, going down!" + e); throw new RuntimeException(e); } } private void registerInBackground() { new AsyncTask() { @Override protected String doInBackground(Void... params) { String msg = ""; try { if (gcm == null) { gcm = GoogleCloudMessaging.getInstance(context); } regId = gcm.register(Config.GOOGLE_PROJECT_ID); Log.d("RegisterActivity", "registerInBackground - regId: " + regId); msg = "Device registered, registration ID=" + regId; storeRegistrationId(context, regId); } catch (IOException ex) { msg = "Error :" + ex.getMessage(); Log.d("RegisterActivity", "Error: " + msg); } Log.d("RegisterActivity", "AsyncTask completed: " + msg); return msg; } @Override protected void onPostExecute(String msg) { Toast.makeText(getApplicationContext(), "Registered with GCM Server." + msg, Toast.LENGTH_LONG) .show(); } }.execute(null, null, null); } private void storeRegistrationId(Context context, String regId) { final SharedPreferences prefs = getSharedPreferences( MainActivity.class.getSimpleName(), Context.MODE_PRIVATE); int appVersion = getAppVersion(context); Log.i(TAG, "Saving regId on app version " + appVersion); SharedPreferences.Editor editor = prefs.edit(); editor.putString(REG_ID, regId); editor.putInt(APP_VERSION, appVersion); editor.commit(); } }
package com.javapapers.android; import android.app.Activity; import android.content.Context; import android.os.AsyncTask; import android.os.Bundle; import android.util.Log; import android.widget.Toast; public class MainActivity extends Activity { ShareExternalServer appUtil; String regId; AsyncTaskshareRegidTask; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); appUtil = new ShareExternalServer(); regId = getIntent().getStringExtra("regId"); Log.d("MainActivity", "regId: " + regId); final Context context = this; shareRegidTask = new AsyncTask () { @Override protected String doInBackground(Void... params) { String result = appUtil.shareRegIdWithAppServer(context, regId); return result; } @Override protected void onPostExecute(String result) { shareRegidTask = null; Toast.makeText(getApplicationContext(), result, Toast.LENGTH_LONG).show(); } }; shareRegidTask.execute(null, null, null); } }
package com.javapapers.android; import android.app.Activity; import android.content.ComponentName; import android.content.Context; import android.content.Intent; import android.support.v4.content.WakefulBroadcastReceiver; public class GcmBroadcastReceiver extends WakefulBroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { ComponentName comp = new ComponentName(context.getPackageName(), GCMNotificationIntentService.class.getName()); startWakefulService(context, (intent.setComponent(comp))); setResultCode(Activity.RESULT_OK); } }
This Android intent service extends the IntentService.
package com.javapapers.android; import android.app.IntentService; import android.app.NotificationManager; import android.app.PendingIntent; import android.content.Context; import android.content.Intent; import android.os.Bundle; import android.os.SystemClock; import android.support.v4.app.NotificationCompat; import android.util.Log; import com.google.android.gms.gcm.GoogleCloudMessaging; public class GCMNotificationIntentService extends IntentService { public static final int NOTIFICATION_ID = 1; private NotificationManager mNotificationManager; NotificationCompat.Builder builder; public GCMNotificationIntentService() { super("GcmIntentService"); } public static final String TAG = "GCMNotificationIntentService"; @Override protected void onHandleIntent(Intent intent) { Bundle extras = intent.getExtras(); GoogleCloudMessaging gcm = GoogleCloudMessaging.getInstance(this); String messageType = gcm.getMessageType(intent); if (!extras.isEmpty()) { if (GoogleCloudMessaging.MESSAGE_TYPE_SEND_ERROR .equals(messageType)) { sendNotification("Send error: " + extras.toString()); } else if (GoogleCloudMessaging.MESSAGE_TYPE_DELETED .equals(messageType)) { sendNotification("Deleted messages on server: " + extras.toString()); } else if (GoogleCloudMessaging.MESSAGE_TYPE_MESSAGE .equals(messageType)) { for (int i = 0; i < 3; i++) { Log.i(TAG, "Working... " + (i + 1) + "/5 @ " + SystemClock.elapsedRealtime()); try { Thread.sleep(5000); } catch (InterruptedException e) { } } Log.i(TAG, "Completed work @ " + SystemClock.elapsedRealtime()); sendNotification("Message Received from Google GCM Server: " + extras.get(Config.MESSAGE_KEY)); Log.i(TAG, "Received: " + extras.toString()); } } GcmBroadcastReceiver.completeWakefulIntent(intent); } private void sendNotification(String msg) { Log.d(TAG, "Preparing to send notification...: " + msg); mNotificationManager = (NotificationManager) this .getSystemService(Context.NOTIFICATION_SERVICE); PendingIntent contentIntent = PendingIntent.getActivity(this, 0, new Intent(this, MainActivity.class), 0); NotificationCompat.Builder mBuilder = new NotificationCompat.Builder( this).setSmallIcon(R.drawable.gcm_cloud) .setContentTitle("GCM Notification") .setStyle(new NotificationCompat.BigTextStyle().bigText(msg)) .setContentText(msg); mBuilder.setContentIntent(contentIntent); mNotificationManager.notify(NOTIFICATION_ID, mBuilder.build()); Log.d(TAG, "Notification sent successfully."); } }
package com.javapapers.android; public interface Config { // used to share GCM regId with application server - using php app server static final String APP_SERVER_URL = "http://192.168.1.17/gcm/gcm.php?shareRegId=1"; // GCM server using java // static final String APP_SERVER_URL = // "http://192.168.1.17:8080/GCM-App-Server/GCMNotification?shareRegId=1"; // Google Project Number static final String GOOGLE_PROJECT_ID = "512218038480"; static final String MESSAGE_KEY = "message"; }
