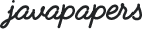
Sending email in java using Gmail SMTP and JavaMail API is fun. Core of sending email is done by JavaMail api (using GMail) and we just need to pass the required email parameters to it. By every release, JavaMail API is getting sophisticated and sending email with GMail is jut a click away.
I have used JavaMail API version 1.4.5 and should add two jars as dependency for sending email mailapi.jar and smtp.jar
package com.javapapers.java; import java.util.Properties; import javax.mail.Message; import javax.mail.MessagingException; import javax.mail.Session; import javax.mail.Transport; import javax.mail.internet.AddressException; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeMessage; public class JavaEmail { Properties emailProperties; Session mailSession; MimeMessage emailMessage; public static void main(String args[]) throws AddressException, MessagingException { JavaEmail javaEmail = new JavaEmail(); javaEmail.setMailServerProperties(); javaEmail.createEmailMessage(); javaEmail.sendEmail(); } public void setMailServerProperties() { String emailPort = "587";//gmail's smtp port emailProperties = System.getProperties(); emailProperties.put("mail.smtp.port", emailPort); emailProperties.put("mail.smtp.auth", "true"); emailProperties.put("mail.smtp.starttls.enable", "true"); } public void createEmailMessage() throws AddressException, MessagingException { String[] toEmails = { "joe@javapapers.com" }; String emailSubject = "Java Email"; String emailBody = "This is an email sent by JavaMail api."; mailSession = Session.getDefaultInstance(emailProperties, null); emailMessage = new MimeMessage(mailSession); for (int i = 0; i < toEmails.length; i++) { emailMessage.addRecipient(Message.RecipientType.TO, new InternetAddress(toEmails[i])); } emailMessage.setSubject(emailSubject); emailMessage.setContent(emailBody, "text/html");//for a html email //emailMessage.setText(emailBody);// for a text email } public void sendEmail() throws AddressException, MessagingException { String emailHost = "smtp.gmail.com"; String fromUser = "your emailid here";//just the id alone without @gmail.com String fromUserEmailPassword = "your email password here"; Transport transport = mailSession.getTransport("smtp"); transport.connect(emailHost, fromUser, fromUserEmailPassword); transport.sendMessage(emailMessage, emailMessage.getAllRecipients()); transport.close(); System.out.println("Email sent successfully."); } }
It is all in the parameters we have set. Just refer the GMail SMTP settings and it does the job. If we need to use other SMTP servers than GMail, then we need to configure its respective settings in the code. Code wise nothing will change and sending email in Java is as simple as that.
Comments are closed for "Send Email in Java using GMail SMTP with JavaMail".
Nice article! One question though. Do we need to do anything to set up the SMTP server on the local machine, or does the smtp.jar take care of everything?
It doesn’t use SMTP at the local host. So no need to set up anything there. It uses the mail server set in the variable emailHost.
Microsoft Windows [Version 6.1.7601]
Copyright (c) 2009 Microsoft Corporation. All rights reserved.
C:\Users\nani\Desktop\java>javac JavaEmail.java
JavaEmail.java:71: reached end of file while parsing
}
^
1 error
C:\Users\nani\Desktop\java>javac JavaEmail.java
JavaEmail.java:5: package javax.mail does not exist
import javax.mail.Message;
^
JavaEmail.java:6: package javax.mail does not exist
import javax.mail.MessagingException;
^
………
Hi, I am getting this error. I have included the jars smtp.jar, mailapi.jar. Could you please suggest…..
Exception in thread “main” java.lang.NoSuchMethodError: com.sun.mail.util.SocketFetcher.getSocket(Ljava/lang/String;ILjava/util/Properties;Ljava/lang/String;Z)Ljava/net/Socket;
at com.sun.mail.smtp.SMTPTransport.openServer(SMTPTransport.java:1250)
at com.sun.mail.smtp.SMTPTransport.protocolConnect(SMTPTransport.java:370)
at javax.mail.Service.connect(Service.java:233)
at javax.mail.Service.connect(Service.java:134)
at JavaEmail.sendEmail(JavaEmail.java:60)
at JavaEmail.main(JavaEmail.java:22)
java.lang.IllegalStateException: already connected
at javax.mail.Service.connect(Service.java:214)
at javax.mail.Service.connect(Service.java:172)
at javax.mail.Service.connect(Service.java:121)
nice tutorial
Good one
Very Good one.. Thank u.. :)
i want to send bulk mail, is it possible?
please explain.
you have to set classpath for that jar file and for the class file directory…
super sir…………..
sir I am getting 20 errors pls explain how to solve the errors and how to execute
James,
use eclipse add jars, and add them to build path its simple and easy.
hi joe im getting the following error,i have added the mailapi.jar and smtp.jar.
