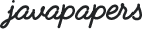
When I say life cycle, I can hear you murmur “Oh no not again, how many life cycles I have to deal with”! In real world everything has life cycle, then why not in programming, after all, software is all about mimicking real life. In a previous article I discussed about methods used for session tracking.
Frog Life Cycle
Now we are going to dive deep into it.
Just to recap, session is a conversion between a server and a client. An elite way to manage the session in servlets is to use API. Any web server supporting servlets will eventually have to implement the servlet API. It may or may not provide with more features of luxury but the minimum is guaranteed. Servlet specification ensures that, the minimum features provided make the session management job easier. Servlet API will use one of the underlying traditional mechanisms like cookies, URL rewriting, but that will happen behind the scenes and you need not worry about it!
Every request is associated with an HttpSession object. It can be retrieved using getSession(boolean create) available in HttpServletRequest. It returns the current HttpSession associated with this request or, if there is no current session and create is true, and then returns a new session. A session can be uniquely identified using a unique identifier assigned to this session, which is called session id. getId() gives you the session id as String.
isNew() will be handy in quite a lot of situations. It returns true if the client does not know about the session or if the client chooses not to join the session. getCreationTime() returns the time when this session was created. getLastAccessedTime() returns the last time the client sent a request associated with this session.
Once you have got access to a session object, it can be used as a HashTable to store and retrieve values. It can be used to transport data between requests for the same user and session. setAttribute(String name, Object value) adds an object to the session, using the name specified. Primitive data types cannot be bound to the session.
An important note to you, session is not a bullock cart. It should be used sparingly for light weight objects. If you are in a situation where you have to store heavy weight objects in session, then you are in for a toss. Now it’s time to consult a software doctor. Your software design is having a big hole in it. HttpSession should be used for session management and not as a database.
Follow a proper naming convention to store data in session. Because it will overwrite the existing object if the name is same. One more thing to note is your object needs to implement Serializable interface if you are going to store it in session and carry it over across different web servers.
getAttribute(String name) returns the object bound with the specified name in this session. Be careful while using this, most programmers fell into a deeply dug pit NullPointerException. Because it returns null if no object is bound under the name. Always ensure to handle null. Then, removeAttribute(String name) removes the object bound with the specified name from the session. Note a point; be cautious not to expose the session id to the user explicitly. It can be used to breach into another client’s session unethically.
By default every web server will have a configuration set for expiry of session objects. Generally it will be some X seconds of inactivity. That is when the user has not sent any request to the server for the past X seconds then the session will expire. What do I mean by expire here. Will the browser blowup into colorful pieces? When a session expires, the HttpSession object and all the data it contains will be removed from the system. When the user sends a request after the session has expired, server will treat it as a new user and create a new session.
Apart from that automatic expiry, it can also be invalidated by the user explicitly. HttpSession provides a method invalidate() this unbinds the object that is bound to it. Mostly this is used at logout. Or the application can have an absurd logic like after the user logins he can use the application for only 30 minutes. Then he will be forced out. In such scenario you can use getCreationTime().
Generally session object is not immortal because of the default configuration by the web server. Mostly these features are left to the imagination of web server implementers. If you take Apache Tomcat 5.5, there is an attribute maxInactiveInterval. A negative value for this will result in sessions never timing out and will be handy in many situations.
Long live the sessions!
import java.io.IOException; import java.io.PrintWriter; import java.util.Date; import java.util.Enumeration; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.http.HttpSession; public class SessionExample extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html"); PrintWriter out = response.getWriter(); // getting current HttpSession associated with this request or, if there // is no current session and create is true, returns a new session. HttpSession session = request.getSession(true); // Calculating Hit Count Integer count = (Integer) session .getAttribute("SessionExample.HitCount"); if (count == null) count = new Integer(1); else count = new Integer(count.intValue() + 1); session.setAttribute("SessionExample.HitCount", count); out.println("<HTML><HEAD><TITLE>SessionExample</TITLE></HEAD>"); out.println("<BODY><H1>Example session servlet to " + "demostrate session tracking and life cycle</H1>"); // Displaying the hit count out.println("Hit count for your current session is " + count); out.println("<H2>Some basic session information:</H2>"); out.println("Session ID: " + session.getId() + "</BR>"); out.println("Is it a new session: " + session.isNew() + "</BR>"); out.println("Session Creation time: " + session.getCreationTime()); out.println("(" + new Date(session.getCreationTime()) + ")</BR>"); out.println("Last accessed time: " + session.getLastAccessedTime()); out.println("(" + new Date(session.getLastAccessedTime()) + ")</BR>"); out.println("Max in active time interval: " + session.getMaxInactiveInterval() + "</BR>"); // Checks whether the requested session ID came in as a cookie out.println("Session ID came in as a cookie: " + request.isRequestedSessionIdFromCookie() + "</BR>"); out.println("<H2>Iteratively printing all the values " + "associated with the session:</H2>"); Enumeration names = session.getAttributeNames(); while (names.hasMoreElements()) { String name = (String) names.nextElement(); String value = session.getAttribute(name).toString(); out.println(name + " = " + value + "</BR>"); } out.println("</BODY></HTML>"); } }
Output of the example session servlet
Comments are closed for "Session Life Cycle".