package com.javapapers.android; import java.io.IOException; import java.io.OutputStream; import java.net.HttpURLConnection; import java.net.MalformedURLException; import java.net.URL; import java.util.HashMap; import java.util.Iterator; import java.util.Map; import java.util.Map.Entry; import android.content.Context; import android.util.Log; public class ShareExternalServer { public String shareRegIdWithAppServer(final Context context, final String regId) { String result = ""; MapparamsMap = new HashMap (); paramsMap.put("regId", regId); try { URL serverUrl = null; try { serverUrl = new URL(Config.APP_SERVER_URL); } catch (MalformedURLException e) { Log.e("AppUtil", "URL Connection Error: " + Config.APP_SERVER_URL, e); result = "Invalid URL: " + Config.APP_SERVER_URL; } StringBuilder postBody = new StringBuilder(); Iterator > iterator = paramsMap.entrySet() .iterator(); while (iterator.hasNext()) { Entry param = iterator.next(); postBody.append(param.getKey()).append('=') .append(param.getValue()); if (iterator.hasNext()) { postBody.append('&'); } } String body = postBody.toString(); byte[] bytes = body.getBytes(); HttpURLConnection httpCon = null; try { httpCon = (HttpURLConnection) serverUrl.openConnection(); httpCon.setDoOutput(true); httpCon.setUseCaches(false); httpCon.setFixedLengthStreamingMode(bytes.length); httpCon.setRequestMethod("POST"); httpCon.setRequestProperty("Content-Type", "application/x-www-form-urlencoded;charset=UTF-8"); OutputStream out = httpCon.getOutputStream(); out.write(bytes); out.close(); int status = httpCon.getResponseCode(); if (status == 200) { result = "RegId shared with Application Server. RegId: " + regId; } else { result = "Post Failure." + " Status: " + status; } } finally { if (httpCon != null) { httpCon.disconnect(); } } } catch (IOException e) { result = "Post Failure. Error in sharing with App Server."; Log.e("AppUtil", "Error in sharing with App Server: " + e); } return result; } }
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.javapapers.android" android:versionCode="1" android:versionName="1.0" > <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.GET_ACCOUNTS" /> <uses-permission android:name="android.permission.WAKE_LOCK" /> <permission android:name="com.javapapers.android.permission.C2D_MESSAGE" android:protectionLevel="signature" /> <uses-permission android:name="com.javapapers.android.permission.C2D_MESSAGE" /> <uses-permission android:name="com.google.android.c2dm.permission.RECEIVE" /> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> <uses-permission android:name="android.permission.VIBRATE" /> <uses-sdk android:minSdkVersion="9" android:targetSdkVersion="16" /> <application android:icon="@drawable/ic_launcher" android:label="@string/app_name" > <activity android:name=".RegisterActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name="com.javapapers.android.MainActivity" android:configChanges="orientation|keyboardHidden" android:label="@string/app_name" > </activity> <receiver android:name=".GcmBroadcastReceiver" android:permission="com.google.android.c2dm.permission.SEND" > <intent-filter> <action android:name="com.google.android.c2dm.intent.RECEIVE" /> <action android:name="com.google.android.c2dm.intent.REGISTRATION" /> <category android:name="com.javapapers.android" /> </intent-filter> </receiver> <service android:name=".GCMNotificationIntentService" /> </application> </manifest>
On sending a successful push notification to Google cloud messaging server:
On successful receive of notification from GCM:
Following zip file contains the Android application eclipse project.
Comments are closed for "Google Cloud Messaging GCM for Android and Push Notifications".
Thanks Firoz. Try this and let me know the results :-)
Great tutorial, but i have one little problem, whenever I click on send message button from web and when the internet is off in the device notification won’t come, but as soon as I turn on internet connectivity the previous message what I have sent when the connectivity was off is not coming, please help me out. Thanks.
What if the client does not have a google account registered ?
Its required only if we are using Android Virtual Device (Emulator) to run the Client (GCM Android App).
If its a real mobile device, then its not required.
Kyle,
Using Google GCM, two-way communication is possible. Two-way means, application-server to mobile via GCM-server and vice versa. In this tutorial, I have covered the application-server to mobile via GCM-server part only. For the other, I am planning to write another tutorial.
It is possible to send broadcast to multiple mobiles from an application via GCM.
hi.. i have tried every step on the above tutorial and run the app on a real mobile not on an emulator, but every time i send message on server to mobile i get this {“multicast_id”:4968672681542797991,”success”:1,”failure”:0,”canonical_ids”:0,”results”:[{“message_id”:”0:1391001084908870%28678a6af9fd7ecd”}]}
but i was not able to receive any notification on my mobile. help please
Hello thanks for this tutorial. i have a problem thou when i connect to the webservice in php the txt file is always empty. In the android side i saw that in lolcat the regid is empty also. Do you why this is hapenning? i just downloaded your project and change the senderId,google api key,and urls… In android side it says it shared succsessfully to server and it does but the regId is an empty string
Granadillo,
Are you running in a real device or AVD?