Exception in thread “main” javax.mail.NoSuchProviderException: smtp
at javax.mail.Session.getService(Session.java:782)
at javax.mail.Session.getTransport(Session.java:708)
at javax.mail.Session.getTransport(Session.java:651)
at javax.mail.Session.getTransport(Session.java:631)
at SendingMail.sendEmail(SendingMail.java:29)
at SendingMail.main(SendingMail.java:21)
You are getting NoSuchProviderException: smtp – looks like you have changed some configuration. emailHost should be smtp.gmail.com
Niket, it is possible to sen bulk mail. We should write a mailer program however we should consider factors like how mails the smtp gateway will allow during a block of time and set proper headers. Otherwise there is a possibility the stmp provider will block the service. Need to use multithreading and write the program efficiently.
@^ Joe, just adding the large no. of recipients in the “String[] toEmails” will not work.
To avoid exception, please download jar file from oracle website (mailapi.jar, smtp.jar)
download JavaMail API
Quite neat explanation.
thanks Joe!!!
currently i was working on the same and u made it easy :)
Great Job.
Nicely done…I’m going to try this one… keep up the great work… u inspire me to be a great programmer one day…. thanks,,,
Nice work – looking forward to trying this tonight – also is it a coincidence that I wanted to create a java email application for a database I’m working on? I think not! Now I just have to connect them – Cheers!
hai please help me how to get the mail “receving date” from our mails…….
very nice article.
Thanks… It’s working :)
Hi..
I am getting the following error:
Exception in thread “main” javax.mail.MessagingException: Could not connect to SMTP host: smtp.gmail.com, port: 587;
Can you help me to sort out this error.
Is it possible to send email without passing password of from email id..??
Yes, it is possible and depends on the configuration of mail SMTP service provider. Generally authentication is enforced to avoid misuse of the server.
Check if you have internet connection and if you have proxy server verify it doesn’t block the smtp port.
are you referring to bulk mail?
check if you have added the jars correctly to the classpath
Thanks for ur reply. I have the internet connection and proxy server is der and I think it is blocking the smtp port. Is there any way to avoid dis proxy server??
One more thing if I have to call the receipents id’s from the database then what shld be d changes that need to be made??
how we can change the desktop background using the java program or batch file
joe,
I have tried the above program.It’s working fine.Thanks for your Excellent Guidence.
I am facing one issue,whatever i have send the email through this program it’s going to Junk Emal/Spam Folder.How do we avoid that situation.
Please suggest me on this
Thanks
Regards,
Manikandan
Mani,
We need to set email headers and try to send a meaningful email then this will not go to spam folder.
Hi Joe,Can u please specify the SMTP details of yahoo mail(ymail).
Thanks,
Praveen.
Thanks posts like this……..
Hi Joe,
Very nice article However It also has very nice functionalities apart from send mail.
like
1) Send mail with attachment
2) send mail to the multiple email ids
sir,
How to set email header ?
I like use apache commons-email to send email, haha !
thanks joe sir
bt i have one query
does gmail provide unauthorised sending …i mean without entering the gmail password….is it possible..
my requirement is that i am building website now in contact us form… we will bw asking
his email id
subject only..
content
so asking for password frm user is nt worth…nobody will trust …
so kindly help me out !!!!!!!!!!!
Deepak,
Gmail does not allows to send anonymous emails. We must authenticate to send email using Gmail SMTP server.
sir,
I want to write a program in java to use all concept of oops in a program? plz help me ….
Hi I am able to do it through the outlook smtp server but not with the gmail.. why is that??
Hello sir please let me know how can i send attachment to someone.
I tried using your program but get this exception. How to tackle with the proxy server issue ?
Exception in thread “main” javax.mail.MessagingException: Could not connect to SMTP host: smtp.gmail.com, port: 587;
nested exception is:
java.net.ConnectException: Connection refused: connect
hi,
I tried using the following code to attach file.It’s working.
