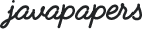
Android platform includes the SQLite embedded database and provides out of the box support to use it via Android APIs. In this tutorial we shall see how to get started with SQLite database in Android. SQLite is nothing but a relational database and our sql skills will help.
To use SQLite in Android, a java class should be created as a sub class of SQLiteOpenHelper. This class will act as a database controller which will have the methods to perform the CRUD operations. This custom java class should override the methods named onCreate() and onUpgrade().
onCreate() method will be called for the first time when the Android application is run. First the database instance should be created using the method like getReadableDatabase() or getWritableDatabase() based on the type of access required. Android supports this method by providing in-built methods. For that, SQLiteQueryBuilder class should be imported.
Download Source of Android SQLite Database
Lets have three Android Activity for List, Add and Edit operations and ensure that these are declared in manifest file. And then we need to create subclass of SQLiteHelper to manage SQLite database.
DBController.java is going to be our custom java class that will manage the SQLite database. We should extend SQLiteOpenHelper and override the essential methods. The constructor is the hook that will be used to setup the database. While running the Android application, the database will be created for the first time.
public DBController(Context applicationcontext) { super(applicationcontext, "androidsqlite.db", null, 1); Log.d(LOGCAT,"Created"); }
SQLiteOpenHelper provides callback methods and we should override it to get our job done. Those callback methods that we can override are onCreate(), onUpgrade(), onOpen() and onDowngrade(). And onCreate() and onUpgrade() are abstract methods and must be overridden.
onCreate(SQLiteDatabase database) – is the method which is called first time when the database is created and we need to use this method to create the tables and populate it as per the need.
@Override public void onCreate(SQLiteDatabase database) { String query; query = "CREATE TABLE animals ( animalId INTEGER PRIMARY KEY, animalName TEXT)"; database.execSQL(query); Log.d(LOGCAT,"animals Created"); }
onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) – is the method called when upgrade is done. We can drop the datbase and reset if required.
@Override public void onUpgrade(SQLiteDatabase database, int version_old, int current_version) { String query; query = "DROP TABLE IF EXISTS animals"; database.execSQL(query); onCreate(database); }
We shall have other user defined methods to handle the sql CRUD operations. The animals table will be created when the onCreate() method is invoked while installing the application. For performing operaations like insert, update, the SQLiteDatabase instance should be created using the methods like getReadableDatabase() or getWritableDatabase(). ContentValues() are used to pass values to the query.
public void insertAnimal(HashMapqueryValues) { SQLiteDatabase database = this.getWritableDatabase(); ContentValues values = new ContentValues(); values.put("animalName", queryValues.get("animalName")); database.insert("animals", null, values); database.close(); }
public HashMapgetAnimalInfo(String id) { HashMap wordList = new HashMap (); SQLiteDatabase database = this.getReadableDatabase(); String selectQuery = "SELECT * FROM animals where animalId='"+id+"'"; Cursor cursor = database.rawQuery(selectQuery, null); if (cursor.moveToFirst()) { do { wordList.put("animalName", cursor.getString(1)); } while (cursor.moveToNext()); } return wordList; }
public int updateAnimal(HashMapqueryValues) { SQLiteDatabase database = this.getWritableDatabase(); ContentValues values = new ContentValues(); values.put("animalName", queryValues.get("animalName")); return database.update("animals", values, "animalId" + " = ?", new String[] { queryValues.get("animalId") }); }
public void deleteAnimal(String id) { Log.d(LOGCAT,"delete"); SQLiteDatabase database = this.getWritableDatabase(); String deleteQuery = "DELETE FROM animals where animalId='"+ id +"'"; Log.d("query",deleteQuery); database.execSQL(deleteQuery); }
Complete code is as follows,