Do you see any exceptions in the log?
Log.d("RegisterActivity", "GCM RegId: " + regId);
In Android side, this line should print the GCM-RegId in log. Just for debugging purpose, copy that Reg-Id and paste it in the server-side text file which is now empty even after sharing.
Then check what happens…
I have used GoogleCloudMessaging object to register my app instead of GCMRegistrar object. Messages are sent from my server to gcm server. But from gcm server to my device (android app), not a single message/notification is received. I also tested points from checklist. Can you please help me to find out what i’m missing ?
Yes Mr. Ravooru. server url is used on application server is correct and i also have verified that message is sent to gcm server. Only thing is notifications are not received on my android device.
Hi Joe:
Not sure if this is a bad question but wanted to know can an android app be both a GCM server and GCM client. This would remove the need to have server implementation and would allow virtual P2P (App-to-App) communication.
If this is not possible, then what could be the possible ways to achieve P2P communication without the need of hosting a server?
Hi Joe, this is a fantastic tutorial. But I am having an issue. similar to this one.
I have an app which shows map and button on it. I want to notify the user’s current location to another mobile, when I click on that button. (just like facebook chat. when One friend sends message to second friend, second friend gets notified)
How do I do that? Please help!
hi joe,
i tried running the android app on a real tablet. It does not return a reg-id string. Please note that have not set up the server (App server) side code yet and just testing communication flow between my app and GCS (Google Cloud Server).
hi joe,
after following your tutorial line by line, everything works correctly except that i do not get a reg id. Currently, i am only implemented the android app gcs server but not the entire app gcs server app server. Could that be a reason?
Hi Joe,
I have set all correctly as per the document, everything is fine. But, the regId is returning empty after registering. Dono, where am going wrong. Please help on this issue..
Regards
Ashwin Ganesh.R
Even im looking for the same exact concept of yours, if u got any idea, plz let me know. thanks in advance.
You are saying us to add google play services library and using the old gcm lib in code. GCMRegistrar is not even available on google play services. What a stupid.
Hi Joe,
You haven’t started service.If the application is not running i.e exited then how notification is received.
{“multicast_id”:8162060780822142071,”success”:0,”failure”:1,”canonical_ids”:0,”results”:[{“error”:”InvalidRegistration”}]}
getting this error using php. have done the following 2 things in yr code:
1. Put my API key in the gcm.php file instead of what was there.
2. Created a file called GCMRegId.txt with my project id in it..
Please help! Appreciate it!
It was Network port problem. Firewall was blocking network ports 5228,29,30. 5228 is default port used by GCM. I unblocked that port to fix the issue. Thanks a lot you for the help
i am not getting regId please help
hi i am not getting regId can you please help me..
Guys,
I have made major changes now to the tutorial sample application / code. Previously I had used some deprecated API instead of GoogleCloudMessaging and also improved the error handling. Please check the latest and comment if you have any issues.
Are you getting any errors. Carefully parse the application log. For sure there will be some traces.
Previously I had used some deprecated API instead of GoogleCloudMessaging and also now improved the error handling. Please check the latest and comment if you have any issues.
1. You need to build a web application (GCM server).
2. Android Application that will POST GCM Notification message to above Server.
3. Now server will broadcast to all other Android apps those should receive the location (which will have broadcast intent receiver). – is the GCM Android client given in above example.
Please go through the above tutorial. You may need one hour to understand and execute.
Downloading the projects and running it can be done in minutes.
I have made major changes now to the tutorial sample application / code. Previously I had used some deprecated API instead of GoogleCloudMessaging and also improved the error handling. Please check the latest and comment if you have any issues.
Sorry Partha, that’s my mistake.
I was trying too many things and posted some wrong version.
Please check the latest now and comment if you have any issues.
Gregor,
You need not create GCMRegId.txt manually. When you run the Android App (client), it will invoke the callback url in the server-side. Then the server application will create the text file with device RegId in it.
Can you please elaborate the issue. Are you not getting in the device side from the GCM-cloud server? or in the application server side?
Post errors/trace if any.
Hi Joe,
I am getting the reg_id but when i am trying to post my reg_id to my application server it is giving POST failure status:400. What am i doing wrong?
Nilesh,
The URL you are using to post to the GCM-server is malformed. Check if the URL is right.
hi i want to implement gcm for my android appln.
i want to use device to device messaging ….
means one android device can send directly message to other android device … without using third party server….
is it possible…
if yes plse help me…..
i have all android device id….
Paramvir,
I am working on that device to device communication tutorial. Will post it in a week.
[…] an earlier tutorial Google Cloud Messaging GCM for Android and Push Notifications, we discussed and worked on an example on how to send a notification message from an application […]
Hi Joe,
I successfully get the following message from my server
{“multicast_id”:5034742404093744888,”success”:1,”failure”:0,”canonical_ids”:0,”results”:[{“message_id”:”0:1394109528288191%31e4cc17f9fd7ecd”}]}
But i didn’t receive any notifications on my device. When i try to send notification from my server, my app was exited on device at the same time.
Kindly do some needful.
Hi Joe,
I am using software to make my localhost public and i am now able to share reg_id with the server. But when i send a message, I receive GCM notification stating ‘Message Received from Google GCM Server:null’.
