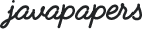
This Android tutorial is to help you build swipe views in an Android app. We will be using FragmentActivity and ViewPager to achieve the swipes. Swipe views are nice way to navigate pages one by one when they are stacked together like a pack of cards.
ViewPager is the layout manager that enables the user to swipe left and right in the view. PageAdapter is the model that controls the data displayed in the swiped pages. ViewPager is used in sync with a Fragment to enable the swipe.
In the previous tutorial we saw about Tab layout in Android. It will be nice to have this swipe views in the tab layout. In the next tutorial, we will see how to achieve that.
The example Android app for this tutorial lists the planets in our solar system. The swipe view allows to flip through the pages.
The main activity is a FragmentActivity. It contains a FragmentPagerAdapter which manages the data displayed in swipe view. In the FragmentPageAdapter a Fragment is used. We have a loaded an image followed by a short description.
package com.javapapers.android.androidswipeableviews.app; import android.os.Bundle; import android.support.v4.app.Fragment; import android.support.v4.app.FragmentActivity; import android.support.v4.app.FragmentManager; import android.support.v4.app.FragmentPagerAdapter; import android.support.v4.view.ViewPager; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.Button; import android.widget.ImageView; import android.widget.TextView; public class MainActivity extends FragmentActivity { static final int NUM_ITEMS = 9; PlanetFragmentPagerAdapter planetFragmentPagerAdapter; ViewPager viewPager; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.fragment_pager); planetFragmentPagerAdapter = new PlanetFragmentPagerAdapter(getSupportFragmentManager()); viewPager = (ViewPager)findViewById(R.id.pager); viewPager.setAdapter(planetFragmentPagerAdapter); Button button = (Button)findViewById(R.id.goto_first); button.setOnClickListener(btnListener); button = (Button)findViewById(R.id.goto_previous); button.setOnClickListener(btnListener); button = (Button)findViewById(R.id.goto_next); button.setOnClickListener(btnListener); button = (Button)findViewById(R.id.goto_next); button.setOnClickListener(btnListener); } private View.OnClickListener btnListener = new View.OnClickListener() { public void onClick(View v) { switch(v.getId()) { case R.id.goto_first: viewPager.setCurrentItem(0); break; case R.id.goto_previous: viewPager.setCurrentItem(viewPager.getCurrentItem()-1); break; case R.id.goto_next: viewPager.setCurrentItem(viewPager.getCurrentItem()+1); break; case R.id.goto_last: viewPager.setCurrentItem(NUM_ITEMS - 1); break; } } }; public static class PlanetFragmentPagerAdapter extends FragmentPagerAdapter { public PlanetFragmentPagerAdapter(FragmentManager fm) { super(fm); } @Override public int getCount() { return NUM_ITEMS; } @Override public Fragment getItem(int position) { SwipeFragment fragment = new SwipeFragment(); return SwipeFragment.newInstance(position); } } public static class SwipeFragment extends Fragment { @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { View swipeView = inflater.inflate(R.layout.swipe_fragment, container, false); TextView tv = (TextView)swipeView.findViewById(R.id.text); ImageView img = (ImageView)swipeView.findViewById(R.id.imageView); Bundle args = getArguments(); int position = args.getInt("position"); String planet = Planet.PLANETS[position]; int imgResId = getResources().getIdentifier(planet, "drawable", "com.javapapers.android.androidswipeableviews.app"); img.setImageResource(imgResId); tv.setText(Planet.PLANET_DETAIL.get(planet)+" - Wikipedia."); return swipeView; } static SwipeFragment newInstance(int position) { SwipeFragment swipeFragment = new SwipeFragment(); Bundle args = new Bundle(); args.putInt("position", position); swipeFragment.setArguments(args); return swipeFragment; } } }
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:padding="4dip" android:gravity="center_horizontal" android:layout_width="match_parent" android:layout_height="match_parent"> <android.support.v4.view.ViewPager android:id="@+id/pager" android:layout_width="match_parent" android:layout_height="0px" android:layout_weight="1"> </android.support.v4.view.ViewPager> <LinearLayout android:orientation="horizontal" android:gravity="center" android:measureWithLargestChild="true" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="0"> <Button android:id="@+id/goto_first" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/first"> </Button> <Button android:id="@+id/goto_previous" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/previous"> </Button> <Button android:id="@+id/goto_next" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/next"> </Button> <Button android:id="@+id/goto_last" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/last"> </Button> </LinearLayout> </LinearLayout>
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@android:color/black"> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/imageView" android:layout_gravity="center_horizontal" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:layout_marginTop="120dp" /> <TextView android:id="@+id/text" android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center_vertical|center_horizontal" android:textAppearance="?android:attr/textAppearanceMedium" android:text="@string/hello_world" android:textColor="@android:color/white" android:layout_alignParentBottom="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:padding="20dp" android:layout_marginBottom="30dp" android:textIsSelectable="false" android:textSize="14sp" /> </RelativeLayout>
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.javapapers.android.androidswipeableviews.app" > <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.javapapers.android.androidswipeableviews.app.MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Added to the above files, we have a Java constant class containing details of the planets. You can find that and the complete source of this Android application in the below download.
Download AndroidSwipeableViews App Project Source
Comments are closed for "Android Swipe Views".
This code showing error in styles.xml file.can you please clarify that.and one more are developing this prorject on eclipse or any other IDE. I am trying to import that in eclipse IDE its giving lot many errors.please help me to resolve this issue…
Thanks you.