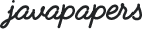
Android In-App Browser will allow retain the users inside your APP. Which is a critical thing nowadays. Retaining an user and making him stay inside our APP as much as possible without fading away is important. This will boost the engagement metrics.
Android platform by default does not provide local browser. When a link is clicked, it opens in the device’s default browser. It make user exit the application. This is the reason why any popular APPs have Android In-App browser built inside.
In this tutorial we will learn to build an Android In-App Browser using WebView. With this app we will be able to,
1. Open any website by typing the URl in the app.
2. Set a home page for our browser.
3. Open a locally saved webpage in our Android App.
To build this Android APP, we will use a webview to display webpages. We will make an editText, in which user will enter URL and a button which on click would load the URL. We will make a toolbar which would have three menu items –Home, file(locally saved web page) and refresh.
This Android App is kept simple to demonstrate the use of WebView, but you could add other features and .
1. Android Manifest
We should add Internet permission in the Android Manifest file.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.javapapers.android.browser"> <uses-permission android:name="android.permission.INTERNET"></uses-permission> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity" android:label="@string/app_name" android:theme="@style/AppTheme.NoActionBar"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
2. Styles.xml
We will create our action bar, so we will remove the default action bar. For this we have to change the app Theme to ‘Theme.AppCompat.Light.DarkActionBar’.
<resources> <style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar"> <item name="colorPrimary">@color/colorPrimary</item> <item name="colorPrimaryDark">@color/colorPrimaryDark</item> <item name="colorAccent">@color/colorAccent</item> </style> <style name="AppTheme.NoActionBar"> <item name="windowActionBar">false</item> <item name="windowNoTitle">true</item> </style> <style name="AppTheme.AppBarOverlay" parent="ThemeOverlay.AppCompat.Dark.ActionBar" /> <style name="AppTheme.PopupOverlay" parent="ThemeOverlay.AppCompat.Light" /> </resources>
3. menu.xml
Now We will create a menu.xml file in menu directory which holds the content of toolbar.
<menu xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" tools:context="com.javapapers.browser.MainActivity"> <item android:id="@+id/action_Home" android:icon="@drawable/home" android:orderInCategory="100" android:title="action_bookmark" app:showAsAction="always" /> <item android:id="@+id/action_file" android:icon="@drawable/file" android:orderInCategory="101" android:title="file" app:showAsAction="always" /> <item android:id="@+id/action_refresh" android:icon="@drawable/refresh" android:orderInCategory="102" android:title="refresh" app:showAsAction="always" />
4. content_main.xml
Now we will create a resource file that holds the content of activity main. It has a webview, an editText and a button.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#FF4081" app:layout_behavior="@string/appbar_scrolling_view_behavior" tools:context="com.javapapers.browser.MainActivity" tools:showIn="@layout/activity_main"> <EditText android:id="@+id/editText" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignBottom="@+id/b1" android:layout_alignParentLeft="true" android:hint="Enter URL ex-www.javapapers.com" /> <Button android:id="@+id/b1" android:layout_width="50dp" android:layout_height="wrap_content" android:layout_alignParentRight="true" android:layout_alignParentTop="true" android:background="@drawable/forward" android:onClick="click" /> <WebView android:id="@+id/webView1" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_below="@+id/editText" android:layout_centerHorizontal="true" /> <ProgressBar android:id="@+id/progressBar" style="?android:attr/progressBarStyleLarge" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_centerVertical="true" /> </RelativeLayout>
5. Main Activity
activity_main.xml
I have added toolbar and a progressBar that would show loading of webpages.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#FF4081" app:layout_behavior="@string/appbar_scrolling_view_behavior" tools:context="com.javapapers.browser.MainActivity" tools:showIn="@layout/activity_main"> <EditText android:id="@+id/editText" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignBottom="@+id/b1" android:layout_alignParentLeft="true" android:hint="Enter URL ex-www.javapapers.com" /> <Button android:id="@+id/b1" android:layout_width="50dp" android:layout_height="wrap_content" android:layout_alignParentRight="true" android:layout_alignParentTop="true" android:background="@drawable/forward" android:onClick="click" /> <WebView android:id="@+id/webView1" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_below="@+id/editText" android:layout_centerHorizontal="true" /> <ProgressBar android:id="@+id/progressBar" style="?android:attr/progressBarStyleLarge" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_centerVertical="true" /> </RelativeLayout>
Here is the description about the methods and classes used:-
package com.javapapers.android.browser; import android.graphics.Bitmap; import android.os.Bundle; import android.support.design.widget.FloatingActionButton; import android.support.design.widget.Snackbar; import android.support.v7.app.AppCompatActivity; import android.support.v7.widget.Toolbar; import android.view.KeyEvent; import android.view.View; import android.view.Menu; import android.view.MenuItem; import android.webkit.WebSettings; import android.webkit.WebView; import android.webkit.WebViewClient; import android.widget.Button; import android.widget.EditText; import android.widget.ProgressBar; import android.widget.Toast; public class MainActivity extends AppCompatActivity { Button b1; String link; WebView webView; EditText editText; ProgressBar progressBar; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar); setSupportActionBar(toolbar); b1 = (Button) findViewById(R.id.b1); webView = (WebView) findViewById(R.id.webView1); progressBar = (ProgressBar) findViewById(R.id.progressBar); progressBar.setVisibility(View.GONE); editText = (EditText) findViewById(R.id.editText); } @Override protected void onStart() { super.onStart(); home(); } public void click(View view) { link = "http://" + editText.getText().toString(); loadLink(); } @Override public boolean onCreateOptionsMenu(Menu menu) { getMenuInflater().inflate(R.menu.menu_main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { int id = item.getItemId(); if (id == R.id.action_file) { loadLocalPage(); } if (id == R.id.action_Home) { home(); } if (id == R.id.action_refresh) { refresh(); } return super.onOptionsItemSelected(item); } public void loadLink() { WebSettings webSetting = webView.getSettings(); webSetting.setBuiltInZoomControls(true); webView.setWebViewClient(new WebViewClient()); webSetting.setJavaScriptEnabled(true); } public void loadLocalPage() { WebSettings webSetting = webView.getSettings(); webSetting.setBuiltInZoomControls(true); webView.setWebViewClient(new WebViewClient()); webView.loadUrl("file:///android_asset/webpage.html"); } public void refresh() { loadLink(); } public void home() { WebSettings webSetting = webView.getSettings(); webSetting.setBuiltInZoomControls(true); webSetting.setJavaScriptEnabled(true); webView.setWebViewClient(new WebViewClient()); webView.loadUrl("https://javapapers.com"); } @Override public boolean onKeyDown(int keyCode, KeyEvent event) { if ((keyCode == KeyEvent.KEYCODE_BACK) && webView.canGoBack()) { webView.goBack(); return true; } return super.onKeyDown(keyCode, event); } public class WebViewClient extends android.webkit.WebViewClient { @Override public void onPageStarted(WebView view, String url, Bitmap favicon) { // TODO Auto-generated method stub super.onPageStarted(view, url, favicon); progressBar.setVisibility(View.VISIBLE); } @Override public boolean shouldOverrideUrlLoading(WebView view, String url) { // TODO Auto-generated method stub view.loadUrl(url); return true; } @Override public void onPageFinished(WebView view, String url) { // TODO Auto-generated method stub super.onPageFinished(view, url); progressBar.setVisibility(View.GONE); } } }
Finally our Android In-App Browser using WebView App is ready.
After the launch of app, home page loads in webview:
Opening a url:
Opening locally saved webpage:-
Android In-APP Browser Project Source