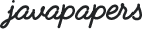
This Java NIO tutorial is to learn about how to access file attributes and if possible modify it. java.nio.file.attribute
package contains classes that helps to get and set file attributes. Before Java SE 7 we did not have direct support to access the large array file attributes. Now the NIO API is advanced, it has abstracted the underlying OS and its file system by providing seamless less access to the attributes and a way of manipulating them. This tutorial is part of the Java NIO tutorial series.
Again the NIO Files util class comes into play. We can use it to get an instance of BasicFileAttributes by passing its class as argument using the method readAttributes. Once we get an instance of BasicFileAttributes then its just about accessing the attributes using its get accessor methods. We can modify some attributes like lastModifiedTime. The below example code demonstrates accessing the basic file attributes. It also showcases how we can modify the last modified time for a file.
//Path path = Paths.get("temp.txt"); //nioBasicFileAttributes(path); public static void nioBasicFileAttributes(Path path) throws IOException { BasicFileAttributes basicFileAttributes = Files.readAttributes(path, BasicFileAttributes.class); // Print basic file attributes System.out.println("Creation Time: " + basicFileAttributes.creationTime()); System.out.println("Last Access Time: " + basicFileAttributes.lastAccessTime()); System.out.println("Last Modified Time: " + basicFileAttributes.lastModifiedTime()); System.out.println("Size: " + basicFileAttributes.size()); System.out.println("Is Regular file: " + basicFileAttributes.isRegularFile()); System.out .println("Is Directory: " + basicFileAttributes.isDirectory()); System.out.println("Is Symbolic Link: " + basicFileAttributes.isSymbolicLink()); System.out.println("Other: " + basicFileAttributes.isOther()); // modify the lastmodifiedtime FileTime newModifiedTime = FileTime.fromMillis(basicFileAttributes .lastModifiedTime().toMillis() + 60000); Files.setLastModifiedTime(path, newModifiedTime); // check if the lastmodifiedtime is changed System.out.println("After Changing lastModifiedTime, "); System.out.println("Creation Time: " + basicFileAttributes.creationTime()); System.out.println("Last Access Time: " + basicFileAttributes.lastAccessTime()); System.out.println("Last Moodified Time: " + basicFileAttributes.lastModifiedTime()); }
Creation Time: 2015-07-21T05:02:46.120261Z Last Access Time: 2015-07-21T05:02:46.120261Z Last Modified Time: 2015-07-21T15:22:41.408Z Size: 4 Is Regular file: true Is Directory: false Is Symbolic Link: false Other: false After Changing lastModifiedTime, Creation Time: 2015-07-21T05:02:46.120261Z Last Access Time: 2015-07-21T05:02:46.120261Z Last Moodified Time: 2015-07-21T15:22:41.408Z
Let us see how we can get and set the owner of a file using Java NIO. We can use the same NIO Files util class and use method getFileAttributeView by passing argument FileOwnerAttributeView class. Then we get the UserPrincipal using which we can get and set the file owner name. Setting will throw exception if the passed name is not present in the user principle or if access is denied to set the owner name. Following is the example code demonstrating it.
//Path path = Paths.get("temp.txt"); //nioBasicFileAttributes(path); public static void nioFileOwnerAttributeView(Path path) throws IOException { FileOwnerAttributeView fileOwnerAttributeView = Files .getFileAttributeView(path, FileOwnerAttributeView.class); UserPrincipal userPrincipal = fileOwnerAttributeView.getOwner(); System.out.println("File Owner: " + userPrincipal.getName()); FileSystem fileSystem = FileSystems.getDefault(); UserPrincipalLookupService userPLS = fileSystem .getUserPrincipalLookupService(); // java.nio.file.attribute.UserPrincipalNotFoundException possible here // if the user name passed is not present // java.nio.file.AccessDeniedException possible if not enough rights UserPrincipal newUserPrincipal = userPLS .lookupPrincipalByName("accountgroup\\username"); fileOwnerAttributeView.setOwner(newUserPrincipal); UserPrincipal changedUserPrincipal = fileOwnerAttributeView.getOwner(); System.out.println("Changed File Owner: " + changedUserPrincipal); }
Let us see how we can get space related information of the underlying disk. We can use NIO classes FileSystems and FileStore to get the space information.
public static void nioFileStoreSpace() throws IOException { // Loop through the set of FileStores for the default FileSystem for (FileStore fileStore : FileSystems.getDefault().getFileStores()) { System.out.println("Name: " + fileStore.name()); System.out.println("Type: " + fileStore.type()); System.out.println("Total Space (MB): " + fileStore.getTotalSpace() / 1024 / 1024); System.out.println("Unallocated Space (MB): " + fileStore.getUnallocatedSpace() / 1024 / 1024); System.out.println("Usable Space (MB): " + fileStore.getUsableSpace() / 1024 / 1024); } }
Name: PROG Type: NTFS Total Space (MB): 93889 Unallocated Space (MB): 38873 Usable Space (MB): 38873 Name: INF Type: NTFS Total Space (MB): 20479 Unallocated Space (MB): 9280 Usable Space (MB): 9280 Name: System Reserved Type: NTFS Total Space (MB): 99 Unallocated Space (MB): 71 Usable Space (MB): 71
NIO provides API to get and set an user defined attribute to a file. This will be hand in many real time situations. We can use the NIO API UserDefinedFileAttributeView and Files class to write and read a custom attribute to a file.
// Path path = Paths.get("temp.txt"); // nioUserDefinedFileAttributeView(path); public static void nioUserDefinedFileAttributeView(Path path) throws IOException { if (File.separatorChar != '\\') { System.out.println("This is Windows specific and so exit!"); return; } UserDefinedFileAttributeView userDefinedFAView = Files .getFileAttributeView(path, UserDefinedFileAttributeView.class); ListattributeList = userDefinedFAView.list(); System.out.println("User Defined Attribute List Size Before Adding: " + attributeList.size()); // set user define attribute String attributeName = "foo"; String attributeValue = "bar"; userDefinedFAView.write(attributeName, Charset.defaultCharset().encode(attributeValue)); attributeList = userDefinedFAView.list(); if (attributeList.size() > 0) { for (String attName : attributeList) { ByteBuffer attValue = ByteBuffer.allocate(userDefinedFAView .size(attName)); userDefinedFAView.read(attName, attValue); attValue.flip(); System.out.println("User Defined Attribute Name: " + attName); System.out.println("User Defined Attribute Value: " + Charset.defaultCharset().decode(attValue).toString()); } } else { System.out .println("User define attribute count should be at least 1" + " as we have set an attribute just now!"); } userDefinedFAView.delete(attributeName); // And list the attributes again to check they're back to 0 attributeList = userDefinedFAView.list(); System.out.println("User Defined Attribute List Size After Deleting: " + attributeList.size()); }
User Defined Attribute List Size Before Adding: 0 User Defined Attribute Name: foo User Defined Attribute Value: bar User Defined Attribute List Size After Deleting: 0
Comments are closed for "File Attributes using Java NIO".
[…] File Attributes using Java NIO […]