package com.javapapers.android.sqlitestorageoption; import java.util.ArrayList; import java.util.HashMap; import android.util.Log; import android.content.ContentValues; import android.content.Context; import android.database.Cursor; import android.database.sqlite.SQLiteDatabase; import android.database.sqlite.SQLiteOpenHelper; public class DBController extends SQLiteOpenHelper { private static final String LOGCAT = null; public DBController(Context applicationcontext) { super(applicationcontext, "androidsqlite.db", null, 1); Log.d(LOGCAT,"Created"); } @Override public void onCreate(SQLiteDatabase database) { String query; query = "CREATE TABLE animals ( animalId INTEGER PRIMARY KEY, animalName TEXT)"; database.execSQL(query); Log.d(LOGCAT,"animals Created"); } @Override public void onUpgrade(SQLiteDatabase database, int version_old, int current_version) { String query; query = "DROP TABLE IF EXISTS animals"; database.execSQL(query); onCreate(database); } public void insertAnimal(HashMapqueryValues) { SQLiteDatabase database = this.getWritableDatabase(); ContentValues values = new ContentValues(); values.put("animalName", queryValues.get("animalName")); database.insert("animals", null, values); database.close(); } public int updateAnimal(HashMap queryValues) { SQLiteDatabase database = this.getWritableDatabase(); ContentValues values = new ContentValues(); values.put("animalName", queryValues.get("animalName")); return database.update("animals", values, "animalId" + " = ?", new String[] { queryValues.get("animalId") }); } public void deleteAnimal(String id) { Log.d(LOGCAT,"delete"); SQLiteDatabase database = this.getWritableDatabase(); String deleteQuery = "DELETE FROM animals where animalId='"+ id +"'"; Log.d("query",deleteQuery); database.execSQL(deleteQuery); } public ArrayList > getAllAnimals() { ArrayList > wordList; wordList = new ArrayList >(); String selectQuery = "SELECT * FROM animals"; SQLiteDatabase database = this.getWritableDatabase(); Cursor cursor = database.rawQuery(selectQuery, null); if (cursor.moveToFirst()) { do { HashMap map = new HashMap (); map.put("animalId", cursor.getString(0)); map.put("animalName", cursor.getString(1)); wordList.add(map); } while (cursor.moveToNext()); } return wordList; } public HashMap getAnimalInfo(String id) { HashMap wordList = new HashMap (); SQLiteDatabase database = this.getReadableDatabase(); String selectQuery = "SELECT * FROM animals where animalId='"+id+"'"; Cursor cursor = database.rawQuery(selectQuery, null); if (cursor.moveToFirst()) { do { wordList.put("animalName", cursor.getString(1)); } while (cursor.moveToNext()); } return wordList; } }
NOTE: If you are interested only in the core database handling, with this the tutorial is over. What follows is, we are going to create a simple Android app which will provide user interface to operate on the database. Just giving a finishing touch to this database tutorial.
This activity will be launched first, on running this Android database application. The layout for this activity includes a ListView and its entry is supplied by the other xml file. This is done by the ListViewAdapter. The following xml files shows how to list data.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" > <RelativeLayout android:id="@+id/relativeLayout1" android:layout_width="fill_parent" android:layout_height="40dp" android:background="#000000" android:orientation="vertical" > <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="5dp" android:text="Animals" android:textAppearance="?android:attr/textAppearanceLarge" android:textColor="#FFFFFF" /> <Button android:id="@+id/button1" android:layout_width="41dp" android:layout_height="40dp" android:layout_alignParentRight="true" android:layout_alignParentTop="true" android:background="#454545" android:onClick="showAddForm" android:text="+" android:textColor="#FFFFFF" android:textSize="30sp" /> </RelativeLayout> <RelativeLayout android:id="@+id/relativeLayout1" android:layout_width="fill_parent" android:layout_height="match_parent" android:layout_alignParentLeft="true" android:layout_below="@+id/relativeLayout1" android:orientation="vertical" android:layout_marginTop="40dp"> <ListView android:id="@android:id/list" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentLeft="true"> </ListView> </RelativeLayout> </RelativeLayout>
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="vertical" > <TextView android:id="@+id/animalId" android:layout_width="fill_parent" android:layout_height="wrap_content" android:visibility="gone" /> <TextView android:id="@+id/animalName" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginBottom="5dp" android:layout_marginTop="5dp" android:paddingLeft="6dip" android:paddingTop="6dip" android:textColor="#A4C739" android:textSize="17sp" android:textStyle="bold" /> </LinearLayout>
After designing the layout, the list of animals will be retrieved from the database using the java class and it should be the sub class of ListActivity. Then the list of data will be loaded into the design layout using the list adapter as shown below..
