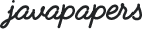
In this Android tutorial you will learn about Geocoding and how to find latitude/longitude for a give address location. In a previous tutorial we learnt about reverse geocoding to find address for a latitude/longitude. This tutorial is a reverse of that.
If you are looking for tutorial to find the current location then refer the get location using fused provider tutorial. In this tutorial we will get input address from the user and use Geocoder
API to get the Latitude and Longitude
Geocoding is converting the physical location address to latitude and longitude. Reverse geocoding is finding the location address based on the latitude and longitude. In this Android tutorial, we will be doing Geocoding to find the Latitude and Longitude of a given address location.
We need location access permissions. Following lines should be added to the AndroidManifest.xml.
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="android.permission.INTERNET" />
The complete AndroidManifest.xml
:
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.javapapers.androidgeocoding" > <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="android.permission.INTERNET" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name=".GeocodingActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Get user input for address location. Let us have an EditText
and get address input. Following is the activity file named activity_geocoding.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".GeocodingActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Enter Address" android:id="@+id/addressTV" android:textAppearance="?android:attr/textAppearanceMedium" android:layout_alignParentStart="true" /> <EditText android:layout_width="fill_parent" android:layout_height="wrap_content" android:id="@+id/addressET" android:layout_alignParentTop="true" android:layout_toEndOf="@+id/addressTV" android:singleLine="true" android:text="1600 Pennsylvania Ave NW Washington DC 20502" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Show Lat/Long" android:id="@+id/addressButton" android:layout_below="@+id/addressTV" android:layout_toEndOf="@+id/addressTV" android:layout_marginTop="50dp" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceLarge" android:text="" android:id="@+id/latLongTV" android:layout_centerVertical="true" android:layout_toEndOf="@+id/addressTV" /> </RelativeLayout>
Following is the Android Activity class GeocodingActivity
package com.javapapers.androidgeocoding; import android.app.Activity; import android.os.Bundle; import android.os.Handler; import android.os.Message; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; public class GeocodingActivity extends Activity { Button addressButton; TextView addressTV; TextView latLongTV; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_geocoding); addressTV = (TextView) findViewById(R.id.addressTV); latLongTV = (TextView) findViewById(R.id.latLongTV); addressButton = (Button) findViewById(R.id.addressButton); addressButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View arg0) { EditText editText = (EditText) findViewById(R.id.addressET); String address = editText.getText().toString(); GeocodingLocation locationAddress = new GeocodingLocation(); locationAddress.getAddressFromLocation(address, getApplicationContext(), new GeocoderHandler()); } }); } private class GeocoderHandler extends Handler { @Override public void handleMessage(Message message) { String locationAddress; switch (message.what) { case 1: Bundle bundle = message.getData(); locationAddress = bundle.getString("address"); break; default: locationAddress = null; } latLongTV.setText(locationAddress); } } }
We should run the external API access in a separate thread in order not to disturb the UI activity. We should instantiate the Geocoder
class and use the method getFromLocationName
to get the complete address which includes the latitude and longitude.
package com.javapapers.androidgeocoding; import android.content.Context; import android.location.Address; import android.location.Geocoder; import android.os.Bundle; import android.os.Handler; import android.os.Message; import android.util.Log; import java.io.IOException; import java.util.List; import java.util.Locale; public class GeocodingLocation { private static final String TAG = "GeocodingLocation"; public static void getAddressFromLocation(final String locationAddress, final Context context, final Handler handler) { Thread thread = new Thread() { @Override public void run() { Geocoder geocoder = new Geocoder(context, Locale.getDefault()); String result = null; try { List addressList = geocoder.getFromLocationName(locationAddress, 1); if (addressList != null && addressList.size() > 0) { Address address = addressList.get(0); StringBuilder sb = new StringBuilder(); sb.append(address.getLatitude()).append("\n"); sb.append(address.getLongitude()).append("\n"); result = sb.toString(); } } catch (IOException e) { Log.e(TAG, "Unable to connect to Geocoder", e); } finally { Message message = Message.obtain(); message.setTarget(handler); if (result != null) { message.what = 1; Bundle bundle = new Bundle(); result = "Address: " + locationAddress + "\n\nLatitude and Longitude :\n" + result; bundle.putString("address", result); message.setData(bundle); } else { message.what = 1; Bundle bundle = new Bundle(); result = "Address: " + locationAddress + "\n Unable to get Latitude and Longitude for this address location."; bundle.putString("address", result); message.setData(bundle); } message.sendToTarget(); } } }; thread.start(); } }
Latitude, Longitude: 38.898748, -77.037684 Location Address: 1600 Pennsylvania Ave NW Washington DC 20502 Latitude, Longitude: 34.101509, -118.32691 Location Address: Hollywood Blvd & Vine St Los Angeles CA 90028
Comments are closed for "Android Geocoding to Get Latitude Longitude for an Address".
how to show latitude and lang in androi please give your feedback in easy steps .
how to access lat and long via wifi connectivity
Hello Joe,
First of all, thanks a lot for time spent in this tuto.
I followed it and made some modifications, it perfect to me. I just have a doubt set latitude and longitude in differents fields.
In fact, what I am trying to do is modify the following code snippet. to get latitude and longitude and send to 2 different fields.
Address endereco = addressList.get(0);
StringBuilder sb = new StringBuilder();
sb.append(endereco.getLatitude()).append(“\n”);
sb.append(endereco.getLongitude()).append(“\n”);
result = sb.toString();
The code crashes and gives an Exception: Unable to connect to Geocoder, for some locations. It times out while trying to connect.
How to resolve that?
You need to set maxResult to 5 at least
e.g.
getFromLocationName(place, 5);