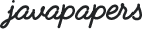
This Java tutorial is to demonstrate the Dropbox Java API which can be used to manage the files in a Dropbox account via Java code. This is fairly a simple Java API and easy to use.
Do we need an introduction for Dropbox? If you are thinking about what is Dropbox, its a really popular application to store and edit documents from computer, mobile and tablet. Dropbox is one of the successful YCombinator start-ups and now worth in billions. We get 2GB free when we signup and additional storage can be purchased. This can be effectively used as a backup space. Dropbox innovated and set this domain on fire. Now we have Google Drive, Microsoft OneDrive and so many players into this business.
Dropbox provides API for almost all popular programming platforms. API provides programmatic way to read and write to Dropbox. Java Dropbox API can be used to interact with Dropbox from our Java applications.
Following example illustrates how to write a Java application to upload a file to Dropbox, create folder, check the size and other information.
We need to create an application in Dropbox. Login and go to the Dropbox Apps console.
We need the Dropbox App secret and Dropbox App key to authorize with the Dropbox API. Grab them as shown below.
As a prerequisite, we should create a Dropbox App and get the Drobox App Secret, App Key as detailed above. We should instantiate DbxAppInfo
by passing the Dropbox App Secret and App key. Once instantiated, we can authorize using the web request using DbxWebAuthNoRedirect
. We can get the authorize url and go to that URL and then allow access and get the auth code.
Use the above access code in Java application to gain access to the Dropbox API.
public DbxClient authDropbox(String dropBoxAppKey, String dropBoxAppSecret) throws IOException, DbxException { DbxAppInfo dbxAppInfo = new DbxAppInfo(dropBoxAppKey, dropBoxAppSecret); DbxRequestConfig dbxRequestConfig = new DbxRequestConfig( "JavaDropboxTutorial/1.0", Locale.getDefault().toString()); DbxWebAuthNoRedirect dbxWebAuthNoRedirect = new DbxWebAuthNoRedirect( dbxRequestConfig, dbxAppInfo); String authorizeUrl = dbxWebAuthNoRedirect.start(); System.out.println("1. Authorize: Go to URL and click Allow : " + authorizeUrl); System.out .println("2. Auth Code: Copy authorization code and input here "); String dropboxAuthCode = new BufferedReader(new InputStreamReader( System.in)).readLine().trim(); DbxAuthFinish authFinish = dbxWebAuthNoRedirect.finish(dropboxAuthCode); String authAccessToken = authFinish.accessToken; dbxClient = new DbxClient(dbxRequestConfig, authAccessToken); System.out.println("Dropbox Account Name: " + dbxClient.getAccountInfo().displayName); return dbxClient; }
/* returns Dropbox size in GB */ public long getDropboxSize() throws DbxException { long dropboxSize = 0; DbxAccountInfo dbxAccountInfo = dbxClient.getAccountInfo(); // in GB :) dropboxSize = dbxAccountInfo.quota.total / 1024 / 1024 / 1024; return dropboxSize; }
public void uploadToDropbox(String fileName) throws DbxException, IOException { File inputFile = new File(fileName); FileInputStream fis = new FileInputStream(inputFile); try { DbxEntry.File uploadedFile = dbxClient.uploadFile("/" + fileName, DbxWriteMode.add(), inputFile.length(), fis); String sharedUrl = dbxClient.createShareableUrl("/" + fileName); System.out.println("Uploaded: " + uploadedFile.toString() + " URL " + sharedUrl); } finally { fis.close(); } }
File uploaded to Dropbox successfully.