ListAdapter adapter = new SimpleAdapter( MainActivity.this,animalList, R.layout.view_animal_entry, new String[] { "animalId","animalName"}, new int[] {R.id.animalId, R.id.animalName}); setListAdapter(adapter);
On clicking each item shown in the ListView, then we will be redirected to a separate layout edit or delete the selected list item. For that, OnItemClickListener should be set to the ListView instance. This will be done by the following code.
ListView lv = getListView(); lv.setOnItemClickListener(new OnItemClickListener() { @Override public void onItemClick(AdapterView> parent, View view,int position, long id) { animalId = (TextView) view.findViewById(R.id.animalId); String valAnimalId = animalId.getText().toString(); Intent objIndent = new Intent(getApplicationContext(),EditAnimal.class); objIndent.putExtra("animalId", valAnimalId); startActivity(objIndent); } });
To add new entry into the animals database, we need to click the add icon shown at the top right corner of the animal list layout.
To Add new animal entry into the table, this activity is used. So the add layout should be designed with the list of widgets as shown as follows
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#CCCCCC" android:orientation="vertical" android:paddingTop="1dp" > <TextView android:id="@+id/textView1" android:layout_width="match_parent" android:layout_height="wrap_content" android:background="#000000" android:padding="5dp" android:text="Add Animal" android:textAppearance="?android:attr/textAppearanceLarge" android:textColor="#FFFFFF" /> <RelativeLayout android:layout_width="match_parent" android:layout_height="match_parent" android:background="#FFFFFF" android:orientation="vertical" android:padding="10dp" > <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_alignParentTop="true" android:layout_marginLeft="24dp" android:layout_marginTop="30dp" android:text="Animal" /> <EditText android:id="@+id/animalName" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignLeft="@+id/textView1" android:layout_below="@+id/textView1" android:ems="10" > <requestFocus /> </EditText> <Button android:id="@+id/btnadd" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignLeft="@+id/animalName" android:layout_below="@+id/animalName" android:layout_marginTop="32dp" android:text="Save" android:onClick="addNewAnimal" /> </RelativeLayout> </LinearLayout> </pre> </p> <p>while clicking the save button, the following method will be invoked.</p> <p> <pre class="prettyprint lang-java"> public void addNewAnimal(View view) { HashMap<String, String> queryValues = new HashMap<String, String>(); queryValues.put("animalName", animalName.getText().toString()); controller.insertAnimal(queryValues); this.callHomeActivity(view); }
In this method, the content of TextView is read and put into hashmap to send as an argument of the DBController methods to perform insert. After insert, the launcher activity will be called to see the recently added item.
The entire logic for the add activity is as shown in the following code
package com.javapapers.android.sqlitestorageoption; import java.util.ArrayList; import java.util.Calendar; import java.util.HashMap; import java.util.List; import java.util.Map.Entry; import com.javapapers.android.sqlitestorageoption.R; import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.view.Menu; import android.view.MenuInflater; import android.view.MenuItem; import android.view.View; import android.widget.ArrayAdapter; import android.widget.DatePicker; import android.widget.EditText; import android.widget.ListAdapter; import android.widget.SimpleAdapter; import android.widget.Spinner; public class NewAnimal extends Activity{ EditText animalName; DBController controller = new DBController(this); @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.add_new_animal); animalName = (EditText) findViewById(R.id.animalName); } public void addNewAnimal(View view) { HashMapqueryValues = new HashMap (); queryValues.put("animalName", animalName.getText().toString()); controller.insertAnimal(queryValues); this.callHomeActivity(view); } public void callHomeActivity(View view) { Intent objIntent = new Intent(getApplicationContext(), MainActivity.class); startActivity(objIntent); } }
