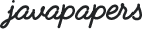
This Hiberate tutorial is part of the Hiberate introduction series. We will see how to configure the Hiberate options and instantiate SessionFactory.
Hibernate provides different options to create a SessionFactory instance; SessionFactory
is used for creating multiple lightweight instances of Session object, which in turn enables database operations. We need not worry about the performance side of creating/destroying those Hibernate Session
instances; Because they’re lightweight components.
On the other side the process of creating instance for Hibernate SessionFactory is an expensive operation. So we need to exercise caution while instantiating Hibernate SessionFactory
. We can choose to create the instance through a singleton design pattern.
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- Database connection settings --> <property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property> <property name="hibernate.connection.url">jdbc:mysql://192.168.1.102:3306/javapapers</property> <property name="hibernate.connection.username">root</property> <property name="hibernate.connection.password">root</property> <!-- SQL dialect --> <property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property> <!-- Specify session context --> <property name="hibernate.current_session_context_class">org.hibernate.context.internal.ThreadLocalSessionContext</property> </session-factory> </hibernate-configuration>
Similar to hibernate.cfg.xml, hibernate.properties file should defined for all of these mandatory entries. hibernate.properties file should be located in the application classpath.
hibernate.connection.driver_class = com.mysql.jdbc.Driver hibernate.connection.url = jdbc:mysql://localhost:3306/javapapers hibernate.connection.username = root hibernate.connection.password = root hibernate.dialect = org.hibernate.dialect.MySQLDialect hibernate.current_session_context_class=thread
With hibernate.properties the will project look like:
Create an example Hibernate application and you need to setup the development environment. Follow the linked tutorial to setup the project and put the following Java class file and run it.
package com.javapapers; import java.io.FileNotFoundException; import java.io.IOException; import java.util.Properties; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.boot.registry.StandardServiceRegistryBuilder; import org.hibernate.cfg.Configuration; import org.hibernate.service.ServiceRegistry; public class App { public static void main(String[] args) throws FileNotFoundException, IOException{ configureUsingHibernatePropertiesFile(); } public static void configureUsingHibernateConfigXMLFile(){ // Create configuration instance Configuration configuration = new Configuration(); // Pass hibernate configuration file configuration.configure("hibernate.cfg.xml"); // Since version 4.x, service registry is being used ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder(). applySettings(configuration.getProperties()).build(); // Create session factory instance SessionFactory factory = configuration.buildSessionFactory(serviceRegistry); // Get current session Session session = factory.getCurrentSession(); // Begin transaction session.getTransaction().begin(); // Print out all required information System.out.println("Session Is Opened :: "+session.isOpen()); System.out.println("Session Is Connected :: "+session.isConnected()); // Commit transaction session.getTransaction().commit(); System.exit(0); } public static void configureUsingHibernatePropertiesFile() throws IOException{ // Create configuration instance Configuration configuration = new Configuration(); // Create properties file Properties properties = new Properties(); properties.load(Thread.currentThread().getContextClassLoader().getResourceAsStream("hibernate.properties")); // Pass hibernate properties file configuration.setProperties(properties); // Since version 4.x, service registry is being used ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder(). applySettings(configuration.getProperties()).build(); // Create session factory instance SessionFactory factory = configuration.buildSessionFactory(serviceRegistry); // Get current session Session session = factory.getCurrentSession(); // Begin transaction session.getTransaction().begin(); // Print out all required information System.out.println("Session Is Opened :: "+session.isOpen()); System.out.println("Session Is Connected :: "+session.isConnected()); // Commit transaction session.getTransaction().commit(); System.exit(0); } }
Comments are closed for "Hibernate Configuration And SessionFactory Instantiation".
[…] Hibernation Configuration Options and SessionFactory setup. […]
Hi Joe,
I need a small help from you. Can you please explain about the ecache in hibernate how it works. Consider me as i don’t know anything about the ecache.
I went through may site and i still not comfortable with the way they have presented(Sorry to say this on the others site. They have presented good but it little confusing me).
Thanks,
Nagendra.
Small correcting its Ehcache.
your blog is very cool, thank you very much!
Can you please explain what is the use of declaring ThreadLocalSessionContext in configuration.
org.hibernate.context.internal.ThreadLocalSessionContext