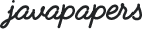
“An object kept as a reminder of a person or event” is what Wikipedia says for the word “memento”. I cannot write about memento design pattern without mentioning Christopher Nolan’s movie Memento. The hero of Memento has a type of amnesia and he tries to backtrack events to trace the killer.
Memento movie and memento design pattern are synonymous. Memento design pattern helps to restore an object’s state to it previous state. Should we call it Ctrl+z design pattern instead?
Undo or backspace or ctrl+z is one of the most used operation in an editor. Memento design pattern is used to implement the undo operation. This is done by saving the current state of the object as it changes state. One important point to not in implementing memento design pattern is, the encapsulation of the object should not be compromised.
In implementing memento pattern, we have two objects of interest,
The originator will store the state information in the memento object and retrieve old state information when it needs to back track. The memento just stores what the originator gives to it. Memento object is unreachable for other objects in the application.
package com.javapapers.designpattern.memento; public class Memento { private String state; public Memento(String state){ this.state = state; } public String getState() { return state; } }
package com.javapapers.designpattern.memento; public class Originator { //this String is just for example //in real world application this //will be a java class - the object //for which the state to be stored private String state; public void setState(String state) { this.state = state; } public String getState() { return state; } public Memento createMemento() { return new Memento(state); } public void setMemento(Memento memento) { state = memento.getState(); } }
package com.javapapers.designpattern.memento; import java.util.ArrayList; import java.util.List; public class Caretaker { private ListstatesList = new ArrayList (); public void addMemento(Memento m) { statesList.add(m); } public Memento getMemento(int index) { return statesList.get(index); } }
package com.javapapers.designpattern.memento; public class MementoClient { public static void main(String[] args) { Originator originator = new Originator(); originator.setState("Lion"); Memento memento = originator.createMemento(); Caretaker caretaker = new Caretaker(); caretaker.addMemento(memento); originator.setState("Tiger"); originator.setState("Horse"); memento = originator.createMemento(); caretaker.addMemento(memento); originator.setState("Elephant"); System.out .println("Originator Current State: " + originator.getState()); System.out.println("Originator restoring to previous state..."); memento = caretaker.getMemento(1); originator.setMemento(memento); System.out .println("Originator Current State: " + originator.getState()); System.out.println("Again restoring to previous state..."); memento = caretaker.getMemento(0); originator.setMemento(memento); System.out .println("Originator Current State: " + originator.getState()); } }
Originator Current State: Elephant
Originator restoring to previous state…
Originator Current State: Horse
Again restoring to previous state…
Originator Current State: Lion
Note:
From JDK API, StateHolder interface provides a contract for implementing memento design pattern.
Comments are closed for "Memento Design Pattern".
Originator object is unreachable for other objects in the application.? You meant “Memento object is unreacheable”
Vishal, thanks a lot. I have updated the article.
You are amazingly quick!
Thanks Joe for the wonderful work you are doing in educating java people.
Simple code explanations easily drives the concept home.
Thank you once again, please keep going.
good effort, thanks
Welcome Prakash, lot more to come. Keep reading.
Appreciate your efforts!!
Wonderful explanation, Your each and every article is very helpful to me. Even i know about the topic you are writing, i read it and i learned something new. Good job joy keep teaching us.
Thanks
Well done!
Thanks for giving an introduction to Memento pattern.
Whats advantage does this pattern provide, over storing the State entity of the Originator in a ArrayList within the Originator?
Hi Joe,
Nice explanation. We are using undo operation in our project and now only I came to know its a design pattern. Thankz.. :) :) LOL :)
Hi Joe,
Thanks for your interesting articles. Here, I don’t understand how Momento is unreachable for the rest of the objects in the application. Momento is public class, it’s object can be created anywhere.
Memento class can be public and no harm in reusing that class to create instances. If that class suits to create a memento for other classes let them reuse it. In wikipedia, there is an example, showing it as an inner class of Originator and that is not required. But what should be taken care is, once a memento is created, no others including the caretaker should not be allowed to modify the memento instance.