What could i be doing wrong?
Satheesh,
In the Android app side, check for log messages. Once you receive the message bundle it will print log message stating that it has received message. When your app crashes it will also leave trace in log. Share all those here and lets find out.
‘Message Received from Google GCM Server:null’ – this is shown in the Android App UI (Intent).
Can you check the Android log for error messages?
Hi Joe,
I have the same problem. I tried to send “asdsdad” from the form, and it shown {“multicast_id”:7879656234367994045,”success”:1, “failure”:0,”canonical_ids”:0, “results”:[{“message_id”: “0:1394166356544304%31e4cc17f9fd7ecd”}]}
Then, a few seconds later, I got a GCM Notification: “Message Received from Google GCM Server:null”. When I click on that, it shown “RegId shared with Application server. RegId:Null”
I tried to send several times, and until it shown error in the php side “{“multicast_id”:5824038452200434731,”success”:0, “failure”:1,”canonical_ids”:0, “results”:[{“error”:”InvalidRegistration”}]}”
I checked the file GCMRegId.txt, and I found that its content was deleted.
In the LogCat, it shown:03-06 23:41:40.427: I/GCMNotificationIntentService(925): Received: Bundle[{m=asdsdad, from=1033922223757, android.support.content.wakelockid=9, collapse_key=do_not_collapse}]
And I can see the true message “asdsdad” in the log.
It is working now, in your code one change is required in Config.java.
String MESSAGE_KEY = “message”; it should be String MESSAGE_KEY = “m”; That fixed the issue for me.
Tony,
Great!
The message key should be same as in the server-side and Android client-side. With respect to Google GCM it has got nothing to do. :-)
Hi,
Thanks for the best GCM tutorial out there! I am using a server on the web and am getting an Unauthorized message when I try to send a message through the php. I’ve checked both the API key and my IP is listed in Google, I can’t seem to find the problem. Could it have something to do with that my server is not related to my ip, or do you have any ideas?
Thanks,
Jake
Hi Joe,
I have done this tutorial, but if I touch the notification from the status bar, the regId in GCMRegId.txt is deleted, and the content of that file is replaced by “null”, and I have to click on “register…” and “share…” buttons to make work again. And another question is how to make sound when the notification comes, since if the phone is in screen timeout state, the notification comes silently and does not turn the phone screen on. How can I fix those things? Thank you Joe!
Guyz.. if you are doing everything perfectly and not getting notifications on your device..though
you get MessageId from GCM server..
Your incoming packets to device must be blocked by your forewall… Try using real device with its own internet connection and not the one using WiFi.
Or try to run the same project at your home with a personal WiFi connection.. (y)
Sir,i am getting an exception in ShareExternalServer class….i.e IOException is occuring when i am trying to register the app with the web server…what could be the problem ?
Hi , it’s a good tutorial , hmm , is there any other way to push a notification in android without using google cloud messaging(GCM)?. i want to create an app that will notify all user’s in android and iOS, but i don’t know where to start. Anyway TIA.
Sir, thanks for the tutorial..I have a problem, My client side indicate that regid successfully send the to webserver. But icant receive regid in my webserver(it is in java).what are the possible chances for this pblm? Can u please me!!
Hello
I am facing the following issue.
“Registered with GCM Server.Error:
SERVICE_NOT_AVAILABLE”
It looks like I am not able to connect eith GCM service correctly. Please provide me the solution.
I am facing the same issue. I am testing on a real phone without a sim card. Is this the reason?
android app working fine but app server in php doent work properly…
could you please help in this topic?
hello joe..i am getting notifications..but i have 2 problems remaining.
1.Notification can show what i typed in php using MESSAGE_KEY variable.
but application is not understanding MESSAGE_KEY
2.Tell me how to update PHP file for storing multiple reg id s ??
kindly help me urgently coz i have to show my project at 31st of march…
i am trying to run the app using web apps t(servlet)
but its troom wing exception GCMRegId.txt not found,from where configure the text file GCMRegId.txt
what is the way to use in miobile……plz rpl
I am getting this on server:
{“multicast_id”:4739377494510639849,”success”:1,”failure”:0,”canonical_ids”:0,”results”:[{“message_id”:”0:1395843739625862%31e4cc17f9fd7ecd”}]}
But on an emulator wen i send msg 4m server it stops working. Evn on real device its happening the same. Also am getting the same RegID on emulator and real device.. Plz help.. I need it urgently
Evn am having same problem.. Did u solve it?
This example Android app is working for me and for many others too.
There might be something missing in your configuration. Need more detail to help you.
Can you check the log and post the detail like exception trace.
See, the same thing again. Can you please be specific about the error you get.
For 1. what is the error you get?
For 2. Hope you have read my other tutorial https://javapapers.com/android/android-multicast-notification-using-google-cloud-messaging-gcm/
I have given the code for multiple reg ids in Java. Instead of rewriting the regid.txt file everytime, you need to append the regids and second thing is the request should be appended and posted to Google GCM server.
Please avoid sms language, its difficult to read.
So the message is successfully sent by the GCM-server application and the GCM-client (Android app) is not receiving it. Is my understanding right?
