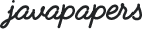
Couple of weeks back, I wrote an article to introduce JVM server and client mode and discussed about how they differ performance wise. I just thought of running some programs and capture performance metrics on these two modes and show case it to you.
As I have explained in my previous article, JVM server mode will give significant performance increase on long running java applications where there is a scope for larger performance optimizations.
Just for experiment sake, I intended to capture performance metrics on short lived core java programs. Do not try to read much out of these metrics as these two modes does not make much sense for this type of programs. Just did this out of curiosity.
Started this fun exercise with a dummy loop and recorded duration on both server and client mode.
package com.javapapers.corejava; public class DummyLoop { public static void main(String args[]) { int i = Integer.parseInt(args[0]); long start = System.currentTimeMillis(); dummyLoop(i); long end = System.currentTimeMillis(); System.out.println(end -start); } public static void dummyLoop(int i) { for (; i>0; i--) { } } }
So how to read the following table. I ran the program consequetively for five times with the same input first in client mode and then in server mode. Last column shows the difference (performance improvement) of server mode over client mode. Time is measured in milli seconds. I ran the loop for 99999999 iterations.
Run | Client Mode | Server Mode | Difference |
---|---|---|---|
1 | 32 | 15 | 17 |
2 | 31 | 0 | 31 |
3 | 32 | 0 | 32 |
4 | 31 | 0 | 31 |
5 | 31 | 0 | 31 |
By seeing the statistics, I was awed and felt amazing. After the first execution, Server mode runs in no time! Theoretically its impossible. But it says that it has taken negligible amount of time to execute. That means the optimization by JIT compiler at runtime has done its job. This is happening only because its a dumb program. JIT compiler has recognized that there is nothing inside the for loop and have removed it as part of optimization.
It was so easy for the JIT to optimize the for loop as there is nothing inside it in the previous program. I thought of adding a statement inside the for loop and see what happens. But still I wanted to you use a dummy statement, just increment a variable inside the for loop and never use that variable. Ran the loop as the same number of times as above 99999999.
package com.javapapers.corejava; public class CheatJava { public static void main(String args[]){ long start = System.currentTimeMillis(); int a = calculate(); long end = System.currentTimeMillis(); System.out.println("Duration: " + (end - start)); } public static int calculate() { int i = 10; int j = 10; int z = i + j; for(int m = 0 ;m<999999999; m++) i++; return z; } }
Run | Client Mode | Server Mode | Difference |
---|---|---|---|
1 | 450 | 0 | 450 |
2 | 430 | 15 | 415 |
3 | 443 | 0 | 443 |
4 | 468 | 0 | 468 |
5 | 452 | 0 | 452 |
JIT is brilliant enough to optimize it!
I just wanted to run a meaning ful program which has a loop and see how the server mode optimizes it. Following program just sums the first n natural numbers. I ran with input 99999999.
package com.javapapers.corejava; class FirstNNaturalSeries { public static void main(String args[]) { long sum; int n = Integer.parseInt(args[0]); long start = System.currentTimeMillis(); sum = sum(n); long end = System.currentTimeMillis(); System.out.println("Sum: " + sum + ". Duration: "+(end-start)); } public static long sum(int n) { long sum = 0; for (int i = 1; i <= n; i++) { sum = sum + i; } return sum; } }
Run | Client Mode | Server Mode | Difference |
---|---|---|---|
1 | 125 | 125 | 0 |
2 | 141 | 93 | 48 |
3 | 125 | 94 | 31 |
4 | 125 | 109 | 16 |
5 | 140 | 93 | 47 |
There is not much optimization done in this case as we should understand that there is no scope for improvement.
Shall we run a standard algorithm and see the performance and I chose the bubble sort. I have used a random number generator to generate the candidate numbers for sorting. I sorted 9999 numbers and recorded the following milli seconds.
package com.javapapers.corejava; import java.util.Random; public class BubbleSort { public static void main(String args[]) { long start = System.currentTimeMillis(); int numbers[] = generateNumbers(); bubbleSort(numbers); long end = System.currentTimeMillis(); System.out.println("Duration: " + (end - start)); } public static int[] generateNumbers() { int numbers[] = new int[9999]; Random random = new Random(); for (int i = 0; i < 9999; i++) numbers[i] = random.nextInt(); return numbers; } public static void bubbleSort(int numbers[]) { int temp; int numbersCount = numbers.length; for (int i = 0; i < numbersCount; i++) { for (int j = 1; j < (numbersCount - i); j++) { if (numbers[j - 1] > numbers[j]) { temp = numbers[j - 1]; numbers[j - 1] = numbers[j]; numbers[j] = temp; } } } } }
Run | Client Mode | Server Mode | Difference |
---|---|---|---|
1 | 171 | 156 | 15 |
2 | 172 | 156 | 16 |
3 | 172 | 156 | 16 |
4 | 172 | 156 | 16 |
5 | 171 | 171 | 0 |
Not much improvement in this case either.
I thought of running the program for a little bit longer with input larger input 99999. That is to sort 99999 number.
Run | Client Mode | Server Mode | Difference |
---|---|---|---|
1 | 19687 | 19502 | 185 |
2 | 20471 | 19441 | 1030 |
3 | 19422 | 19410 | 12 |
4 | 20513 | 19383 | 1130 |
5 | 19722 | 19353 | 369 |
These experiments were conducted using Oracle’s JDK (client and server mode).
Comments are closed for "Performance of JVM Server and Client Mode".
very useful article..
Hi Joe,
Thanks for sharing this wonderful information, i tried the same you said but i am not getting much difference, even many of time both are same, both are sticking with 4 and 5.
why is this so, does it also depends on machine configuration?
Interesting, Thank You Joe
I like this.
[…] In this example, we will use Java instrumentation to modify the loaded bytecode (java classes) and add statements. Using which we will find out how much duration a method executes. This is a real time use case for java performance profiling. […]