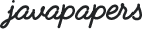
This Servlet tutorial is to take you to the next step in learning servlets. As stated earlier in start of the servlet tutorial series, servlets are primarily meant for web applications. In this let us see how we can read html form data from a URL and process it in a servlet and then send the response back to the client.
As seen earlier in Servlet terminologies, GET and POST methods are used to pass information from a form to a Java Servlet. While using GET method the form data is passed in the url as query parameters. GET method is the default method used. It looks like,
http://localhost:8080/hello?key1=value1&key2=value2
If POST method is used then the form data is passed in the HTTP request message body. It cannot be seen in the URL as seen in GET method. URL length has a limitation and so if we are passing large volume of data we should use POST. Similarly sensitive data like passwords should also be passed using POST method.
we have methods doXYZ(…) in the HTTPServlet class. The method doGet(…) is invoked by the servlet container if the form uses GET method or doPost(…) if the the form uses POST method. In this example we are using GET method.
package com.javapapers.servlet.introduction; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class HelloFormData extends HttpServlet { private static final long serialVersionUID = 1L; public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html"); PrintWriter out = response.getWriter(); String title = "Servlet: Read Form Data"; out.println("<html>" + "<head><title>" + title + "</title></head><body>" + "<h1>" + title + "</h1>" + "<p>Hi " + request.getParameter("name") + "</p>" + "</body></html>"); } }
This web.xml defines the Servlet mapping for the form data servlet.
<web-app xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd" version="2.4"> <display-name>Servlet Form Data Handling</display-name> <servlet> <servlet-name>HelloFormData</servlet-name> <servlet-class>com.javapapers.servlet.introduction.HelloFormData</servlet-class> </servlet> <servlet-mapping> <servlet-name>HelloFormData</servlet-name> <url-pattern>/hello</url-pattern> </servlet-mapping> </web-app>
<!DOCTYPE html> <html> <head> <meta charset="ISO-8859-1"> <title>Servlet Read Form Data</title> </head> <body> <form action="./hello" method="GET"> Enter your Name: <input type="text" name="name"> <input type="submit" value="Submit" /> </form> </body> </html>
In the above HTML page in form tag instead of GET use as POST. Then in the servlet to read the form data add a doPost method as below,
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request, response); }
Download the example Servlet project:Servlet Read Form Data Project Source
Comments are closed for "Servlet Read Form Data".
A very good intro on servlets! I have been following your tutorial for quite sometime now. You have been doing awesome! Please post more java8 related topics.
Thanks Jawahar.
Yes sure, I will continue to write more Java 8 tutorials.
Hi Joe,
Thanks for the article and one request , can you please write some articles on J2EE patterns
Hi Joe,
how to use anchor tag using PrintWriter object to get back to the index.html from “Servlet:Read Form Data…. Hi Joe”
Hi Joe,
How is the url: localhost:8080/Form_Data/ being redirected to index.html.
Shouldn’t index.html be specified in the welcome file list in web.xml.
Thanks
Prabhu
Hi
I have html page in that data , checkboxs are there .
I want read information of date and checkboxs in servlet
How can I get them
plz reply me
good
Hey,
Would this be able to work when hosted on server?