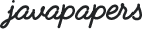
java.lang.Object class is the super base class of all Java classes. Every other Java classes descends from Object. Should we say the God class? Why is that so? This Java article is to discuss around it.
In Mathematics, an axiom is a starting point of reasoning using which other statement can be logically derived. The concept of Object class as super class of all classes in Java looks similar to it.
It is not a explicit requirement forced on the developer. If a class is declared without extending another class then it will implicitly extend Object class. This is taken care of by the JVM. This is not applicable for the interfaces. A Java interface does not extends the Object.
Following could be the reasons for this design decision,
This concept cannot be generalized to all object oriented programming (OOPS) languages. For instance, in C++ there is no such super class of all classes.
Following program shows
package com.javapapers.java; public class SuperClass { public static void main(String... args) { String str = new String("Hi"); Class strClass = str.getClass(); System.out .println("Super class of String: " + strClass.getSuperclass()); Object obj = new Object(); Class objClass = obj.getClass(); System.out .println("Super class of Object: " + objClass.getSuperclass()); Class classClass = objClass.getClass(); System.out.println("Super class of Class: " + classClass.getSuperclass()); } }
Super class of String: class java.lang.Object Super class of Object: null Super class of Class: class java.lang.Object
Piyush Raj sent me a question, “why Object is superclass of all the classes in Java”, hope I have answered it Piyush and thanks.
Comments are closed for "Why Object is Super Class in Java?".
Thank you very much sir
looking forward the see some more posts related to java and thanks a lot for the sample code , very easy to understand and store.
Thank you Joe.
Very nice :)
Great Joe..I have two more questions:
1) Why is clone method protected in Java?
2) Why is finalize method protected in Java?
Thank You very much for the valuable information sir
Thank you very much for the information.
But i got the following output while i am running above program on my machine.
Super class of String : class java.lang.String
Super class of Object : class java.lang.Object
Super class of Class : class java.lang.Class
Sir kindly please explain me the reason for this different output.
Thank You
We are really en’joe’ing here.Please can you Post on
Extending Thread vs Implementing Runnable??
Many Thanks..
This is amazing, I always love your articles.
Kishore, that’s highly unlikely. What is the Java environment you have? JDK version etc.
“By having the Object as the super class of all Java classes, without knowing the type we can pass around objects using the Object declaration.”
Sir can you kindly explain some what more clearly the above mentioned statement plz.
sir the “Tutorials Menu” is not working.
when i click on java then complete tutorials is not coming except a single page with latest tutorials about it.
In the above example we have create string object, “Obect ” object, but sir why we did not create class object using new. Please give me answer sir i am very confused in this context.
Thanks.. This is very helpful :)
Because Java doesn’t allow you to create instance of class Class.
See Class.java
/*
* Constructor. Only the Java Virtual Machine creates Class
* objects.
*/
private Class() {}
Good one Joe. More elaborate explanation on the object part will be much more helpful. Thanks.
Jitu,
Thanks for the comment. Yes that’s right.
Presently I am working to debug/fix a performance issue with the website. So I have temporarily disabled it. Surely, I will enable it back once this is done. It may take a week to do.
Appreciate writing it to me about it. It helps me understand that it is important to the visitors.
Good Work Joe.. keep it up.
If an interface does not extends Object class then why the interface references shows toString(), hashCode() and other Object’s method.
Is there any abstract here maintained by Java? Interface can not extend a class for sure. But is Object class an exception for this?
Hi joe,
This is very helpful tutorial.
But can you please post on is java a purely object oriented programming language or not.
Thanks in advance
“purely object oriented programming language” is a very subjective matter. The whole explanation that Joe has given can be simply summarized as – Java is an Object oriented language where every single thing is an object (or at least intended to be). So every class has to extend an Object type.
Also when you are programming in Java you will always think in terms of objects, their attribute\properties and what can these objects do. So in that way Java is as Object oriented as you can get.
At the same time there are many places where Java deviates from real world object oriented concepts. Inheritance is a one such concept. In Java you just have Classical Inheritance that is one type (class) inherits from another type. Where as in real world the most visible inheritance is in the form of one object inherits from another objects which is not supported by Java. JavaScript supports this concepts.
So the answer to your question is Java a purely Object Oriented programming language… well its not as easy a question to answer….
Great Explanation :)
Hello Sir
Object Class is an super Class of Every JAVA Classes .
But If I want to See How Object Class is an Super Class Of Particular class ? How to See it Please Give me as Solution
Thank you
extend Thread gives you scope to extends only one class, but by implementing runnable interface there is a scope to extend any number of classes and interface
same like multiple inheritance.. That is one of the advantage of interface
If u r application is having only one thread then go for Extending Thread class
& if ur application is having multiple threads then use implements Runnable interface
I have never seen this type of explanation, specially the code you put here to reveal the concept.
Kudos to you !!!
Really very nice Explanation Why java.lang.Object is Super Class of all Classes.You are talented..JOE
Answer to Shaesh Question:
“If an interface has no direct superinterfaces, then the interface implicitly declares a public abstract member method m with signature s, return type r, and throws clause t corresponding to each public instance method m with signature s, return type r, and throws clause t declared in Object, unless a method with the same signature, same return type, and a compatible throws clause is explicitly declared by the interface.” As perJava Language Specification
why Object is superclass of all the classes in java .can u explain sir
Thank you for the above post. You explained “why Object is super class” but could you explain ” How Object class is super class of all classes? “. To be more precise is there any code inside JRE which is the implementation of JVM that has logic which makes the Object class as parent class to all the other classes?
Thanks in advance.
sir please post the actual code for composition & aggregation
Why String declared as immutable object?