public void createFolder(String folderName) throws DbxException { dbxClient.createFolder("/" + folderName); }
public void listDropboxFolders(String folderPath) throws DbxException { DbxEntry.WithChildren listing = dbxClient .getMetadataWithChildren(folderPath); System.out.println("Files List:"); for (DbxEntry child : listing.children) { System.out.println(" " + child.name + ": " + child.toString()); } }
public void downloadFromDropbox(String fileName) throws DbxException, IOException { FileOutputStream outputStream = new FileOutputStream(fileName); try { DbxEntry.File downloadedFile = dbxClient.getFile("/" + fileName, null, outputStream); System.out.println("Metadata: " + downloadedFile.toString()); } finally { outputStream.close(); } }
package com.javapapers.java.dropbox; import java.io.BufferedReader; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStreamReader; import java.util.Locale; import com.dropbox.core.DbxAccountInfo; import com.dropbox.core.DbxAppInfo; import com.dropbox.core.DbxAuthFinish; import com.dropbox.core.DbxClient; import com.dropbox.core.DbxEntry; import com.dropbox.core.DbxException; import com.dropbox.core.DbxRequestConfig; import com.dropbox.core.DbxWebAuthNoRedirect; import com.dropbox.core.DbxWriteMode; public class JavaDropbox { private static final String DROP_BOX_APP_KEY = "bxsicd65ljq0ymh"; private static final String DROP_BOX_APP_SECRET = "3t1lqvq1amaehb1"; DbxClient dbxClient; public DbxClient authDropbox(String dropBoxAppKey, String dropBoxAppSecret) throws IOException, DbxException { DbxAppInfo dbxAppInfo = new DbxAppInfo(dropBoxAppKey, dropBoxAppSecret); DbxRequestConfig dbxRequestConfig = new DbxRequestConfig( "JavaDropboxTutorial/1.0", Locale.getDefault().toString()); DbxWebAuthNoRedirect dbxWebAuthNoRedirect = new DbxWebAuthNoRedirect( dbxRequestConfig, dbxAppInfo); String authorizeUrl = dbxWebAuthNoRedirect.start(); System.out.println("1. Authorize: Go to URL and click Allow : " + authorizeUrl); System.out .println("2. Auth Code: Copy authorization code and input here "); String dropboxAuthCode = new BufferedReader(new InputStreamReader( System.in)).readLine().trim(); DbxAuthFinish authFinish = dbxWebAuthNoRedirect.finish(dropboxAuthCode); String authAccessToken = authFinish.accessToken; dbxClient = new DbxClient(dbxRequestConfig, authAccessToken); System.out.println("Dropbox Account Name: " + dbxClient.getAccountInfo().displayName); return dbxClient; } /* returns Dropbox size in GB */ public long getDropboxSize() throws DbxException { long dropboxSize = 0; DbxAccountInfo dbxAccountInfo = dbxClient.getAccountInfo(); // in GB :) dropboxSize = dbxAccountInfo.quota.total / 1024 / 1024 / 1024; return dropboxSize; } public void uploadToDropbox(String fileName) throws DbxException, IOException { File inputFile = new File(fileName); FileInputStream fis = new FileInputStream(inputFile); try { DbxEntry.File uploadedFile = dbxClient.uploadFile("/" + fileName, DbxWriteMode.add(), inputFile.length(), fis); String sharedUrl = dbxClient.createShareableUrl("/" + fileName); System.out.println("Uploaded: " + uploadedFile.toString() + " URL " + sharedUrl); } finally { fis.close(); } } public void createFolder(String folderName) throws DbxException { dbxClient.createFolder("/" + folderName); } public void listDropboxFolders(String folderPath) throws DbxException { DbxEntry.WithChildren listing = dbxClient .getMetadataWithChildren(folderPath); System.out.println("Files List:"); for (DbxEntry child : listing.children) { System.out.println(" " + child.name + ": " + child.toString()); } } public void downloadFromDropbox(String fileName) throws DbxException, IOException { FileOutputStream outputStream = new FileOutputStream(fileName); try { DbxEntry.File downloadedFile = dbxClient.getFile("/" + fileName, null, outputStream); System.out.println("Metadata: " + downloadedFile.toString()); } finally { outputStream.close(); } } public static void main(String[] args) throws IOException, DbxException { JavaDropbox javaDropbox = new JavaDropbox(); javaDropbox.authDropbox(DROP_BOX_APP_KEY, DROP_BOX_APP_SECRET); System.out.println("Dropbox Size: " + javaDropbox.getDropboxSize() + " GB"); javaDropbox.uploadToDropbox("happy.png"); javaDropbox.createFolder("tutorial"); javaDropbox.listDropboxFolders("/"); javaDropbox.downloadFromDropbox("happy.png"); } }
