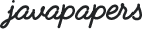
In this lesson on Android notifications, we will take a look at how to create and manage bundled notifications. Earlier we saw an introductory tutorial on Android notifications.
A notification is an alert or a simple message shown to the user in the notification panel of an Android device. This alert is separate from the normal interface of an app and is used to alert the user about an event in or outside the app.
We will start by creating simple notifications using a very simple API NotificationCompat.
Every notification consists of few parts, mainly:
With above data present, we can create an Androi notification using NotificationCompat.Builder and send it to the UI, like:
private void createNotification() { NotificationCompat.Builder builder = new NotificationCompat.Builder(this, "MY_CHANNEL_ID"); //set small icon and content title builder .setSmallIcon(R.drawable.ic_launcher_background) .setContentTitle("J.Papers"); String text = "Hey, how are the notifications going?"; //set supporting text builder.setContentText(text); Notification notification = builder.build(); //send notification NotificationManagerCompat.from(this) .notify(notificationId++, notification); }
Tapping on this notification does nothing! Well, this was because we didn’t specify the Intent of this notification.
We need a content intent which is a PendingIntent. A content intent can be set as:
Intent intent = new Intent( this, SecondActivity.class); PendingIntent pendingIntent = PendingIntent.getService( this, 0, intent, PendingIntent.FLAG_CANCEL_CURRENT); builder.setContentIntent(pendingIntent);
Now that a PendingIntent is added, once we tap on the notification, an activity starts. Once we call createNotification() method in my onCreate(…), we can see the following notification when the app starts:
That looks good! But what if we start our application again and again? We will just see the same notification appear again and again. Well, this is a general problem which used to happen with some chat apps a lot. What is the solution for this? Android N brought a smart solution known as Bundled Notifications. Before moving onto that, let us see what all code of MainActivity looks like:
package com.javapapers.android.bundlednotifications; import android.app.Notification; import android.app.PendingIntent; import android.content.Intent; import android.os.Bundle; import android.support.v4.app.NotificationCompat; import android.support.v4.app.NotificationManagerCompat; import android.support.v7.app.AppCompatActivity; import android.view.View; public class MainActivity extends AppCompatActivity { private int notificationId = 1; private String groupKey = "JavaPapers"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } public void createNotification(View view) { NotificationCompat.Builder builder = new NotificationCompat.Builder(this, groupKey); //set small icon and content title builder .setSmallIcon(R.drawable.ic_launcher_background) .setContentTitle("J.Papers"); String text = "Hey, how are the notifications going?"; //set supporting text builder.setContentText(text); Intent intent = new Intent( this, SecondActivity.class); PendingIntent pendingIntent = PendingIntent.getService( this, 0, intent, PendingIntent.FLAG_CANCEL_CURRENT); builder.setContentIntent(pendingIntent); Notification notification = builder.build(); //send notification NotificationManagerCompat.from(this) .notify(notificationId++, notification); } }
By bundling similar notifications, we can make sure that redundant data is not shown to the user. Similar notifications can be grouped like:
Bundling similar notifications is easy. Just provide them with a group key and Android will identify if it should be put in an existing group or create a new one!
Let’s see how can we make group notifications appear. Just add a new call:
String text = "Hey, how are the notifications going?"; //set supporting text builder.setContentText(text); Intent intent = new Intent( this, SecondActivity.class); PendingIntent pendingIntent = PendingIntent.getService( this, 0, intent, PendingIntent.FLAG_CANCEL_CURRENT); builder.setContentIntent(pendingIntent); builder.setStyle(new NotificationCompat.InboxStyle() .addLine("Hello") .addLine("Hi")) .setGroup(groupKey) .setGroupSummary(true); Notification notification = builder.build();
groupKey is a simple String defined in our Activity. Now, if we run our app, we can see the same number of notifications as shown:
Notice the difference? First visible notification is actually an expanded version of the second visible notification. In the code above, we set multiple notification lines and set group summary as true.
It is worth noticing that this summary notification only works after Android API 24.
Bundled notifications are very helpful and supported as well. Let’s summarise this in few points:
In this blog post, we saw how Bundled notifications help us to save more space in notification panel and present to user a very sleek and summarized user interface.
Find all project source code here and share your feedback in the comments below.