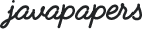
This temporal adjuster topic is very interesting. I am going to post a series of example tutorials for temporal adjuster. We have been long struggling with date and time operations in Java. With the arrival of Java 8, the date and time API is really wonderful and makes all the operations simple and easy.
In a previous tutorial we saw about what is temporal adjuster and how to use it and followed by an example to write a custom temporal adjuster. In this tutorial let use see how to write a temporal adjuster to find the next leap year day.
package com.javapapers.java8.dateandtime; import java.time.LocalDate; import java.time.temporal.Temporal; import java.time.temporal.TemporalAdjuster; public class NextLeapYearDayTemporalAdjuster implements TemporalAdjuster { @Override public Temporal adjustInto(Temporal temporalAdjusterInput) { LocalDate temporalAdjusterDate = LocalDate.from(temporalAdjusterInput); int year = temporalAdjusterDate.getYear(); for (; !((year % 400 == 0) || ((year % 4 == 0) && (year % 100 != 0))); year++) ; LocalDate leapYearDate = LocalDate.of(year, 2, 29); return leapYearDate; } public static void main(String... strings) { LocalDate currentDate = LocalDate.now(); NextLeapYearDayTemporalAdjuster leapYearAdjuster = new NextLeapYearDayTemporalAdjuster(); LocalDate leapYearDay = currentDate.with(leapYearAdjuster); System.out.println("Next Leap Year Day " + leapYearDay); } }
This temporal adjuster, gets the year for the input year and checks for the next leap year. Then constructs the date for that year and returns the adjusted date.
Next Leap Year Day 2016-02-29