It is similar to the add process, only difference is it displays the data from the database. The design and logic is shown by the following code.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#CCCCCC" android:orientation="vertical" android:paddingTop="1dp" > <TextView android:id="@+id/textView1" android:layout_width="match_parent" android:layout_height="wrap_content" android:background="#000000" android:padding="5dp" android:text="Edit Animal" android:textAppearance="?android:attr/textAppearanceLarge" android:textColor="#FFFFFF" /> <RelativeLayout android:layout_width="match_parent" android:layout_height="match_parent" android:background="#FFFFFF" android:orientation="vertical" android:padding="10dp" > <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_alignParentTop="true" android:layout_marginLeft="24dp" android:layout_marginTop="30dp" android:text="Task" /> <EditText android:id="@+id/animalName" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignLeft="@+id/textView2" android:layout_below="@+id/textView2" android:ems="10" > <requestFocus /> </EditText> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignLeft="@+id/animalName" android:layout_below="@+id/animalName" android:layout_marginTop="19dp" android:onClick="editAnimal" android:text="Edit" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignBaseline="@+id/button1" android:layout_alignBottom="@+id/button1" android:layout_centerHorizontal="true" android:onClick="removeAnimal" android:text="Delete" /> </RelativeLayout> </LinearLayout>
package com.javapapers.android.sqlitestorageoption; import java.util.Calendar; import java.util.HashMap; import android.os.Bundle; import android.util.Log; import android.widget.DatePicker; import android.widget.EditText; import com.javapapers.android.sqlitestorageoption.R; import android.app.Activity; import android.content.Intent; import android.view.Menu; import android.view.MenuInflater; import android.view.MenuItem; import android.view.View; public class EditAnimal extends Activity{ EditText animalName; DBController controller = new DBController(this); @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_edit_animal); animalName = (EditText) findViewById(R.id.animalName); Intent objIntent = getIntent(); String animalId = objIntent.getStringExtra("animalId"); Log.d("Reading: ", "Reading all contacts.."); HashMapanimalList = controller.getAnimalInfo(animalId); Log.d("animalName",animalList.get("animalName")); if(animalList.size()!=0) { animalName.setText(animalList.get("animalName")); } } public void editAnimal(View view) { HashMap queryValues = new HashMap (); animalName = (EditText) findViewById(R.id.animalName); Intent objIntent = getIntent(); String animalId = objIntent.getStringExtra("animalId"); queryValues.put("animalId", animalId); queryValues.put("animalName", animalName.getText().toString()); controller.updateAnimal(queryValues); this.callHomeActivity(view); } public void removeAnimal(View view) { Intent objIntent = getIntent(); String animalId = objIntent.getStringExtra("animalId"); controller.deleteAnimal(animalId); this.callHomeActivity(view); } public void callHomeActivity(View view) { Intent objIntent = new Intent(getApplicationContext(), MainActivity.class); startActivity(objIntent); } }
Download Source of Android SQLite Database
Now we have a database! Ho – Ho – Ho! Go ahead and launch this app and enjoy access to SQLite database in Android.
Comments are closed for "Android SQLite Database".
Nice one.. :) Thank u.. :)
really nice
Nice Tutorial . . .
Thank you
after importing your projects i am getting errors. why ?
Dear Joe,
I came acroos your blog, and its very useful. Could you please have a tag for Android, and all the posts are not tagged properly.
Thanks
Senthilkumar .J
Good one Its great that you have in to android.
What is the error you get?
Thanks Senthilkumar.
Now I added ‘Android’ in menu. :-)
Hi Joe
I am following your tutorials from past one year thank you so much for this kind of wonderful tutorials may i know which server is you are using for javapapers like(tomcat)?
Hi Joe,
will you please help me , i am irritating with one error in java in apachetomcat7 starting problem in eclipse , it is worked 4 days back well but now i dont know what happened its not working the error is like ” Server Tomcat v7.0 Server at localhost was unable to start within 45 seconds. If the server requires more time, try increasing the timeout in the server editor.” I changed time in server editor but not solved i re-installed eclipse and server also same problem plzzzzzz help meeee . . . .
thanks for posting this tutorial
Where is the main class? Plz upload the code for the main class
Hi Joe,
Please expand the coding window.Because not able to view frequently,each time scrolling it and saw that so oly.
Thanks
easy to understand nice!
Just want to drop a note of thanks that and let you know your tutorials are awesome. Liked your blog design as well, very neat.
hello sir when i am running my project,there is an error says illegal argument exception ?please help me for solving this error
I need the same one, but instead of list view , i need it in table with two or more columns.
how can i do it.?
nice one !
Joe please help me out..
I need the same one, but instead of list view , i need it in table with two or more columns.
how can i do it.?
