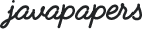
This Android tutorial is to help create tab layout with swipe views in an Android application. Swipe views in a tab layout are for convenience for the user. Swipe adds a real world feel to the tabs.
In a previous tutorial we saw about creating a tab layout in an Android application. In an another tutorial we saw about creating swipe views in Android. Now all we are going to do is combine the both and create a simple app that has tabs and swipe able views.
Following XML is the main layout of the application. We use the ViewPager to implement the Swipe views
<android.support.v4.view.ViewPager xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/pager" android:layout_width="match_parent" android:layout_height="match_parent" />
Following layout contains the individual design for the Tabs
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="New Text" android:id="@+id/textView" android:layout_gravity="center_horizontal" android:layout_marginTop="20dp" /> </LinearLayout>
package com.javapapers.android.swipetablayout.app; import android.app.ActionBar; import android.app.ActionBar.Tab; import android.app.FragmentTransaction; import android.os.Bundle; import android.support.v4.app.FragmentActivity; import android.support.v4.view.ViewPager; public class MainActivity extends FragmentActivity implements ActionBar.TabListener { private ViewPager viewPager; private ActionBar actionBar; private TabPagerAdapter tabPagerAdapter; private String[] tabs = { "Missed Calls", "Dialled", "Received" }; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); viewPager = (ViewPager) findViewById(R.id.pager); tabPagerAdapter = new TabPagerAdapter(getSupportFragmentManager()); viewPager.setAdapter(tabPagerAdapter); actionBar = getActionBar(); actionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS); for (String tab_name : tabs) { actionBar.addTab(actionBar.newTab().setText(tab_name) .setTabListener(this)); } viewPager.setOnPageChangeListener(new ViewPager.OnPageChangeListener() { /** * on swipe select the respective tab * */ @Override public void onPageSelected(int position) { actionBar.setSelectedNavigationItem(position); } @Override public void onPageScrolled(int arg0, float arg1, int arg2) { } @Override public void onPageScrollStateChanged(int arg0) { } }); } @Override public void onTabReselected(Tab tab, FragmentTransaction ft) { } @Override public void onTabSelected(Tab tab, FragmentTransaction ft) { viewPager.setCurrentItem(tab.getPosition()); } @Override public void onTabUnselected(Tab tab, FragmentTransaction ft) {} }
package com.javapapers.android.swipetablayout.app; import android.os.Bundle; import android.support.v4.app.Fragment; import android.support.v4.app.FragmentManager; import android.support.v4.app.FragmentPagerAdapter; public class TabPagerAdapter extends FragmentPagerAdapter { public TabPagerAdapter(FragmentManager fm) { super(fm); } @Override public Fragment getItem(int index) { Bundle bundle = new Bundle(); String tab = ""; int colorResId = 0; switch (index) { case 0: tab = "List of Missed Calls"; colorResId = R.color.color1; break; case 1: tab = "List of Dialled Calls"; colorResId = R.color.color2; break; case 2: tab = "List of Received Calls"; colorResId = R.color.color3; break; } bundle.putString("tab",tab); bundle.putInt("color", colorResId); SwipeTabFragment swipeTabFragment = new SwipeTabFragment(); swipeTabFragment.setArguments(bundle); return swipeTabFragment; } @Override public int getCount() { return 3; } }
package com.javapapers.android.swipetablayout.app; import android.os.Bundle; import android.support.v4.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.TextView; public class SwipeTabFragment extends Fragment { private String tab; private int color; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); Bundle bundle = getArguments(); tab = bundle.getString("tab"); color = bundle.getInt("color"); } @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { View view = inflater.inflate(R.layout.swipe_tab, null); TextView tv = (TextView) view.findViewById(R.id.textView); tv.setText(tab); view.setBackgroundResource(color); return view; } }
Download Android Tab with Swipe View Example Application
Comments are closed for "Android Tab Layout with Swipe Views".
Since 3-4 week i was waiting for this tutorial in my favourite javapapaers…. Thank you so much
thanks you for this post wonderfull
plz sir, post all android version support swipe views tab layout. i try this examples but it support only above androidos version3( support above AP11). plz sir post all version support examples swipe views tab layout(also suppoprt below Ap11)
Sir how to give colour, colour1, colour 2, colour3 because its showing me error
Where do I add stuff to the tabs?? Sorry, beginner here.
Hi Sir,
I have an requirement in my project :
I have 3 tab as shown in this example,Now when user navigates from one tab1 to tab2,I need to perform certain validation, and if all the validations are performed successfully then only user should be allowed to navigate to Tab2.
And also I need to send the i/p data entered by user in tab1 to tab2 as I will save the data at the end of tab2.
Thanks in advance .
Please do the needfull.
Hi, Can we achieve this without FragmentPagerAdapter ? Coz I am already working with “android.app.Fragment” instead of “android.support.v4.app.Fragment”. Please help me in this issue.
Thanks a lot in advance
actionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS);
This statement is giving null pointer exception
Error occurred in style.xml file at parent=….
It’s work fine to me… Thanks u so much..