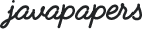
Firebase is a platform provided by Google to power up your mobile App. Firebase is packed with salient features like user authentication and cloud messaging. In this tutorial, i will demonstrate the use of authentication feature in Firebase.
I will build an android app and show how Firebase auth feature is implemented in android.
I will walk you through to build a sign up and a login activity. In sign up activity, we’ll make user enter email address and set password. We’ll also provide login option to the registered user. Register users will be directed to a new activity. This user activity will have a dummy text and a logout button. Button click will direct user to login activity.
Following image lists the module available in Firebase courtesy https://firebase.google.com/products/
I have tried to make things as simple as possible. You can use this in your Firebase based Android APP as base structure and can add or modify the feature according to your requirements.
Before we proceed, we need to link our app to Firebase. Follow these steps,
So, now we got our app linked to firebase, lets get started with code!
It is our sign up activity. It has two EditTexts, a Button and a TextView. Here, EditTexts are used to get email and password from user. An option is provided to log in for registered users. TextView is used for this purpose.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:gravity="center" tools:context="com.javapapers.firebaseauthdemo.MainActivity"> <EditText android:id="@+id/ETemail" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="15dp" android:hint="Email" android:inputType="textEmailAddress" /> <EditText android:id="@+id/ETpassword" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="15dp" android:hint="Password" android:inputType="numberPassword" /> <Button android:id="@+id/btnSignUp" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="15dp" android:text="Sign Up"/> <TextView android:id="@+id/TVSignIn" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Already have an account? Sign in Here" android:layout_margin="15dp" android:textSize="20dp"/> </LinearLayout>
Layout of login activity is similar to sign up activity.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:orientation="vertical"> <EditText android:id="@+id/loginEmail" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="15dp" android:hint="Email" android:inputType="textEmailAddress" /> <EditText android:id="@+id/loginpaswd" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="15dp" android:hint="Password" android:inputType="numberPassword"/> <Button android:id="@+id/btnLogIn" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="15dp" android:text="Login In" android:textSize="25dp" /> <TextView android:id="@+id/TVSignIn" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="15dp" android:text="New User? Register Here" android:textSize="20dp" /> </LinearLayout>
This activity contain a textview and a Button for logout. TextView has a dummy text.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_gravity="center" android:orientation="vertical" tools:context="com.javapapers.firebaseauthdemo.UserActivity"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_centerVertical="true" android:layout_gravity="center" android:gravity="center" android:text="Welcome!" android:textSize="40dp" /> <Button android:id="@+id/btnLogOut" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_margin="15dp" android:text="Logout" /> </RelativeLayout>
Here, we have two click listeners, one attached to button and other to textview. On button click, it is checked if any of the parameter empty .If TextView is clicked, then user will be directed to login activity. If that’s the case then error message is displayed. If both textviews has data, then createUserWithEmailAndPassword() method is invoked.
Inside createUserWithEmailAndPassword() method, task success is checked. If task is successful then user is directed to user activity. Sometimes user enter/mistype email(like no use of @ symbol) and password(like too short password), then app may crash. We don’t want that to happen. To avoid that, we’ll use getException() method and display it in a toast.
package com.javapapers.firebaseauthdemo; import android.content.Intent; import android.support.annotation.NonNull; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast; import com.google.android.gms.tasks.OnCompleteListener; import com.google.android.gms.tasks.Task; import com.google.firebase.auth.AuthResult; import com.google.firebase.auth.FirebaseAuth; public class MainActivity extends AppCompatActivity { public EditText emailId, passwd; Button btnSignUp; TextView signIn; FirebaseAuth firebaseAuth; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); firebaseAuth = FirebaseAuth.getInstance(); emailId = findViewById(R.id.ETemail); passwd = findViewById(R.id.ETpassword); btnSignUp = findViewById(R.id.btnSignUp); signIn = findViewById(R.id.TVSignIn); btnSignUp.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { String emailID = emailId.getText().toString(); String paswd = passwd.getText().toString(); if (emailID.isEmpty()) { emailId.setError("Provide your Email first!"); emailId.requestFocus(); } else if (paswd.isEmpty()) { passwd.setError("Set your password"); passwd.requestFocus(); } else if (emailID.isEmpty() && paswd.isEmpty()) { Toast.makeText(MainActivity.this, "Fields Empty!", Toast.LENGTH_SHORT).show(); } else if (!(emailID.isEmpty() && paswd.isEmpty())) { firebaseAuth.createUserWithEmailAndPassword(emailID, paswd).addOnCompleteListener(MainActivity.this, new OnCompleteListener() { @Override public void onComplete(@NonNull Task task) { if (!task.isSuccessful()) { Toast.makeText(MainActivity.this.getApplicationContext(), "SignUp unsuccessful: " + task.getException().getMessage(), Toast.LENGTH_SHORT).show(); } else { startActivity(new Intent(MainActivity.this, UserActivity.class)); } } }); } else { Toast.makeText(MainActivity.this, "Error", Toast.LENGTH_SHORT).show(); } } }); signIn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Intent I = new Intent(MainActivity.this, ActivityLogin.class); startActivity(I); } }); } }
Here, code is similar to that of MainActivity. Only major difference is use of signInWithEmailAndPassword() method in place of createUserWithEmailAndPassword().