Add this snippet in createEmailMessage():
File f = new File(Filepath);
MimeBodyPart msg = new MimeBodyPart();
msg.setText(“Hi Testing mail”);
Multipart m = new MimeMultipart();
m.addBodyPart(msg);
msg = new MimeBodyPart();
DataSource ds = new FileDataSource(f);
msg.setDataHandler(new DataHandler(ds));
msg.setFileName(name of the file with extension);
m.addBodyPart(msg);
emailMessage.setSubject(emailSubject);
//emailMessage.setContent(emailBody, “text/html”);//for a html email
//emailMessage.setText(emailBody);// for a text email
emailMessage.setContent(m);
hi,
I tried using the following code to attach file.It’s working.
Add this snippet in createEmailMessage():
File f = new File(Filepath);
MimeBodyPart msg = new MimeBodyPart();
msg.setText(“Hi Testing mail”);
Multipart m = new MimeMultipart();
m.addBodyPart(msg);
msg = new MimeBodyPart();
DataSource ds = new FileDataSource(f);
msg.setDataHandler(new DataHandler(ds));
msg.setFileName(name of the file with extension);
m.addBodyPart(msg);
emailMessage.setSubject(emailSubject);
//emailMessage.setContent(emailBody, “text/html”);//for a html email
//emailMessage.setText(emailBody);// for a text email
emailMessage.setContent(m);
i have the sane issue….please help me…we are using proxy server..java mail not sending through proxy server.
Sir, how do the program know tat our mail is meaningful or not… pls explain..
how to send bulk mailing using java mail api……i mean above 1000 mails
what is that package called package com.javapapers.java;
It is a custom package which I use for my programs :-)
After setting classpath as follows:
D:\java assignments>set CLASSPATH=”C:\Program Files\Java\javamail-1.4.6\mail.jar
“;
Im getting following error:
D:\java assignments>javac JavaEmail.java
JavaEmail.java:5: error: package javax.mail does not exist
import javax.mail.Message;
^
JavaEmail.java:6: error: package javax.mail does not exist
import javax.mail.MessagingException;
^
JavaEmail.java:7: error: package javax.mail does not exist
import javax.mail.Session;
^
JavaEmail.java:8: error: package javax.mail does not exist
import javax.mail.Transport;
^
JavaEmail.java:9: error: package javax.mail.internet does not exist
import javax.mail.internet.AddressException;
^
JavaEmail.java:10: error: package javax.mail.internet does not exist
import javax.mail.internet.InternetAddress;
^
JavaEmail.java:11: error: package javax.mail.internet does not exist
import javax.mail.internet.MimeMessage;
^
JavaEmail.java:17: error: cannot find symbol
Sesion mailSession;
^
symbol: class Sesion
location: class JavaEmail
JavaEmail.java:18: error: cannot find symbol
MimeMessage emailMessage;
^
symbol: class MimeMessage
location: class JavaEmail
JavaEmail.java:20: error: cannot find symbol
public static void main(String args[]) throws AddressException,Messaging
Exception
^
symbol: class AddressException
location: class JavaEmail
JavaEmail.java:20: error: cannot find symbol
public static void main(String args[]) throws AddressException,Messaging
Exception
^
symbol: class MessagingException
location: class JavaEmail
JavaEmail.java:42: error: cannot find symbol
public void createEmailMessage() throws AddressException,MessagingExcept
ion
^
symbol: class AddressException
location: class JavaEmail
JavaEmail.java:42: error: cannot find symbol
public void createEmailMessage() throws AddressException,MessagingExcept
ion
^
symbol: class MessagingException
location: class JavaEmail
JavaEmail.java:62: error: cannot find symbol
public void sendEmail() throws AddressException, MessagingException
^
symbol: class AddressException
location: class JavaEmail
JavaEmail.java:62: error: cannot find symbol
public void sendEmail() throws AddressException, MessagingException
^
symbol: class MessagingException
location: class JavaEmail
JavaEmail.java:48: error: cannot find symbol
mailSession = Session.getDefaultInstance(emailProperties, null);
^
symbol: variable Session
location: class JavaEmail
JavaEmail.java:49: error: cannot find symbol
emailMessage = new MimeMessage(mailSession);
^
symbol: class MimeMessage
location: class JavaEmail
JavaEmail.java:53: error: package Message does not exist
emailMessage.addRecipient(Message.RecipientType.TO, new
InternetAddress(toEmails[i]));
^
JavaEmail.java:53: error: cannot find symbol
emailMessage.addRecipient(Message.RecipientType.TO, new
InternetAddress(toEmails[i]));
^
symbol: class InternetAddress
location: class JavaEmail
JavaEmail.java:69: error: cannot find symbol
Transport transport = mailSession.getTransport(“smtp”);
^
symbol: class Transport
location: class JavaEmail
20 errors
Please help im unable to debug the error..
