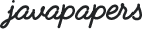
This Android tutorial is about refreshing a ListView by swiping down. It will enable the user to refresh the Android ListView in an Android application by providing a good user experience.
Earlier I published a tutorial to build a Todo list APP in Android and this kind of swipe to refresh feature will be comfortable for the user to refresh the listed items. This feature can also be used in the Android cards listview.
For swipe down refresh first you need to put your ListView inside <android.support.v4.widget.SwipeRefreshLayout>
layout. It will link the Swipe down refresh function to ListView
.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context="com.javapapers.android.swipe.MainActivity"> <android.support.v4.widget.SwipeRefreshLayout android:id="@+id/pullToRefresh" android:layout_width="match_parent" android:layout_height="wrap_content"> <ListView android:id="@+id/mobile_lis" android:layout_width="match_parent" android:layout_height="wrap_content"> </ListView> </android.support.v4.widget.SwipeRefreshLayout> </LinearLayout>
Make a layout file activity_listview.xml
inside your “main/res/layout” folder and put a TextView
inside it. This layout will be used in the ArrayAdapter
. This is how your single list item will look like.This layout will use again and again by the adapter to show all list items in the ListView
.
<?xml version="1.0" encoding="utf-8"?> <!-- Single List Item Design --> <TextView xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/label" android:layout_width="fill_parent" android:layout_height="fill_parent" android:padding="10dp" android:textSize="16sp" android:textStyle="bold" > </TextView>
Then in your main Android activity file just make an object of SwipeRefreshLayout, ArrayAdapter
and ListView
corresponding to their id’s and for ArrayAdapter
use the constructor ArrayAdapter(Context context, int resource, T[] objects)
.
addContent()
is a custom method, this is used to to create a new ArrayList()
and to pre add some items in the ListView
. Adding items in ArrayList()
is done by YourArrayListName.add("Item to add")
function so it will add the item to the array then we pass this array to the ArrayAdapter
to make a list of it’s content .Then set the adapter to the ListView by setAdapter()
. Then put a listener OnRefreshListener
on SwipeRefreshLayout
object. onRefresh()
is the function of the listener OnRefreshListener
it will get trigger when user refreshes the layout(i.e On swipe down). Now, we have to tell the onRefresh()
function what it has to do after it has refreshed the ListView
once.
package com.javapapers.android.swipe; import android.support.v4.widget.SwipeRefreshLayout; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.widget.ArrayAdapter; import android.widget.ListView; import android.widget.Toast; import java.util.ArrayList; public class MainActivity extends AppCompatActivity { SwipeRefreshLayout pullToRefresh; ArrayList menu; ArrayAdapter adapter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); pullToRefresh = (SwipeRefreshLayout) findViewById(R.id.pullToRefresh); addContent(); adapter = new ArrayAdapter(this, R.layout.activity_listview, menu); ListView listView = (ListView) findViewById(R.id.mobile_lis); listView.setAdapter(adapter); //setting an setOnRefreshListener on the SwipeDownLayout pullToRefresh.setOnRefreshListener(new SwipeRefreshLayout.OnRefreshListener() { int Refreshcounter = 1; //Counting how many times user have refreshed the layout @Override public void onRefresh() { //Here you can update your data from internet or from local SQLite data if (Refreshcounter == 1) { menu.add("Blackberry"); } if (Refreshcounter == 2) { menu.add("WebOS"); } if (Refreshcounter == 3) { menu.add("Ubuntu"); } if (Refreshcounter == 4) { menu.add("Windows7"); } if (Refreshcounter == 5) { menu.add("Max OS X"); } if (Refreshcounter > 5) { Toast.makeText(MainActivity.this, "No more data to load!!", Toast.LENGTH_SHORT).show(); } Refreshcounter = Refreshcounter + 1; adapter.notifyDataSetChanged(); pullToRefresh.setRefreshing(false); } }); } //Function to add Item in the list public void addContent() { menu = new ArrayList(); menu.add("Android"); menu.add("IPhone"); menu.add("WindowsMobile"); } }
We are using the function notifyDataSetChanged()
of the class adapter
to notify the adapter that data has been change and using the function setRefreshing()
of class SwipeRefreshLayout
which takes true
or false
as an argument. We are passing false
as the argument because we need to stop the refresh after one successful refresh.
Reference: Android developer document for swipe to refresh
Comments are closed for "Android Swipe Down to Refresh a ListView".
Can you explain the same a bit more.