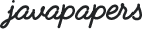
This tutorial is to learn how to show the current location on map in an Android application using Google Maps API. Previously we have seen tutorials to get the current location using the different location providers. If you are looking to just get the latitude and longitude of a location, the refer get current location using Android Fused location provider tutorial.
In this tutorial we will use the location manager to get the best provider for the device and use it to get the current location. Then the current location will be shown in the Google map using a marker.
We need API Key to access the Google service for displaying the maps. Following are the steps to get the API Key.
keytool -list -v -keystore "C:\Users\\Laptop\\.android\debug.keystore" -alias androiddebugkey -storepass android -keypass android
We need SHA1 fingerprint to bind the system which will access the Google API. Run the above command from command line and grab the SHA1 value from the output. Keytool is a tool available with Java JDK.
Login to the Google Developers Console and create a project. Then click into the project and click Credentials as shown below.
Then click create new Key and enter the SHA1 fingerprint value followed by a semicolon and then the Android application package.
On click of create we will get the Google API Key.
We need to set access permissions in the AndroidManifest file and the Google Maps API key should be specified in the meta-data.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.javapapers.currentlocationinmap"> <permission android:name="com.javapapers.currentlocationinmap.permission.MAPS_RECEIVE" android:protectionLevel="signature" /> <uses-permission android:name="com.javapapers.currentlocationinmap.permission.MAPS_RECEIVE" /> <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" /> <uses-permission android:name="com.google.android.providers.gsf.permission.READ_GSERVICES" /> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> <uses-feature android:glEsVersion="0x00020000" android:required="true" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme"> <activity android:name=".MyActivity" android:label="@string/app_name"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <meta-data android:name="com.google.android.gms.version" android:value="@integer/google_play_services_version" /> <meta-data android:name="com.google.android.maps.v2.API_KEY" android:value="AIzaS33yBBDxSDFsdf33BK-7HCasdfSADFfa72wJnKLZvq6A" /> </application> </manifest>
First we check if the GooglePlayServices is available. Add Google Play Services as a dependency in project. We use SupportMapFragment
and get map. Then use the LocationManager
to get the best provider and use it to get the last known location. We show markers in Google maps to point the current location.
package com.javapapers.currentlocationinmap; import android.location.Criteria; import android.location.Location; import android.location.LocationListener; import android.location.LocationManager; import android.os.Bundle; import android.support.v4.app.FragmentActivity; import android.widget.TextView; import com.google.android.gms.common.ConnectionResult; import com.google.android.gms.common.GooglePlayServicesUtil; import com.google.android.gms.maps.CameraUpdateFactory; import com.google.android.gms.maps.GoogleMap; import com.google.android.gms.maps.SupportMapFragment; import com.google.android.gms.maps.model.LatLng; import com.google.android.gms.maps.model.MarkerOptions; public class MyActivity extends FragmentActivity implements LocationListener { GoogleMap googleMap; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); //show error dialog if GoolglePlayServices not available if (!isGooglePlayServicesAvailable()) { finish(); } setContentView(R.layout.activity_my); SupportMapFragment supportMapFragment = (SupportMapFragment) getSupportFragmentManager().findFragmentById(R.id.googleMap); googleMap = supportMapFragment.getMap(); googleMap.setMyLocationEnabled(true); LocationManager locationManager = (LocationManager) getSystemService(LOCATION_SERVICE); Criteria criteria = new Criteria(); String bestProvider = locationManager.getBestProvider(criteria, true); Location location = locationManager.getLastKnownLocation(bestProvider); if (location != null) { onLocationChanged(location); } locationManager.requestLocationUpdates(bestProvider, 20000, 0, this); } @Override public void onLocationChanged(Location location) { TextView locationTv = (TextView) findViewById(R.id.latlongLocation); double latitude = location.getLatitude(); double longitude = location.getLongitude(); LatLng latLng = new LatLng(latitude, longitude); googleMap.addMarker(new MarkerOptions().position(latLng)); googleMap.moveCamera(CameraUpdateFactory.newLatLng(latLng)); googleMap.animateCamera(CameraUpdateFactory.zoomTo(15)); locationTv.setText("Latitude:" + latitude + ", Longitude:" + longitude); } @Override public void onProviderDisabled(String provider) { // TODO Auto-generated method stub } @Override public void onProviderEnabled(String provider) { // TODO Auto-generated method stub } @Override public void onStatusChanged(String provider, int status, Bundle extras) { // TODO Auto-generated method stub } private boolean isGooglePlayServicesAvailable() { int status = GooglePlayServicesUtil.isGooglePlayServicesAvailable(this); if (ConnectionResult.SUCCESS == status) { return true; } else { GooglePlayServicesUtil.getErrorDialog(status, this, 0).show(); return false; } } }
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <fragment android:id="@+id/googleMap" android:layout_width="match_parent" android:layout_height="match_parent" class="com.google.android.gms.maps.SupportMapFragment" android:layout_above="@+id/latlongLocation" /> <TextView android:id="@+id/latlongLocation" android:layout_width="fill_parent" android:layout_height="wrap_content" android:gravity="bottom" android:layout_alignParentBottom="true" android:background="#ff058fff" android:paddingTop="5dp" android:paddingBottom="5dp" android:textColor="#ffffffff" android:paddingLeft="5dp" android:paddingRight="5dp" /> </RelativeLayout>
Comments are closed for "Android Show Current Location on Map using Google Maps API".
[…] Show current location on map using Google Maps API. […]
Sir I’m your fan,Best tutorial on web for java and Android.Thank you sir
Thanks for the tutorial, but what about getting the address of the location (street, postal code, etc) ?
I ask this because geocoder might not be installed on the device so what explained in the google doc would not work.
@Marco, use this https://javapapers.com/android/android-get-address-with-street-name-city-for-location-with-geocoding/ tutorial to get the address of the location.
Hi, I was wondering if it is possible for a video to play when I am in a particular location from my phone KitKat 4.4 Thanks
thank you so much it helped lot
Hello sir, i followed your tutorial at first everythig worked, but now i am not getting the location ballon eventhough it is displaying my present location in blue circle, and the textview is not showing my co-ordinates.
Hello Joe,
Excellent tutorial!
Could you shed some light on how to clear the old markers, when the location is updated.
Thanks,
Praty
Thank you sir for this post
This post gave me much knowledge about Google Maps usage
So Simple and useful really handy
thanks man
My application is crashing while debugging in my mobile. am getting the error UNFORTUNATELY YOUR APPLICATION HAS CLOSED on opening the app. I copied the same code which was given above and my .java and .xml files show no errors.
if i want to display additional locations in addition to my location, how shall i go ahead
Sir i copied your project and tried it i have to pass my own latitude and longitude from ddms or else it will not work and also the position point is not showing
Dear sir
i used your application Android Show Current Location on Map using Google Maps API map is not showing. so please help me
sir i used your tutorial but it is showing only longitude and latitude.MAp is not showing please help
Dear Sir,
I am able to view the current location but I’m able to view the map. I’m getting the following message in my log.
D/WifiService﹕ releaseWifiLockLocked: WifiLock{NlpWifiLock type=2 binder=android.os.BinderProxy@1e4bcc8}
I am using same code as you have written in this article. But onLocationChanged(Location location) method called only once at beginning.
I want to move my location on map whenever I change my location. Do I need to add any extra things here. ?
woww… Ii works successfully..
thaks a lot… sr..
HAPPY DEPAWLI….