If so, when the Android app stops working, can you watch the log and report what you get?
whenever I send message from server the app crashes and i get these errors
1. MESSAGE_KEY=”m” . and i see real value of m(strings i typed in php)only in notification.my activity shows ‘m’ as value of MESSAGE_KEY.
for 2. i have uploaded php to 000webhost which is free it doesnt support jsp.so plssss.. tell me what to do with php.please tell me how to edit that php for storing reg ids in regid.txt
plus 3. GCM forgets my device in a minute or 2.and i have to re-register after nearly 5 minutes..
4. can i use GCM for sending alerts to more than a million devices???? tell me when u will be online..we ll sort this out.because i have to show some progress in my project on 29th of march.
thank u joe..u r like a boon to newbies. :)
com.javapapers.android.GcmBroadcastReceiver: java.lang.ClassNotFoundException:
i guess ..there is some mistake in importing library services.
Check the IP address of the GCM application server and see if you can reach it.
Using a free-hosting webhost to learn a new technology will not workout. They may not give you enough privilege and it will be always behind a strong firewall. Debugging will be an issue always.
Why not try in your local machine. For the Google GCM server, if you are a Java guy, use the same sample I have given and it should work with only Android server key and project id change.
If you wish to work based on PHP, you my try XAMPP to create a PHP setup in local and work based on it. This setup can be done in minutes.
Please throw away the Internet free-hosting stuff for these kind of tutorial based learning. As I have stated in my tutorial above, Google GCM project is all about setting up the environment and configurations. Code is very simple. So, get your local environment fixed first. Once things are running and then you can see about hosting it live :-)
I can register with the GCM server and I can share my GCM RegID with the app server. However when I try and send a message I get the following error:
Warning: file_get_contents(GCMRegId.txt): failed to open stream: No such file or directory in /Library/WebServer/Documents/gcm/gcm.php on line 37
So it looks like GCMRegId.txt is not being created. Any ideas?
Thanks
Great tutorial by the way
thanx joe…i get the notifications..everything is fine.but i need your guidance for next step.which is ..
1.i want to show what i typed in server in an activity.
for example if i send “hii” from server ..for php $message=hiii
and in config.java file MESSAGE_KEY=”message”
the problem is “hii” is only seen in notifications.when i try to pass that string in an activity it sees “hii” as “message” and as a result i see “message” as value of MESSAGE_KEY
i want to show in the activity what i typed in server after clicking the notification..
as u suggested, for multiple devices i will try java in my localhost but as i get notifications properly, i dont think firewalls are an issue .so adding multiple reg Ids to php array seems simple to me :)
hey joe…i did it.. all previous questions are solved ..
i didnt know about the ‘msg’ variable in notification..
i want an activity containing various images which is opened after respected alert is clicked.. how to..?
Thanks David.
Looks like the GCMRegId.txt file is not getting created.
Can you manually create this file and keep it empty at the application-server root and then try?
so happy that you are writing a tutorial for that soon…if possible plssssss upload the tutorial this week..or tell me when will u upload that?i am working for an application which will help finding missing people,criminals.. :)
Hey! Awesome tutorial, thanks for it.
I’m now facing a problem tho.
The app gets gerID from GCM and it is able to send it to our server and the php server application is able to write it into the text file as supposed to.
The problem comes when I try to send push message trough the php app.
I get this:
{“multicast_id”:5760807111590163335,”success”:0,”failure”:1,”canonical_ids”:0,”results”:[{“error”:”MismatchSenderId”}]}
What could be causing this?
Thank you.
Solved. Had to reinstall the app.
Hi Joe,
Thanks for your tutorial, very useful. And I have run successfully in my app client site, which shows “RegID shared with Application Server”. However, I have two little problem: (I am using Java)
1. the link for the java should be
“xxx/GCMNotification.java?shareRegId=1”;
instead of
“xxx/GCMNotification?shareRegId=1”;
otherwise, I would get a “404 error”
2. I have problem of the server site, actually I am little focusing about the data process of the web server. How can I get the result page (with the regID) automatically? I can only open the index.jsp page, but how to let the result shows like yours? I can’t do that, please give some advice:)
Cheers,
Sky
how to send message from Android device to Google Cloud Messaging server..?
[…] just starting with Google Cloud Messaging (GCM), I recommend you to go through the earlier tutorial Google Cloud Messaging GCM for Android and Push Notifications. It gives you more detailed step by step instruction to get started with Google Cloud Messaging. In […]
What are the problems behind “error:InvalidRegistration”??Only the incorrect reistration id?
I tried to send messages to an android app. every time i got the same output .that is shown below:
{“multicast_id”:9115386997854774649,”success”:0,”failure”:1,”canonical_ids”:0,”results”:[{“error”:”InvalidRegistration”}]}
I am sure that the registration id is correct. yet i don’t know why it happens so..
hello joe,
there is no error but..
i see my alerts sent from server in activity.but i see first alert only even if i try to view new alerts.
i copied my code in onresume() method but nothing happened.tell me how do i set text dynamically with respect to alerts.i have to reinstall the app again n again in order to see updated alerts….
make sure that it was shared with ur app server.and make sure u allow any IPs in your google console settings
Have got internet on emulator..i’ve checked that still it shows
“Registered with GCM Server.Error:
SERVICE_NOT_AVAILABLE”
I haven’t coded server part just want to get reg_id first bt am unable to get that due to above stated error
make sure you have added all the permissions.and your emulator is connected to the internet.last option:try turning off ur fierewalls.
thank’s this tutorial is good
i use this tutorial to make my gcm service,
this tutorial successfully work on my android emulator but after i tray on my real device my apps cannot get the gcm notification.
anyone can help me?
thank’s this tutorial is good
i use this tutorial to make my gcm service,
this tutorial successfully work on my android emulator but after i tray on my real device my apps cannot get the gcm notification.
anyone can help me?
