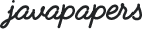
In this tutorial i will walk you through to create an android application demonstrating the use of proximity sensor. It is a sensor which detects the presence of an object/person in vicinity of the devices’ sensor.
Proximity sensors are used in many applications but it is most widely used in Smartphones. Most of the modern android devices comes with an inbuilt IR-based proximity sensor.
To built this example Android app we will use SensorManager and Sensor classes from the Android API. In this application we will display “NEAR” when an object is close to the device and display “AWAY” when the object is moved away. This is how we will demonstrate the use of Android proximity sensor.
Then, we will also see how to switch screen off when something is near the proximity sensor by making simple changes in the code. We will use PowerManager and WindowManager classes for this purpose.
We need to give uses-permission for Proximity sensor, other than that the AndroidManifest.xml is pretty standard one.
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.javapapers.android.proximity"> <uses-permission android:name="android.hardware.sensor.proximity"/> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
activity_main.xml
The Android Activity is designed to be simple. We have two TextView. One will display “No Proximity Sensor!” if application don’t find proximity sensor on the Android device. The other one will display “away” if there is no object in the vicinity of the device and the text will change to “near” if any object comes close to the Android device.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:gravity="center" android:orientation="vertical"> <TextView android:id="@+id/proximitySensor" android:layout_width="fill_parent" android:layout_height="wrap_content" /> <TextView android:id="@+id/data" android:layout_width="fill_parent" android:layout_height="wrap_content" android:textSize="50dp" /> </LinearLayout>
MainActivity.java
Here SensorManager, Sensor classes and SensorEventListener interface is used, which provides two callback methods to get information when sensor values (x,y and z) change or sensor accuracy changes.
Firstly, i have used myProximitySensor to check availability of proximity Sensor on the Android device. Then SensorEventListener is used which provides provides two callback methods to get information when sensor values (x,y and z) change or sensor accuracy changes.
package com.javapapers.android.proximity; import android.app.Activity; import android.content.Context; import android.hardware.Sensor; import android.hardware.SensorEvent; import android.hardware.SensorEventListener; import android.hardware.SensorManager; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.widget.TextView; public class MainActivity extends AppCompatActivity { TextView ProximitySensor, data; SensorManager mySensorManager; Sensor myProximitySensor; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); ProximitySensor = (TextView) findViewById(R.id.proximitySensor); data = (TextView) findViewById(R.id.data); mySensorManager = (SensorManager) getSystemService( Context.SENSOR_SERVICE); myProximitySensor = mySensorManager.getDefaultSensor( Sensor.TYPE_PROXIMITY); if (myProximitySensor == null) { ProximitySensor.setText("No Proximity Sensor!"); } else { mySensorManager.registerListener(proximitySensorEventListener, myProximitySensor, SensorManager.SENSOR_DELAY_NORMAL); } } SensorEventListener proximitySensorEventListener = new SensorEventListener() { @Override public void onAccuracyChanged(Sensor sensor, int accuracy) { // TODO Auto-generated method stub } @Override public void onSensorChanged(SensorEvent event) { // TODO Auto-generated method stub if (event.sensor.getType() == Sensor.TYPE_PROXIMITY) { if (event.values[0] == 0) { data.setText("Near"); } else { data.setText("Away"); } } } }; }
When there is no object near the Android device:
When an object comes close to Android device, the text on the screen changes to:
Now we will see one more application of proximity sensor. We will make our example application to make screen go off when something is near the proximity sensor. We just need to make the following changes in onSensorChanged method in MainActivity.java file.
public void onSensorChanged(SensorEvent event) { WindowManager.LayoutParams params = this.getWindow().getAttributes(); if(event.sensor.getType()==Sensor.TYPE_PROXIMITY){ if(event.values[0]==0){ params.flags |= WindowManager.LayoutParams.FLAG_KEEP_SCREEN_ON; params.screenBrightness = 0; getWindow().setAttributes(params); } else{ params.flags |= WindowManager.LayoutParams.FLAG_KEEP_SCREEN_ON; params.screenBrightness = -1f; getWindow().setAttributes(params); } } }
Android Proximity Sensor Example App
Comments are closed for "Android Proximity Sensor Example App".
Nice one again from you. Thank you.
I started blog reading with your blog, today I am reading a lot of blogs from other bloggers and books. Hope I could meet you someday :)