Hi,
My mail contains one pdf file as an attachment.after sending mail it prints pdf content in encrypted format server.log file of jboss 5.I do not want this in log file.Do you have any suggestion?
may be your mail.jar file is corrupted just open the jar file and check whether above used classes are there in the mail.jar file or not if not there then try to download it again
Hi Joe,
Using java, I can send mails but unfortunately those mails sent to spam folder(only few times), how to restrict.
javax.mail.MessagingException: Could not connect to SMTP host: smtp.gmail.
com, port: 465, response: -1
Hi Joe,
An exception will ocuur at runtime, try to give me the solution for the same…
It is the exception occur when i give 587 smtp port number
javax.mail.AuthenticationFailedException
Hi Joe,
I am getting the below error. But I have not changed String emailHost = “smtp.gmail.com”;
I have just changed fromUser and fromUserEmailPassword. Could you please help me on this.
Exception in thread “main” javax.mail.NoSuchProviderException: smtp
at javax.mail.Session.getService(Session.java:782)
at javax.mail.Session.getTransport(Session.java:708)
at javax.mail.Session.getTransport(Session.java:651)
at javax.mail.Session.getTransport(Session.java:631)
at com.email.JavaEmail.sendEmail(JavaEmail.java:65)
at com.email.JavaEmail.main(JavaEmail.java:26)
Dear Sir,
I am in danger of losing my Job.I have a problem and that is I have a form to be submitted and multiple mails are to be sent from when clicking the submit button but when I submit it takes me over 45 seconds to send all the mails, but I want to send the mails in the backend without the user getting to wait for the success message, can you please give me an nice example of how to do that, please help me sir
How to retrieve mail using POP3 ?
I have run this program as it is, just enterd my user name,
I got bellow error:
Exception in thread “main” javax.mail.MessagingException: Could not connect to SMTP host: smtp.gmail.com, port: 587;
nested exception is:
java.net.ConnectException: Connection timed out: connect
at com.sun.mail.smtp.SMTPTransport.openServer(SMTPTransport.java:1934)
at com.sun.mail.smtp.SMTPTransport.protocolConnect(SMTPTransport.java:638)
at javax.mail.Service.connect(Service.java:295)
at javax.mail.Service.connect(Service.java:176)
at WebDriver.Email.sendEmail(Email.java:69)
at WebDriver.Email.main(Email.java:28)
Caused by: java.net.ConnectException: Connection timed out: connect
at java.net.DualStackPlainSocketImpl.connect0(Native Method)
at java.net.DualStackPlainSocketImpl.socketConnect(Unknown Source)
at java.net.AbstractPlainSocketImpl.doConnect(Unknown Source)
at java.net.AbstractPlainSocketImpl.connectToAddress(Unknown Source)
at java.net.AbstractPlainSocketImpl.connect(Unknown Source)
at java.net.PlainSocketImpl.connect(Unknown Source)
at java.net.SocksSocketImpl.connect(Unknown Source)
at java.net.Socket.connect(Unknown Source)
at java.net.Socket.connect(Unknown Source)
at com.sun.mail.util.SocketFetcher.createSocket(SocketFetcher.java:288)
at com.sun.mail.util.SocketFetcher.getSocket(SocketFetcher.java:231)
at com.sun.mail.smtp.SMTPTransport.openServer(SMTPTransport.java:1900)
… 5 more
I am using latest api – javax.mail-1.5.0.jar
Hai Sir,
i did everything properly.