When I run GCM server application, the following error occurs
RegId required: java.io.FileNotFoundException: GCMRegId.txt (The system cannot find the file specified)
please let me know any answer.
I had implemented GCM Appliction It works fine in emulator but while comming to the Mobile device it shows GCM error even after adding google account to my phone
Just a few tips for success in this example:
-Remember, when you change the data in Config.java or other in this Android project, Build the project another time to apply the changes!!
-BEWARE! IT’S A MISTAKE, YOU’LL NEED TO CORRECT THIS: Change parameter to send notificacion to GCM in PHP (in my case) app server: $message = array(“m” => $pushMessage); to —> $message = array(“message” => $pushMessage);
-And yeah, at the first try be in localhost.
Thank you very much for the tutorial, Joe.
I have a question. In GCMNotificationIntentService.java there is the message’s handler. Well, there is a thread sleep, I’m wondering why this 5×3 seconds of wait is necessary. It’s a performance requeriment?? or a simulation of massive usage of the system???
Commented this bucle, the response becomes immediate.
Zach Baudrey, I think you need to correct the mistake I was talking about.
You should check the key using which the message is retrieved at the Android client side. It should be same as being sent from the GCM Server side. May be, you should print the complete message in the Android log and check.
I’m sorry but I’m having problems with your tutorial . . .
I can’t seem to open GCM-App-Sever in Eclipse. After unziping I tried to import it into a new workspace but Eclipse says it’s not a project. I tried opening it directly in Eclipse but the same thing. What could I be doing wrong.
Dear Joe,
The ‘Java GCM Server Web Application’ package seems, not a complete enclipse androis project. I could not ‘import’ it as android project in eclipse.
But ‘client’ application project is getting imported in Eclipse.
Is anything wrong in my approach? Please guide me in loading the server application.
You have issue with dependency. Check in section-3 under “GCM Android App Prerequisite”.
Registration id generated succefully in adroid device…
But when run Java Application it gives following error.
RegId required: com.google.android.gcm.server.InvalidRequestException: HTTP Status Code: 401
Help plz…
Hi,
I want to Send current location from my Device to Application Server as a response to the Request made by Application Server.
Example :
Consider a Passenger waiting for a Driver
1. Passenger Requests to Application Server from its app,
2. Application Server Requests the Driver on its app,
3. Driver’s Location is Sent to Application Server.
What I figured out through GCM is we can Send / Recieve Messages to GCM. I just Want to know How we can send Location Through GCM.
Hello Dear jeo,
I have successfully register regId and send message and
get follwing output
“[ messageId=0:1397796580428924%31e4cc17f9fd7ecd ] ”
But i could not receive message in emulator…
I am getting following “error log”
“connectionClose : no XMPPConnection – That’s strange!”
Please help.
How can I make sure that it is shared with my app server? Im using MAMP and in the config.java file, i enter the url as my ip address and then gcm/gcm.php?shareRegId=1 which is the path to the file. Still I get the same error as mentioned above. The url to the server is correct, as when i try to connect to other php files from the same ip address, they work perfectly fine.
I am uses php implementation for the server.
Sorry, if my question is too trivial. I am a total newbie to android development. Your help would be most appreciated.
I got this error:
{“multicast_id”:4926266773825479032,”success”:0,”failure”:1,”canonical_ids”:0,”results”:[{“error”:”MismatchSenderId”}]}
Please help.. I am sure that i have provide correct project id and api key
hi, i got this error after pushing the notification “HTTP Status 404 – /GCM-App-Server/GCMNotification”.any one tell me what might be the reason?
Thank you I too got same issue and solved with uninstall and reinstall
Thank you for the great tutorial.
I have three things to mention, one has already been posted:
1st:
I used the php-server code and therefore i had to change the “message” to “m” in config.java (android app), otherwise I also received the notification “Message Received from Google GCM Server:null”
2nd:
After the first push notification via the webserver it seems that the content in the “GCMRegId.txt” is replaced with the value “null”
I fixed it that way, that after the first app-share request (which is successfull) i commented the following code snippet “out”.
/*
if(!empty($_GET[“shareRegId”])) {
$gcmRegID = $_POST[“regId”];
file_put_contents(“GCMRegId.txt”,$gcmRegID);
echo “Ok!”;
exit;
}
*/
3rd:
in the php server code I replaced the line
with
because otherwise in the URL bar it will look like that gcm.php/gcm.php/?push=1 after to requests, but with the replacement the url keeps the same.
last i have to mention, that in my app i receive every message correctly, but when i click at the notification i always get the message “RegId: null”, but everything is working fine :-)
Thanks a lot. GCM is perfectly working for me. Nice post. It is really useful for the beginners who works on GCM
Hi Jeo,
My app crashes with an error java.lang.NoClassDefFoundError
com.google.android.gms.gcm.GoogleCloudMessaging
com.sanju.cloudmessaging.RegisterActivity.registerGCM(RegisterActivity.java:79)
com.sanju.cloudmessaging.RegisterActivity$1.onClick(RegisterActivity.java:46)
at this line GoogleCloudMessaging.getInstance(this);
I have been trying from two days your not replying me .reply me waiting for your reply.