1. Authorize: Go to URL and click Allow : https://www.dropbox.com/1/oauth2/authorize?locale=en_IN&client_id=bxsicd65ljq0ymh&response_type=code 2. Auth Code: Copy authorization code and input here _-heFn6Qtl8AAAAAAAALAEtTxFPQPHNI-T0PufKYbv0 Dropbox Account Name: Joseph Kulandai R Dropbox Size: 2 GB Uploaded: File("/happy (4).png", iconName="page_white_picture", mightHaveThumbnail=true, numBytes=24670, humanSize="24.1 KB", lastModified="2014/12/07 13:02:12 UTC", clientMtime="2014/12/07 13:02:12 UTC", rev="82e10806e") URL https://www.dropbox.com/s/nq6ebu8mqbfr6pz/happy.png?dl=0 Files List: happy (1).png: File("/happy (1).png", iconName="page_white_picture", mightHaveThumbnail=true, numBytes=24670, humanSize="24.1 KB", lastModified="2014/12/07 12:40:07 UTC", clientMtime="2014/12/07 12:40:07 UTC", rev="42e10806e") happy (2).png: File("/happy (2).png", iconName="page_white_picture", mightHaveThumbnail=true, numBytes=24670, humanSize="24.1 KB", lastModified="2014/12/07 12:58:50 UTC", clientMtime="2014/12/07 12:58:50 UTC", rev="52e10806e") happy (3).png: File("/happy (3).png", iconName="page_white_picture", mightHaveThumbnail=true, numBytes=24670, humanSize="24.1 KB", lastModified="2014/12/07 13:00:23 UTC", clientMtime="2014/12/07 13:00:24 UTC", rev="62e10806e") happy (4).png: File("/happy (4).png", iconName="page_white_picture", mightHaveThumbnail=true, numBytes=24670, humanSize="24.1 KB", lastModified="2014/12/07 13:02:12 UTC", clientMtime="2014/12/07 13:02:12 UTC", rev="82e10806e") happy.png: File("/happy.png", iconName="page_white_picture", mightHaveThumbnail=true, numBytes=24670, humanSize="24.1 KB", lastModified="2014/12/07 12:07:13 UTC", clientMtime="2014/12/07 12:07:14 UTC", rev="22e10806e") tutorial: Folder("/tutorial", iconName="folder", mightHaveThumbnail=false) Metadata: File("/happy.png", iconName="page_white_picture", mightHaveThumbnail=true, numBytes=24670, humanSize="24.1 KB", lastModified="2014/12/07 12:07:13 UTC", clientMtime="2014/12/07 12:07:14 UTC", rev="22e10806e")
Comments are closed for "Dropbox Java API Tutorial".
How to run the code from eclipse plz explain..
Exception in thread “main” java.io.FileNotFoundException: happy.png (The system cannot find the file specified)
at java.io.FileInputStream.open(Native Method)
at java.io.FileInputStream.(Unknown Source)
at com.javapapers.java.dropbox.JavaDropbox.uploadToDropbox(JavaDropbox.java:62)
at com.javapapers.java.dropbox.JavaDropbox.main(JavaDropbox.java:104)
How to handle this error!!
Program not able to get image,
make image available to program or change the image path
Hi!
Can same java API be used in Android?
Thanks.
Where should the file to be uploaded saved? I am getting FileNotFound Exception.
Thank You
I would like to run this as part of an automated process. Is there a way to get the authorization code without prompting the user?
solution to Exception in thread “main” java.io.FileNotFoundException: happy.png (The system cannot find the file specified)
at java.io.FileInputStream.open(Native Method)
at java.io.FileInputStream.(Unknown Source)
at com.javapapers.java.dropbox.JavaDropbox.uploadToDropbox(JavaDropbox.java:62)
at com.javapapers.java.dropbox.JavaDropbox.main(JavaDropbox.java:104)
>> add image to project itself instead of adding it in “src” folder in project.
Use JFile Chooser (Worked for me…….)
public void uploadToDropbox() throws DbxException,
IOException {
File inputFile;
JFileChooser filechoose=new JFileChooser();
filechoose.setFileSelectionMode(JFileChooser.FILES_ONLY);
filechoose.showOpenDialog(null);
inputFile=filechoose.getSelectedFile();
s=inputFile.getName();
//File inputFile = new File(fileName);
FileInputStream fis = new FileInputStream(inputFile);
try {
DbxEntry.File uploadedFile = dbxClient.uploadFile(“/” + inputFile.getName(),
DbxWriteMode.add(), inputFile.length(), fis);
String sharedUrl = dbxClient.createShareableUrl(“/” + inputFile.getName());
System.out.println(“Uploaded: ” + uploadedFile.toString() + ” URL ”
+ sharedUrl);
} finally {
fis.close();
}
}
thanks rpatel !
Hi Sir how to implement dropbox Dbxwebauth in servlet or jsp
Please any one give me solution above program can be cover in jsp and servlet or rest api
i am able to do in jsp using Access_token but only i can see file mine folder i want any any one one integrate my apps then can see them files