package com.javapapers.firebaseauthdemo; import android.content.Intent; import android.support.annotation.NonNull; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast; import com.google.android.gms.tasks.OnCompleteListener; import com.google.android.gms.tasks.Task; import com.google.firebase.auth.AuthResult; import com.google.firebase.auth.FirebaseAuth; import com.google.firebase.auth.FirebaseUser; public class ActivityLogin extends AppCompatActivity { public EditText loginEmailId, logInpasswd; Button btnLogIn; TextView signup; FirebaseAuth firebaseAuth; private FirebaseAuth.AuthStateListener authStateListener; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_login); firebaseAuth = FirebaseAuth.getInstance(); loginEmailId = findViewById(R.id.loginEmail); logInpasswd = findViewById(R.id.loginpaswd); btnLogIn = findViewById(R.id.btnLogIn); signup = findViewById(R.id.TVSignIn); authStateListener = new FirebaseAuth.AuthStateListener() { @Override public void onAuthStateChanged(@NonNull FirebaseAuth firebaseAuth) { FirebaseUser user = firebaseAuth.getCurrentUser(); if (user != null) { Toast.makeText(ActivityLogin.this, "User logged in ", Toast.LENGTH_SHORT).show(); Intent I = new Intent(ActivityLogin.this, UserActivity.class); startActivity(I); } else { Toast.makeText(ActivityLogin.this, "Login to continue", Toast.LENGTH_SHORT).show(); } } }; signup.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Intent I = new Intent(ActivityLogin.this, MainActivity.class); startActivity(I); } }); btnLogIn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { String userEmail = loginEmailId.getText().toString(); String userPaswd = logInpasswd.getText().toString(); if (userEmail.isEmpty()) { loginEmailId.setError("Provide your Email first!"); loginEmailId.requestFocus(); } else if (userPaswd.isEmpty()) { logInpasswd.setError("Enter Password!"); logInpasswd.requestFocus(); } else if (userEmail.isEmpty() && userPaswd.isEmpty()) { Toast.makeText(ActivityLogin.this, "Fields Empty!", Toast.LENGTH_SHORT).show(); } else if (!(userEmail.isEmpty() && userPaswd.isEmpty())) { firebaseAuth.signInWithEmailAndPassword(userEmail, userPaswd).addOnCompleteListener(ActivityLogin.this, new OnCompleteListener() { @Override public void onComplete(@NonNull Task task) { if (!task.isSuccessful()) { Toast.makeText(ActivityLogin.this, "Not sucessfull", Toast.LENGTH_SHORT).show(); } else { startActivity(new Intent(ActivityLogin.this, UserActivity.class)); } } }); } else { Toast.makeText(ActivityLogin.this, "Error", Toast.LENGTH_SHORT).show(); } } }); } @Override protected void onStart() { super.onStart(); firebaseAuth.addAuthStateListener(authStateListener); } }
We need to make user exit his account on button click. We’ll add click listener to this button. signOut() method is used here.
package com.javapapers.firebaseauthdemo; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import com.google.firebase.auth.FirebaseAuth; public class UserActivity extends AppCompatActivity { Button btnLogOut; FirebaseAuth firebaseAuth; private FirebaseAuth.AuthStateListener authStateListener; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_user); btnLogOut = (Button) findViewById(R.id.btnLogOut); btnLogOut.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { FirebaseAuth.getInstance().signOut(); Intent I = new Intent(UserActivity.this, ActivityLogin.class); startActivity(I); } }); } }
Data created via the Android app can be viewed from Firebase console.
Android Firebase Auth APP Project
Comments are closed for "Android Firebase Authentication".
Very useful toutorial