Thank you for the comprehensive tutorial.
[…] Cloud Messaging, I recommend you to go through the introductory tutorial which discusses about notifications in Android using Google cloud messaging. This linked introductory tutorial explains the Google GCM concept with an example and it covers […]
Thx for this helpful tutorial! Helped me a lot :-)
HI Jeo,
I am sanjeev have been asking you about my errors from last week you didn’t reply me.Finally I solved my errors now i can send and receive the messages and thanks for your tutorial.I have learned some thing new about GCM.Thanks for your GCM Example.
I have some problem but I cant. I created GCMRegId.txt on my route file, GCMRegId.txt with gcm.php on the same file. And I write my message and I click send but its turning error, like this :
( ! ) Warning: file_get_contents(GCMRegId.txt): failed to open stream: No such file or directory in C:\wamp\www\gcmnew\gcm.php on line 41
Call Stack
# Time Memory Function Location
1 0.0039 263920 {main}( ) ..\gcm.php:0
2 0.0039 264792 file_get_contents ( ) ..\gcm.php:41
Curl failed: Failed connect to android.googleapis.com:443; No error
Hi Joe,
first of all thanks for the blog.
we are trying to implement push notification service for ADF mobile. and used your server side code to send notification to android device. We are able to receive the notification alert(alert sound with client app) but message is null. We are not able to receive message. could you please suggest where we might did wrong?
here is my onMessage() method implementation(Invoked when a Push Notification arrives)
public void onMessage(Event event) {
//Parsing the payload JSON string
JSONBeanSerializationHelper jsonHelper = new JSONBeanSerializationHelper();
try {
PayloadServiceResponse serviceResponse =
(PayloadServiceResponse)jsonHelper.fromJSON(PayloadServiceResponse.class, event.getPayload());
Map session = (Map)AdfmfJavaUtilities.evaluateELExpression(“#{applicationScope}”);
String newMsg = serviceResponse.getCustomMessage();
session.put(“pNewMessage”, newMsg);
} catch (Exception e) {
e.printStackTrace();
}
}
we are using #{applicationScope.pNewMessage} in our UI to show the notification message, but we are only receiving notification alert(alert sound) but not message. Please suggest.
I am running it on my mobile and sending all the swear words one by one from the net. Everything is working perfectly fine. Thank You Joe.
There was a minor typo in the code in the config.java file I suppose due to which the message received was ‘null’ earlier. But it has been corrected in the comments.
static final String MESSAGE_KEY = “m”;
Once again, hats off for Joe. :D
I am having a problem registration in GCM saying SERVICE_NOT_AVAILABLE, until I found this, I copy the code provided in here and it’s working really just fine now. Great!
I have same problem, means here is no error getting in logcat. and i also have verified that message is sent to gcm server. But not getting any notofication on my device, I had already test on emulator and on real device. I have followed the all step from scretch to end but not getting notification. Pls help, i am trying this by last week. Thnks
hello joe
this blog is really awesome. all Android tutorials are great.
i m getting some issue in this Google GCM setup.
i have followed all your instructions carefully.
my query is what should i write in config.java in APP_SERVER_URL variable, as my php is in localhost and i know my ip is 127.0.0.1 , but it not working when i browse through Android emulator
also where do i get regid? and where do i put it? also gcmregid.txt was not created auto, so i created empty file manually in gcm folder near gcm.php file .
in config.java do we have to use project id or project number in google_project_id variable?
i will be really glad if you help get the Google cloud messaging work for me.
thanks
Hey Joe
I follow this Google cloud messaging tutorial. I wrote gcm server part using java in Netbeans. I set the APP_SERVER_URL = “http://10.0.2.2:8084/GCMServer/GCMNotification”;
I set the ip as loopback address and delete “?shareRegId=1” from url.
Now my problem is when i click on Register with Google GCM Server it shows me the Regid:jshjkjcc….. and when i click on Share GCM-RegId with App Server it goes to the main Android activity and shows me the message RegId shared with Application Server. RegId:jshjkjcc…..
Now when i write something on GCM Server application and click on Send Push Notification via GCM it shows me the error “RegId required: java.io.FileNotFoundException: GCMRegId.txt (The system cannot find the file specified)”
What is wrong with my code..
This Google GCM project code is working fine, i appreciate the effort. Mysteriously i ran into a problem with this script. Whenever u minimize or stop (onstop or ondestroy) after closing network access, strange toast message coming repeatedly. I re-read entire code, but nowhere i see this toast code.
Toast Error: “Failed to Load Database, Please Restart Application”
Toast messages is appearing only after exiting the app + network failure (No internet access)
i want to remove that source code. Above all, this is the best Google Cloud Messaging notification tutorial all through the net, thanks.