Memento instance is created by an originator instance and a caretaker instance stores that memento, thats it.
So we should not have the public-setter method in the memento and I updated it now, thanks.
Look at this example,
Employee { String empid, int emp no, float sal}
Employee – is the originator and all those three fields are state that should be stored. So how do you want to do this now without a caretaker?
Thanks Manni.
hello Joe,
i came across your blog before one year ago. But that time i didn’t read your blog carefully. May be due to my laziness or just because of there are too many blogs or sites available and it’s very difficult to read each one. That’s why i read only those topics in which i am confused. Abstraction is always confusing for me and then i read your article. It’s amazingly awesome. It clears my concept about this and related other terms. From that day i started to read your each article carefully and i always found something new and interesting even within the comments. I always wait for your next article.
Many many thanks for this precious blog. Keep it up.
Thanks Neeraj.
I put immense effort behind every article and enjoy it so much. Internet has become so huge, there are are thousands of blogs coming up everyday. One of my goal is to create that difference in each article I write. So nice that people like you read every line of the article and pick that difference out. I feel very happy and get a mission accomplished feeling when I hear this. Thanks.
good work…keep it up…. thanks
Hey Joe.
kindly consider me as one of your greatest fans if not the great one.Ive been reading your articles for a long time and today I finally found some time to appreciate your art of explaination.Every single article that you’ve written on java is dealt with utmost love and passion which is clearly evident from the examples you cite and an introductry pic which bridges the gap between java and the real world.
Consider this as a request which has been made by couple of my fellow readers and deligated by me (they made me a scape goat) regarding your examples.Most of your java examples begins directly by explaining sub-classes, pojo’s which are being consumed by the main class/client.This sometimes deviates the readers from getting the big picture that the code technically wants to show as plenty of readers or beginners ignore understanding the uml’s.So what I may suggest is that instead of explaining the auxillaries of the client at first place, you might consider explaining the main class first like what all the fields do and why are they required.
Boy I dont know imho how the hell i got nerves to write such a huge essay but this was pending in the jms topic/queue for a very very very long time :-)
Does’nt matter what you do i’ll be reading your articles like a child reads a comics for ever.You are the man !!!!
btw m working in your city-chennai.my first time here!!!
Wah man, thanks a lot for spending your time to write this. When you express like this, I become dumbfounded. Thank you so much for fueling me, as there is long way to go.
Do not keep the messages in jms queue for long, sometimes it might get expired :-)
I agree with your comment on the order of explanation, sure I will keep that in mind going forward.
It is highly important to express these kind of opinions, as it will help you and as well as many others (some might by shy to talk). No matter what you feel about your comment as small or big, pass it on to me, it will surely help JavaPapers.
Thanks a lot, keep reading and talking.
Good to know that you have landed in Chennai. Lot of place out there to enjoy. Visit all the malls and have fun.
Why are you specifying the CareTaker’s stateList property type to ArrayList?
Why not use the interface (List)? That way, in the future if you choose, you can update the stateList to be a LinkedList and traverse your ‘Momento’ Objects one by one…
Dan, you got me! Article updated, thanks.
Hi Joe,
I like your articles very much. whenever i read the concepts (even if its already known to me) i always find something new in your articles.. Thank you very much for putting the effort …
I have one question .. Is it really necessary to have the control of going back to the previous state to be there in MementoClient class or It can be present in the class CareTaker ?. .
Can we have some method present in Caretaker to go back to previous state probably with a counter variable maintaining the current state.
Forgive me if its a dump question.
Thanks,
Krishna
If we have a very huge Object as a Memento, am i right in saying that we will need to do deep cloning for that Memento to store its state in List ?
Nice article. You are really passionate of writing technical stuff. Thanks much!
Nice as always.. Thank you Joe…
Hi,
Thanks for a simple explanation.
Can you please list out the difference between State pattern and Memento pattern.
thanks
Is this design pattern(Memento) useful for frequent “undo” operation,ie if we do frequent “undo” operation then a lot of Memento object is created and might cause problem in heap memory.
Please suggest ….