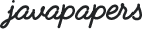
In this Android tutorial is I will walk you through to create a minimal image slider. What prompted me to write this tutorial is, when I did a recce on this topic on various tutorial sites all I found is complicated, elongated bunch of crap. Creating a image slider in an Android application is a simple task and that need not be complicated with hundreds of lines of code.
A minimal Android image slider requires just a single class and around fifty lines of code. If you need to make the image loading as an efficient process then you need to add few more lines to it. But what I found is hundreds of lines of code for just a primitive slider. In this tutorial we will see how to create a minimal image slider. In the next tutorial we will see how to load the images efficiently.
Out example image slider Android application will have one activity. We will use Fragment and ViewPager to display the images. I have added some sample images to “drawable” folder and these images will be loaded into image slider.
package com.javapapers.android.swipeimageslider; import android.os.Bundle; import android.support.v4.app.Fragment; import android.support.v4.app.FragmentActivity; import android.support.v4.app.FragmentManager; import android.support.v4.app.FragmentPagerAdapter; import android.support.v4.view.ViewPager; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.ImageView; public class MainActivity extends FragmentActivity { static final int NUM_ITEMS = 6; ImageFragmentPagerAdapter imageFragmentPagerAdapter; ViewPager viewPager; public static final String[] IMAGE_NAME = {"eagle", "horse", "bonobo", "wolf", "owl", "bear",}; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.fragment_pager); imageFragmentPagerAdapter = new ImageFragmentPagerAdapter(getSupportFragmentManager()); viewPager = (ViewPager) findViewById(R.id.pager); viewPager.setAdapter(imageFragmentPagerAdapter); } public static class ImageFragmentPagerAdapter extends FragmentPagerAdapter { public ImageFragmentPagerAdapter(FragmentManager fm) { super(fm); } @Override public int getCount() { return NUM_ITEMS; } @Override public Fragment getItem(int position) { SwipeFragment fragment = new SwipeFragment(); return SwipeFragment.newInstance(position); } } public static class SwipeFragment extends Fragment { @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { View swipeView = inflater.inflate(R.layout.swipe_fragment, container, false); ImageView imageView = (ImageView) swipeView.findViewById(R.id.imageView); Bundle bundle = getArguments(); int position = bundle.getInt("position"); String imageFileName = IMAGE_NAME[position]; int imgResId = getResources().getIdentifier(imageFileName, "drawable", "com.javapapers.android.swipeimageslider"); imageView.setImageResource(imgResId); return swipeView; } static SwipeFragment newInstance(int position) { SwipeFragment swipeFragment = new SwipeFragment(); Bundle bundle = new Bundle(); bundle.putInt("position", position); swipeFragment.setArguments(bundle); return swipeFragment; } } }
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@android:color/black"> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/imageView" android:layout_gravity="center_horizontal" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" /> </RelativeLayout>
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:gravity="center_horizontal" android:layout_width="fill_parent" android:layout_height="fill_parent"> <android.support.v4.view.ViewPager android:id="@+id/pager" android:layout_width="wrap_content" android:layout_height="wrap_content"> </android.support.v4.view.ViewPager> </LinearLayout>
Comments are closed for "Android Image Slider Tutorial".
This is a crisp tutorial and very useful one, thanks. Video showing output is cool. Can you describe the tutorial content in the future videos?
Hi Joe,
Hope you’ll respond soon. I am looking for this.
How to put Image Strip at Bottom of screen for this same Example?
Hi Joe,
Thanks for the tutorial. Its nice and clean :)
Just wanted to ask, any specific reason for using FragmentPagerAdapter?
Thanks, its what we expect , when we search .. Code optimization, and less loc …
This is a good quick tutorial. I would suggest only one thing, not creating a static NUM_ITEMS. I’d rather use IMAGE_NAME.length so if I change the string array I don’t have to remember to change the static count too.
Thanks a lot mate! Easy, fast & fun.
It Works with Android 21.
where is adapter file
i have put java file code and xml file code. and also put images in drawable folder. when i run application it will give black screen in android emulator. Can you help me for that.
Hii Anju
Please change your MainActivity.java file line 55 “com.javapapers.android.swipeimageslider” with your respected package name. You will get your desired output.
Thank you. Nice piece of code. Id like to know how can i add next and back buttons events to fragmentactivity. Thank you
please add circle page indicator to this code and auto sliding and please send me that code
noob here..
imported this project code into eclipse…. nothing happens :(
what to do ?