I think you should you GCM Php with Wamp. This way is the best way, I tried GCM-App-Server but I failed,so I used php and it worked. But I have a problem. My mobile doesn’t recieve any notification and now I’m trying to solve it :(
Create a file with the name GCMRegId.txt in c:\folder\GCMRegId.txt and the change the path in GCM Server app as c:/folder/GCMRegId.txt
Hello Amrinder
I follow this tutorial but it does not working for me. Can you please help me. When I click on “Send Push Notification via GCM” it shows me the following output
[ messageId=0:1403074150101397%31e4cc17f9fd7ecd ] and does not show any notification on AVD. Please help me
Hello Ravi
I follow this tutorial but it does not working for me. Can you please help me. When I click on “Send Push Notification via GCM” it shows me the following output
[ messageId=0:1403074150101397%31e4cc17f9fd7ecd ] and does not show any notification on AVD. Please help me
Hello Sir!
Please help me. When I click on “Send Push Notification via GCM” it shows me the following output
[ messageId=0:1403074150101397%31e4cc17f9fd7ecd ]. It does not show the output like
{“multicast_id”:7048577678678825845,”success”:0,”failure”:1,”canonical_ids”:0,”results”:[{“error”:”InvalidRegistration”}]}
Please tell me what is the issue?
While sending 250 characters of data push mesg shows only single line(i.e 30 to 40 char). How can i Display total data in mesg atleast 150 characters.
Hello, best tutorial for beginner, work perfect
Hello, best Google Cloud Messaging tutorial for beginner, works perfect
you should edit : ‘$message=array(“m”=>utf8_encode($pushMessage)); ‘ in php file to send special character
Hello Sir!
Now I am getting the GCM output {“multicast_id”:5163471844000799180,”success”:1,”failure”:0,”canonical_ids”:0,”results”:[{“message_id”:”0:1403507025702682%31e4cc17f9fd7ecd”}]}
but not getting any Google gcm notification on Android AVD or real Android device
First off, thank you for this Google Cloud Messaging GCM tutorial. So far I’m getting all of it. Very helpful!
However, I did run into an issue…
I go to gcm.php and then send a push back to the registered phone. Your code calls the php file with a forward slash “gcm.php/?push=1” With that I receive a 404 error (using nginx).
OK, it must be that slash. Right? So I changed it to “gcm.php?push=1” No 404, but now the page just come up blank; not even a failure message.
Any tips?
Note: Server IP / API Key / Project ID / GCMRegId.txt have been triple check for correctness.
i am using it with java and getting an error as RegId required: java.io.FileNotFoundException: GCMRegId.txt (No such file or directory)
The problem is emulator does not have proper Time Set and it also doesnt have SIM, so google cant register it. Those who are facing SERVICE_NOT_AVAILABLE, try running your app on real phone with a SIM. It will work fine.
Hello, Thank you for this great Google Cloud Messaging tutorial, it’s the best on the net.
my problem is everything is good i send the message i got the GCM notification on my Android phone but not the message that i’ve written. just the gcm notification with the title “Message Received from Google” when i click on it it gives me the Toast “RegId shared with application server RegId null” where can i find the message?
Is it necessary to unblock port no. 5228-5230 for this application.
Hi Joe!
Thank you for this tutorial which helps me a lot!
But I have some problem with the project.
I tried the php server, but I didn’t receive the reg id in the GCMRegId file from the Android application.
I have the 401 error.
Even if I copied the reg id from the logcat and pasted it in the GCMRegId file, the 401 error persists.
I forgot to tell you that I use a real device, an Android tablet.
I don’t know how I can do to solve these issues.
Thank you for helping me!
Hi,
I found my problem.
In Google developer console, I put the ipv6 for the “edit allowed ip”!
Thank you very much for this tutorial!!
Thanks for this great tut brother…
Some notes:
– Its not Project ID use Project Number
– Change MESSAGE_KEY = “message” to MESSAGE_KEY = “m”
– change action=”gcm.php/?push=1″ to change action=”gcm.php?push=1″
After hours of research, I fixed the issue as follows:
In my case I had to go to Properties->Java Build Path->Order and Export and tick the check box Android Private Libraries, then cleaned the project and worked fine.
I got this error while running gcm-server code please help me out.
can please help me to fix !
Hi Joe,
After the text (in web service) is entered and the message is push. That is the error shown in the web service.
RegId required: com.google.android.gcm.server.InvalidRequestException: HTTP Status Code: 401
While in apps the RegId is shared in web server. Please Help.
Thank you.
***Same problem with Girish
Registration id generated succefully in adroid device…
But when run Java Application it gives following error.
RegId required: com.google.android.gcm.server.InvalidRequestException: HTTP Status Code: 401
hai sir,
previous problem is solved(Activity closed when message push from server).But when i click message on emulator i got “registerId=null” Message send from server is not display.
please help me sir..
hai nadia,
I am also face this issue but now i resolve it. create new gmail account and create new project,server key with all ip allowed,android key with YOUR SH1 FINGERPRINT -FE:F9:DD:58:37:A8:06:B2:52:15:8F:69:24:FE:72:AE:25:E9;com.javapapers.android and used your new project ID and serverkey,androidkey..It may work .. Have nice day
hai pal,
right click the project,In properties–>java buit path->order and export-> fill the check box…
All is well
Fantastic job!!
Really good tutorial, thanks Joe
After few tricky issues I made it work (don forget open 5228, 5229 and 5230 port in your firewall and/or router)
Vasantha, edit the manifest file and provide proper package name prefixes for all activities and permissions
I have done a tutorial for Google GCM multicast notification:
https://javapapers.com/android/android-multicast-notification-using-google-cloud-messaging-gcm/