Awaiting ur reply
Hello, For the first time i opened your site and when i saw this page i got very much impressed . i hope your tutorials will help me further. can u tell me if i wan to apply background image in android application, then what code would be use?
Thanks
Hi Joe,
Your blog is very useful..can you tel me the difference between getreadabledatabase () and getwritabledatabase() ?
Regards
~kavitha
Excellent work!
cannot add two values in animales table only one value add animalName please check to add more columns in this table
Hi joe,i read your tutorial from last few months…plz provide tutorial for dropbox sync and google drive sync with android…
Hi Joe,
Thanks to Javapapers and for your effort.
I have a problem in Android.
Please provide some solution for this.
I have 3 android applications which will use single database. When i am inserting one record from 1st application it will be reflect in 2nd application.
For this in development process its working. But when i am installing the apk’s in mobile for 2nd apk giving error not installed. Why this error..?Please resolve myproblem.
Thanks & Regards,
Anush.
this is semi help full can u add other crud parts, like updating and deleting
how to add more than one two columns?
Thanks for this helpful post !!I have been searching lots of sqlite tutorials etc but this one’s the best for a novice like me :)
How can we a csv file and then insert it into sqlite database??
pls help
Hi joe,
Thank you very much for nice post.I m new in java as well as Android. It helped me much to under stand the Sqlite functionality . But I have a question “if I want to add image of animal what should I change or add in following code
public void addNewAnimal(View view) {
HashMap queryValues = new HashMap();
queryValues.put(“animalName”, animalName.getText().toString());
controller.insertAnimal(queryValues);
this.callHomeActivity(view);
}
I’hv already add imageView and modify ur animal table adding column name photo Blob. actually I want to know how can get image in Hashmap?
after reading a csv file..how to insert it into sqlite database??
pls help
Very Easy tutorial for beginners…Thanks
nice example of sqlite.
thank u so much for this tutorial.it is very helpful
Excelent ,the best one!
Joe,
I want to run the same App but crating the database on the SD Card ,any suggestion?
Thanks a lot.
mmm…. thats a good idea. Soon I will write about them :-)
I feel nice to see ur website……. it’s nice nd keep it ONNNNNNNNNNNNNNNNN !!!!!!!!!!!!! i think some android quizes should also be here …. :-)
Hi joe…Ur tutorial is really helpful and Ur website design is really cool….
hi joe
Hi Joe,
I need a little assistance here. I am n00b to Android. I am developing an app for my office friends in which I need to sync my app data with a web app running on my office PC.
Q1:- how and where to deploy my web app so that I can use it from my android app using internet.
Q2:- how to write a sync code for my android app.
i need the same one,but want imageview instead of listview
Hi Mr Joe
it’s Easy tutorial for beginners…
Thanks
hi joe good guide!!!! thank’s!!!
I want add 2 Button for moveToNext row and moveToPrevious row…how to select next row for view on EditText???
How TO DO???
I got a problem in main.xml,I’m using the Eclipse IDE
I am following your tutorials since I have started learning android and they are great…I appreciate you work..Thanks a lot for your tutorials
nice tutorial joe! Helps me alot to get start for my new project :))Thanx!!
its good.but i need code for access external database from android apps.if any one know help me to find some thing.
I have been following your for the last 2 years….. I have seen myself developed quite a lot of knowledge in android as well as java… hats off……
hi joe.. thanks a lot for your wonderful blog.. now I have developed a passion for these technologies for android and java..without this site I wouldn’t have climbed the ladder of success in software field.. once again thaks a lot..
Sir,
Your Description on the topics is very nice…
Dear Sir,
Above example is very good to understand.
I am creating sales app on emulator using sqlite
1)Could you please provide me an example for multiple tables?
2)I also need a proper guidance on how to connect Sqlite with remote server database?
Thank you
Thanks a lot for the article. It gave a good insight on how to have an app with DB. But, I have few clarifications. I wish to manually retrieve the DB files from SQLiteManager to see if the tables are created properly? Where is the DB stored and how can it be located? In case of emulator or device, where is it stored? Pls suggest.
Thanks in advance
I got the location of the DB.. It is stored in /data/data/package_name/databases.
We can log in from emulator through adb shell, go to this location, and perform sql operations.