but i am getting the following exception
plz suggest me what to do…
Exception in thread “main” javax.mail.MessagingException: Could not convert socket to TLS;
nested exception is:
javax.net.ssl.SSLHandshakeException: sun.security.validator.ValidatorException: PKIX path building failed: sun.security.provider.certpath.SunCertPathBuilderException: unable to find valid certification path to requested target
at com.sun.mail.smtp.SMTPTransport.startTLS(SMTPTransport.java:1907)
at com.sun.mail.smtp.SMTPTransport.protocolConnect(SMTPTransport.java:666)
at javax.mail.Service.connect(Service.java:295)
at javax.mail.Service.connect(Service.java:176)
at JavaEmail.sendEmail(JavaEmail.java:67)
at JavaEmail.main(JavaEmail.java:24)
Caused by: javax.net.ssl.SSLHandshakeException: sun.security.validator.ValidatorException: PKIX path building failed: sun.security.provider.certpath.SunCertPathBuilderException: unable to find valid certification path to requested target
at sun.security.ssl.Alerts.getSSLException(Alerts.java:192)
at sun.security.ssl.SSLSocketImpl.fatal(SSLSocketImpl.java:1886)
at sun.security.ssl.Handshaker.fatalSE(Handshaker.java:276)
at sun.security.ssl.Handshaker.fatalSE(Handshaker.java:270)
at sun.security.ssl.ClientHandshaker.serverCertificate(ClientHandshaker.java:1341)
at sun.security.ssl.ClientHandshaker.processMessage(ClientHandshaker.java:153)
at sun.security.ssl.Handshaker.processLoop(Handshaker.java:868)
at sun.security.ssl.Handshaker.process_record(Handshaker.java:804)
at sun.security.ssl.SSLSocketImpl.readRecord(SSLSocketImpl.java:1016)
at sun.security.ssl.SSLSocketImpl.performInitialHandshake(SSLSocketImpl.java:1312)
at sun.security.ssl.SSLSocketImpl.startHandshake(SSLSocketImpl.java:1339)
at sun.security.ssl.SSLSocketImpl.startHandshake(SSLSocketImpl.java:1323)
at com.sun.mail.util.SocketFetcher.configureSSLSocket(SocketFetcher.java:549)
at com.sun.mail.util.SocketFetcher.startTLS(SocketFetcher.java:486)
at com.sun.mail.smtp.SMTPTransport.startTLS(SMTPTransport.java:1902)
… 5 more
Caused by: sun.security.validator.ValidatorException: PKIX path building failed: sun.security.provider.certpath.SunCertPathBuilderException: unable to find valid certification path to requested target
at sun.security.validator.PKIXValidator.doBuild(PKIXValidator.java:385)
at sun.security.validator.PKIXValidator.engineValidate(PKIXValidator.java:292)
at sun.security.validator.Validator.validate(Validator.java:260)
at sun.security.ssl.X509TrustManagerImpl.validate(X509TrustManagerImpl.java:326)
at sun.security.ssl.X509TrustManagerImpl.checkTrusted(X509TrustManagerImpl.java:231)
at sun.security.ssl.X509TrustManagerImpl.checkServerTrusted(X509TrustManagerImpl.java:126)
at sun.security.ssl.ClientHandshaker.serverCertificate(ClientHandshaker.java:1323)
… 15 more
Caused by: sun.security.provider.certpath.SunCertPathBuilderException: unable to find valid certification path to requested target
at sun.security.provider.certpath.SunCertPathBuilder.engineBuild(SunCertPathBuilder.java:196)
at java.security.cert.CertPathBuilder.build(CertPathBuilder.java:268)
at sun.security.validator.PKIXValidator.doBuild(PKIXValidator.java:380)
… 21 more
the above Exception was resolved…
just put following code….
emailProperties.setProperty(“mail.smtp.ssl.trust”, “smpt server”);
for gmail smpt server : smtp.gmail.com
then it will work…
thank you…
Goodmorning SIr im an Insurance agent i want to send bulk sms can u plz help me out
how to solve this proble??
and does it possible to send mail to Outlook Email too
Exception in thread “main” javax.mail.MessagingException: can’t determine local email address
at com.sun.mail.smtp.SMTPTransport.mailFrom(SMTPTransport.java:581)
at com.sun.mail.smtp.SMTPTransport.sendMessage(SMTPTransport.java:319)
at Poonam.send_demo.sendEmail(send_demo.java:69)
at Poonam.send_demo.main(send_demo.java:27)
Thanks
Poonam
[…] already wrote a tutorial for sending email using Java and GMail SMTP with JavaMail API. Refer that for understanding about the core service of sending email. It has got couple of […]
Nice article! Please also post an article on “How to send msg to mobile using JSE application”
I used javax.mail.jar 5.0 and got the following issue.
I tried mostly all sorts of from address.
