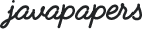
This Android tutorial is a a continuation of our ListView series. We will builld an example Android application to explain how to use the expandable ListView. ExpandableListView is an Android container available as part of the platform. It will be useful to display grouped set of items. On click of the group titles the child elements will be expanded.
As seen in the “Android ListView Custom Layout Tutorial” we can use a type of array adapter to control the data displayed in the expandable list view. We will use the BaseExpandableListAdapter to supply and control the data displayed in this tutorial.
Following example app is to display a list group with three categories. Each category will have five child items grouped under a title. On click on a title it will be expanded and its child item will be listed and on next click it will be collapsed.
We instantiated ExpandableListView and attach the adapter to it.
package com.javapapers.android.expandablelistview.app; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import android.app.Activity; import android.os.Bundle; import android.view.View; import android.widget.ExpandableListView; import android.widget.ExpandableListView.OnChildClickListener; import android.widget.ExpandableListView.OnGroupCollapseListener; import android.widget.ExpandableListView.OnGroupExpandListener; import android.widget.Toast; public class MainActivity extends Activity { ExpandableListView expandableListView; ExpandableListAdapter expandableListAdapter; ListexpandableListTitle; HashMap > expandableListDetail; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); expandableListView = (ExpandableListView) findViewById(R.id.expandableListView); expandableListDetail = ExpandableListDataPump.getData(); expandableListTitle = new ArrayList (expandableListDetail.keySet()); expandableListAdapter = new ExpandableListAdapter(this, expandableListTitle, expandableListDetail); expandableListView.setAdapter(expandableListAdapter); expandableListView.setOnGroupExpandListener(new OnGroupExpandListener() { @Override public void onGroupExpand(int groupPosition) { Toast.makeText(getApplicationContext(), expandableListTitle.get(groupPosition) + " List Expanded.", Toast.LENGTH_SHORT).show(); } }); expandableListView.setOnGroupCollapseListener(new OnGroupCollapseListener() { @Override public void onGroupCollapse(int groupPosition) { Toast.makeText(getApplicationContext(), expandableListTitle.get(groupPosition) + " List Collapsed.", Toast.LENGTH_SHORT).show(); } }); expandableListView.setOnChildClickListener(new OnChildClickListener() { @Override public boolean onChildClick(ExpandableListView parent, View v, int groupPosition, int childPosition, long id) { Toast.makeText( getApplicationContext(), expandableListTitle.get(groupPosition) + " -> " + expandableListDetail.get( expandableListTitle.get(groupPosition)).get( childPosition), Toast.LENGTH_SHORT ) .show(); return false; } }); } }
This is a BaseExpandableListAdapter which supplies and manages the underlying data for the ExpandableListView.
package com.javapapers.android.expandablelistview.app; import java.util.ArrayList; import java.util.HashMap; import java.util.List; public class ExpandableListDataPump { public static HashMap> getData() { HashMap > expandableListDetail = new HashMap >(); List technology = new ArrayList (); technology.add("Beats sued for noise-cancelling tech"); technology.add("Wikipedia blocks US Congress edits"); technology.add("Google quizzed over deleted links"); technology.add("Nasa seeks aid with Earth-Mars links"); technology.add("The Good, the Bad and the Ugly"); List entertainment = new ArrayList (); entertainment.add("Goldfinch novel set for big screen"); entertainment.add("Anderson stellar in Streetcar"); entertainment.add("Ronstadt receives White House honour"); entertainment.add("Toronto to open with The Judge"); entertainment.add("British dancer return from Russia"); List science = new ArrayList (); science.add("Eggshell may act like sunblock"); science.add("Brain hub predicts negative events"); science.add("California hit by raging wildfires"); science.add("Rosetta's comet seen in close-up"); science.add("Secret of sandstone shapes revealed"); expandableListDetail.put("TECHNOLOGY NEWS", technology); expandableListDetail.put("ENTERTAINMENT NEWS", entertainment); expandableListDetail.put("SCIENCE & ENVIRONMENT NEWS", science); return expandableListDetail; } }
Main activity layout which contains the ExpandableListView
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context="com.javapapers.android.expandablelistview.app.MainActivity"> <ExpandableListView android:id="@+id/expandableListView" android:layout_height="match_parent" android:layout_width="match_parent" android:indicatorLeft="?android:attr/expandableListPreferredItemIndicatorLeft" android:divider="#A4C739" android:dividerHeight="0.5dp" /> </RelativeLayout>
Expandable list group item.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/listTitle" android:layout_width="fill_parent" android:layout_height="wrap_content" android:paddingLeft="?android:attr/expandableListPreferredItemPaddingLeft" android:textColor="#A4C739" android:paddingTop="10dp" android:paddingBottom="10dp" /> </LinearLayout>
Expanded list items layout.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="wrap_content"> <TextView android:id="@+id/expandedListItem" android:layout_width="fill_parent" android:layout_height="wrap_content" android:paddingLeft="?android:attr/expandableListPreferredChildPaddingLeft" android:paddingTop="10dp" android:paddingBottom="10dp" /> </LinearLayout>
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.javapapers.android.expandablelistview.app" > <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.javapapers.android.expandablelistview.app.MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Download ExpandableListView Application Source
Comments are closed for "Android Expandable ListView".
Missing ExpandableListAdapter.java file? Just have ExpandableListDataPump.java
Thank You Sir. How can i use intent to open new page when i click on child of a expandable list view ?
You have left out ExpandableListAdapter.java
Also there are some typos in the other code that cause compiler errors, such as
HashMap> expandableListDetail;
HashMap> expandableListDetail = new HashMap>();
Hi – great tutorial, I’ve hacked it successfully for my app.
Question: I have a lot of parent groups (about 200), each with up to 12 children. When I click on a parent, it opens up to show the children, but instantly scrolls the next parent to the top of the visible area on the screen (and you have to thumb down to show the expanded children). Have you encountered this, and can it be fixed?
Iain
Found a fix for my above issue:
http://stackoverflow.com/questions/14479078/smoothscrolltopositionfromtop-is-not-always-working-like-it-should