Nice Tutorial.. Its help me lot. Thank you.
iam getting an error in intent . can u pls say me what we have to write in mainActivity.java
Thanx a lot
sir, how to show these data in gridview?
Sir Joe what if instead of textview i will make to be buttons where the data is displayed, can you give me specific code and where can i put it. tnx…
hi.
i am new to the android development please help me while i have been executed and added the names the list is not visible the error i am getting is package not installed
Great site… I have a question for you though, what if I had (3) activities to be writable. (Ex. Wild Animals, Zoo Animals & Domestic Animals). Each activity has to display a list, but the list can’t display the same material throughout, but is stored in the database. How could I acheive this?
It’s very helpfull……….thanx
db.execSQL(“CREATE TABLE “+employeeTable+”
(“+colID+” INTEGER PRIMARY KEY AUTOINCREMENT, “+
colName+” TEXT, “+colAge+” Integer, “+colDept+”
INTEGER NOT NULL ,FOREIGN KEY (“+colDept+”) REFERENCES
“+deptTable+” (“+colDeptID+”));”);
Sir in the above code an error occurs:”string literal is not closed by a double quote”
how can i fix this problem…..pls help!!!
I am writing an app that queries an already created database (read only).
How do I connect to the database via a file that is already created?
All examples I have seen create the database on the fly.
Where does androidsqlite.db locate? in what folder?
Thanks for this example.
android connectivity explain pls
easy way to learn android & tel best web site..
I am beginner to android! i tried this example and dint understand the ListAdapter what is ‘animalList'(data) under view?
you getting errors because something is not right .
Now get up log look at the log and correct the errors
Rather than asking blindliy
sir its a good example in sqlite but can you send any foreign key concept example publish..plz sir help me
tnx my dear friend…..
hi..sir
i am new for andorid..i know very well java..but sir i tired this example..but i run my application the give me error application stop unexptedly please try again on emulator..plz help me..i tired before one week..stil not run sucessfuly my aplication..
Can i view the database in DDMS using your project?
Thanks
shru
tankssssssssssssssss
good one.
wonderful Article…. very helpful to me…
How could I use this in Visual Studio? No java files.
Very useful..
wow,wow,wow,wow
Hi Joe
Thanks for this useful post:)
In all of your CRUD methods above, except insertAnimal method, don’t others need to close SQLitedatabase instance like this? “database.close();”
Thank you!
Dear Joe, Thank you. It is a very nice tutorial.
Dear Joe
Nice post for learners like me. Can you please help me with why I am getting this error? Thanks in advance. All I am trying is to click checkboxes in a list and save them in SQLite. When I am displaying in a toast the selected items it is showing correctly. But when I am trying to show items that are stored in SQLite in toast, it is showing repetitive long list of numbers: Here is the code:
btn5.setOnClickListener(new Button.OnClickListener(){
@Override public void onClick(View v) {
String selected = “”;
int getClicks = lv.getCount();
SparseBooleanArray sparseBooleanArray = lv.getCheckedItemPositions();
for(int i = 0; i < getClicks; i++){
if(sparseBooleanArray.get(i)) {
selected += lv.getItemAtPosition(i).toString() + "\n";
pl.execSQL("INSERT INTO phonelist (MOBILE) VALUES ('"
+ selected + "');");
}
}
getPhoneList();
Toast.makeText(ContactListActivity.this, selected, Toast.LENGTH_LONG).show();
Toast.makeText(ContactListActivity.this, addedPhoneList.toString(), Toast.LENGTH_LONG).show();
}
});
}
private void getPhoneList(){
Cursor c = pl.rawQuery("SELECT * FROM phonelist", null);
if (c != null) {
if (c.moveToFirst()) {
do {
String mobi = c.getString(c
.getColumnIndex("MOBILE"));
addedPhoneList.add(mobi);
} while (c.moveToNext());
}
}
getReadable is used for only read and not edit update or stored
getwritable is used for update purpose.
download accounttracker app from net BANK ACCOUNT TRACKER you can easily find the difference.
Hello Sir We are developing an app to search the dishes in different restaurants that is user specifies the dish name and the dishes that are available in different restaurants will be displayed.To develop this app is it better to use SQLLite database or MySQL database please suggest which to use.