Exception in thread “main” javax.mail.MessagingException: can’t determine local email address
at com.sun.mail.smtp.SMTPTransport.mailFrom(SMTPTransport.java:1576)
at com.sun.mail.smtp.SMTPTransport.sendMessage(SMTPTransport.java:1132)
at JavaEmail.sendEmail(JavaEmail.java:66)
at JavaEmail.main(JavaEmail.java:24)
Can you help me in figuring out this issue?
[…] far we have seen how we can send email in Java using JavaMail API and then an email app in Android using JavaMail API and GMail SMTP. Now to complete this email […]
How to send email to multiple recipients.
Thank you Sir. The code you have provided is very helpful and its very easy to understand as you written it in a simple way. Thank you.
Hi,
I would like to send same type of email, what you are sending from gmail, from my business email. Will I be able to do that? It is an microsoft exchange server. What are all the configuration changes required?
code not working in eclipse
error generated following….
Exception in thread “main” javax.mail.MessagingException: Could not convert socket to TLS;
nested exception is:
javax.net.ssl.SSLHandshakeException: sun.security.validator.ValidatorException: PKIX path building failed: sun.security.provider.certpath.SunCertPathBuilderException: unable to find valid certification path to requested target
at com.sun.mail.smtp.SMTPTransport.startTLS(SMTPTransport.java:1907)
at com.sun.mail.smtp.SMTPTransport.protocolConnect(SMTPTransport.java:666)
at javax.mail.Service.connect(Service.java:295)
at javax.mail.Service.connect(Service.java:176)
at test.JavaEmail.sendEmail(JavaEmail.java:66)
at test.JavaEmail.main(JavaEmail.java:25)
Caused by: javax.net.ssl.SSLHandshakeException: sun.security.validator.ValidatorException: PKIX path building failed: sun.security.provider.certpath.SunCertPathBuilderException: unable to find valid certification path to requested target
at com.sun.net.ssl.internal.ssl.Alerts.getSSLException(Unknown Source)
at com.sun.net.ssl.internal.ssl.SSLSocketImpl.fatal(Unknown Source)
at com.sun.net.ssl.internal.ssl.Handshaker.fatalSE(Unknown Source)
at com.sun.net.ssl.internal.ssl.Handshaker.fatalSE(Unknown Source)
at com.sun.net.ssl.internal.ssl.ClientHandshaker.serverCertificate(Unknown Source)
at com.sun.net.ssl.internal.ssl.ClientHandshaker.processMessage(Unknown Source)
at com.sun.net.ssl.internal.ssl.Handshaker.processLoop(Unknown Source)
at com.sun.net.ssl.internal.ssl.Handshaker.process_record(Unknown Source)
at com.sun.net.ssl.internal.ssl.SSLSocketImpl.readRecord(Unknown Source)
at com.sun.net.ssl.internal.ssl.SSLSocketImpl.performInitialHandshake(Unknown Source)
at com.sun.net.ssl.internal.ssl.SSLSocketImpl.startHandshake(Unknown Source)
at com.sun.net.ssl.internal.ssl.SSLSocketImpl.startHandshake(Unknown Source)
at com.sun.mail.util.SocketFetcher.configureSSLSocket(SocketFetcher.java:549)
at com.sun.mail.util.SocketFetcher.startTLS(SocketFetcher.java:486)
at com.sun.mail.smtp.SMTPTransport.startTLS(SMTPTransport.java:1902)
… 5 more
Caused by: sun.security.validator.ValidatorException: PKIX path building failed: sun.security.provider.certpath.SunCertPathBuilderException: unable to find valid certification path to requested target
at sun.security.validator.PKIXValidator.doBuild(Unknown Source)
at sun.security.validator.PKIXValidator.engineValidate(Unknown Source)
at sun.security.validator.Validator.validate(Unknown Source)
at com.sun.net.ssl.internal.ssl.X509TrustManagerImpl.validate(Unknown Source)
at com.sun.net.ssl.internal.ssl.X509TrustManagerImpl.checkServerTrusted(Unknown Source)
at com.sun.net.ssl.internal.ssl.X509TrustManagerImpl.checkServerTrusted(Unknown Source)
… 16 more
Caused by: sun.security.provider.certpath.SunCertPathBuilderException: unable to find valid certification path to requested target
at sun.security.provider.certpath.SunCertPathBuilder.engineBuild(Unknown Source)
at java.security.cert.CertPathBuilder.build(Unknown Source)
… 22 more
sorry its working, thanks
its my antivirus blocking error
Good One
I get the below error “Exception in thread “main” com.sun.mail.smtp.SMTPSendFailedException: 530 5.7.0 Must issue a STARTTLS command first. sg1sm32499226pbb.16 – gsmtp”. Please anyone tell me how do i resolve it?
Exception in thread “main” javax.mail.MessagingException: Could not convert soc
et to TLS;
nested exception is:
javax.net.ssl.SSLHandshakeException: sun.security.validator.ValidatorEx
eption: PKIX path building failed: sun.security.provider.certpath.SunCertPathBu
lderException: unable to find valid certification path to requested target
at com.sun.mail.smtp.SMTPTransport.startTLS(SMTPTransport.java:1907)
at com.sun.mail.smtp.SMTPTransport.protocolConnect(SMTPTransport.java:6
6)
at javax.mail.Service.connect(Service.java:295)
at javax.mail.Service.connect(Service.java:176)
at JavaEmail.sendEmail(JavaEmail.java:63)
at JavaEmail.main(JavaEmail.java:22)
Caused by: javax.net.ssl.SSLHandshakeException: sun.security.validator.Validato
Exception: PKIX path building failed: sun.security.provider.certpath.SunCertPat
BuilderException: unable to find valid certification path to requested target
at sun.security.ssl.Alerts.getSSLException(Unknown Source)
at sun.security.ssl.SSLSocketImpl.fatal(Unknown Source)
at sun.security.ssl.Handshaker.fatalSE(Unknown Source)
at sun.security.ssl.Handshaker.fatalSE(Unknown Source)
at sun.security.ssl.ClientHandshaker.serverCertificate(Unknown Source)
at sun.security.ssl.ClientHandshaker.processMessage(Unknown Source)
at sun.security.ssl.Handshaker.processLoop(Unknown Source)
at sun.security.ssl.Handshaker.process_record(Unknown Source)
at sun.security.ssl.SSLSocketImpl.readRecord(Unknown Source)
at sun.security.ssl.SSLSocketImpl.performInitialHandshake(Unknown Sourc
)
at sun.security.ssl.SSLSocketImpl.startHandshake(Unknown Source)
at sun.security.ssl.SSLSocketImpl.startHandshake(Unknown Source)
at com.sun.mail.util.SocketFetcher.configureSSLSocket(SocketFetcher.jav
:549)
at com.sun.mail.util.SocketFetcher.startTLS(SocketFetcher.java:486)
at com.sun.mail.smtp.SMTPTransport.startTLS(SMTPTransport.java:1902)
… 5 more
Caused by: sun.security.validator.ValidatorException: PKIX path building failed
sun.security.provider.certpath.SunCertPathBuilderException: unable to find val
d certification path to requested target
at sun.security.validator.PKIXValidator.doBuild(Unknown Source)
at sun.security.validator.PKIXValidator.engineValidate(Unknown Source)
at sun.security.validator.Validator.validate(Unknown Source)
at sun.security.ssl.X509TrustManagerImpl.validate(Unknown Source)
at sun.security.ssl.X509TrustManagerImpl.checkTrusted(Unknown Source)
at sun.security.ssl.X509TrustManagerImpl.checkServerTrusted(Unknown Sou
ce)
… 16 more
Caused by: sun.security.provider.certpath.SunCertPathBuilderException: unable t
find valid certification path to requested target
at sun.security.provider.certpath.SunCertPathBuilder.engineBuild(Unknow
Source)
at java.security.cert.CertPathBuilder.build(Unknown Source)
… 22 more
I have tried this by adding the jars in classpath but i’m getting below exception
Exception in thread “main” javax.mail.MessagingException: can’t determine local email address
at com.sun.mail.smtp.SMTPTransport.mailFrom(SMTPTransport.java:1576)
at com.sun.mail.smtp.SMTPTransport.sendMessage(SMTPTransport.java:1132)
at com.apollo.client.JavaEmail.sendEmail(JavaEmail.java:68)
at com.apollo.client.JavaEmail.main(JavaEmail.java:26)
Thanks also provide some tips to send SMS……
Sir I am getting the following error please help me
Exception in thread “main” javax.mail.NoSuchProviderException: smtp
at javax.mail.Session.getService(Session.java:792)
at javax.mail.Session.getTransport(Session.java:728)
at javax.mail.Session.getTransport(Session.java:668)
at javax.mail.Session.getTransport(Session.java:648)
at com.javapapers.java.JavaEmail.sendEmail(JavaEmail.java:65)
at com.javapapers.java.JavaEmail.main(JavaEmail.java:26)
i am getting following exception javax.mail.MessagingException: can’t determine local email address
how to slove……..please explain
HI I am using following code to send mail in JSP.
I have set teh class path for mail.jar and activation.jar.
Below is my code and i am getting message exception:
Error:
I do not get any stack trace error. I get “message exception” on my webpage and i do not receive mail.
Can someone please tell what is the mistake here?
Hi
Please help me to resolve the the below error.
Exception in thread “main” javax.mail.MessagingException: can’t determine local email address
at com.sun.mail.smtp.SMTPTransport.mailFrom(SMTPTransport.java:1576)
at com.sun.mail.smtp.SMTPTransport.sendMessage(SMTPTransport.java:1132)
at JavaEmail.sendEmail(JavaEmail.java:67)
at JavaEmail.main(JavaEmail.java:24)
I really like your blog.. very nice colors & theme.
Did you make this website yourself or did you hire
someone to do it for you? Plz reply as I’m looking to create
my own blog and would like to find out where u got this from.
cheers
My web site – JOSEPH PANTEL (Dustin)
Thanks Dustin. Happy that you like the design.
I am using WordPress as platform. I have created my own theme. Do you want to hire me to do similar work?
Hi Joe,
I have a java program which reads the details from DB and send to SMTP server. I used SimpleMail for sending the mail.
How can I handle the bounced back mails in the program?
I just want to know which all mails bounced back.
Thanks in advance
Hello,
Isn’t it a little strange that I have to hardcode my password in order to send emails.
I’m not programming a website yet, just doing exercises but wouldn’t there be a danger in writing code that has my gmail account’s password explicitly stated in it.
Thanks, I’m quite new to programming.
_
i got folliwing error….
Exception in thread “main” javax.mail.AuthenticationFailedException
at javax.mail.Service.connect(Service.java:267)
at javax.mail.Service.connect(Service.java:137)
at JavaEmail.sendEmail(JavaEmail.java:67)
at JavaEmail.main(JavaEmail.java:26)
…..
can you help me with this problem…
Yes thats right. To just explain a concept and for tutorial purposes it is fine.
The real implementation should never have password (any password) put in a plain text file. It is not safe. We must keep it in an encrypted form.
Hi,
Im getting below error,
javax.mail.MessagingException: Could not connect to SMTP host: smtp.gmail.com, port: 587;
nested exception is:
java.net.ConnectException: Connection refused: connect
at com.sun.mail.smtp.SMTPTransport.openServer(SMTPTransport.java:1282)
at com.sun.mail.smtp.SMTPTransport.protocolConnect(SMTPTransport.java:370)
at javax.mail.Service.connect(Service.java:275)
at javax.mail.Service.connect(Service.java:156)
at com.mkyong.common.test.sendFromGMail(test.java:53)
at com.mkyong.common.test.main(test.java:21)
Caused by: java.net.ConnectException: Connection refused: connect
at java.net.PlainSocketImpl.socketConnect(Native Method)
at java.net.PlainSocketImpl.doConnect(PlainSocketImpl.java:351)
at java.net.PlainSocketImpl.connectToAddress(PlainSocketImpl.java:213)
at java.net.PlainSocketImpl.connect(PlainSocketImpl.java:200)
at java.net.SocksSocketImpl.connect(SocksSocketImpl.java:366)
at java.net.Socket.connect(Socket.java:529)
at java.net.Socket.connect(Socket.java:478)
at com.sun.mail.util.SocketFetcher.createSocket(SocketFetcher.java:232)
at com.sun.mail.util.SocketFetcher.getSocket(SocketFetcher.java:189)
at com.sun.mail.smtp.SMTPTransport.openServer(SMTPTransport.java:1250)
… 5 more
I am using similar code to send email. Now the requirement is to send secure email. So I want to send email using SMTPS and port 465. What changes I will have do?
Everything is very open with a very clear description of
the issues. It was really informative. Your website is very useful.
Many thank for sharing!
works!!
Happy to hear that :-)
There is no such thing as javax.mail??!! Help me!!!!
javax.mail Java Mail API is not part of the default Java SE package. You need to download the jar separately and add it to your application classpath.
You can download Java Mail package from:
http://www.oracle.com/technetwork/java/javamail/index.html