I am a beginner to java & servlets. I went through your article fully. Its good. It is very helpful to me to understand the concepts. But i have a doubt.
***********
Integer count=(Integer)session.getAttribute(“SessionExample.HitCount”);
In this above line, what is “SessionExample.HitCount”? For you this question maybe simple. But i cant able to get the exact point of using that. Is it a field ID in a HTML plage?
***********
Enumeration names = session.getAttributeNames();
will return the session names. But you gave a string in .getAttribute() method. So, whenever a new person logged in the same name will be set huh?
************
while (names.hasMoreElements()) {
String name = (String) names.nextElement();
String value = session.getAttribute(name).toString();
out.println(name + ” = ” + value + “”);
}
Will the name variable get “SessionExample.HitCount” for every time this loop?
If i need to put new value means, do i need to set that value dynamically?
***********
Again, i tell you i am a noob in servlets. I seeking clear implementation about sessions. But no need to explain me. Just clear my quries. I ll make myself clear after that.
@ Vendhan,
SessionExample.HitCount is just another name of attribute (you may say variable) in session. Just be familiar with the signatures of these methods:
session.setAttribute(“NameHere”, valueHere);
session.getAttribute(“NameHere”);
And you wud not be bothered of what the name looks like.
***********
Enumeration names = session.getAttributeNames(); returns all the session attributes as a enum, and for the getAttribute String you are talking is just a name and it will print its corresponding value for every new user.
***********
the third question was not clear to me,
Comment if i answered your question fairly.
It is good for who learnig basic servlets and jsp
Nice Explanation
this is very wonderfull to study thanks for your article this is the is very original…
This is really good article to understand the basics.
Good ..but some places it is better to explain with small code of lines. like setting the values in session, and getting.
nice
good.
now , I like reading the English site , but my English is very poor,I am chinese , learning !
I am a beginner in servlets i want to know the differences between ServletContext and ServletConfig and where it is used exactly.
this is good and useful work. thank you
this is really great to understand…thank you…god bless ur work
really useful..thank you so much..
can you post some documentations on struts….
you have explained everything so nicely.
It is so much helpful for me.Thank you
nice example,bt one thing i was not clear is , when i run session example in IE , every time a new session is getting created,and tracks the data only of that particular session.once the session is closed, the data also getting lost.instead when i run that in firefox,everytime it was giving same session id, and showing previous session values also.
is it bcz of disable state of cookies in IE?
Really nice and helpful Blog.
Nice article on session
im beginner in j2ee concepts could you help to be a master in that
hi sir as we know that servlet context and config are interfaces…..so why do we create their objects…please help me out.
Its pleasure to tell you that this site really helpful to learn java concepts. you are rocking. thanks
realy its very usefull for fresher .
Specify parallel technologies to the servlet?
ok its is goood …please post in detail description…
nice job..
yes it had been very useful for me but sir i m programming on session and i have a doubt sir we can transfer the data from one page to another by using setAttribute and getAttribute methods but sir how can we bind an object into session and solve the problem of null pointer exception.sir could you please impact light on this.
thankyou so much…
hi sir
ur blog is awesome .. i understood many concepts. can u give a complete explanation of servlets. like about http request and response and many other things… only few topics are here. i like to completly understand servlets and jee cocepts..
if u think this is not possible can u reffer me book which can help me to understand servlets and jee topics clearly..
cookies based hit counter is really usefull
Hi i have one doubt
I am using the java struts1.2 web application server is jboss5 ,
I am getting the error while the user login and using the application suddenly new session get created so I loss all session data application throw an error didnt get the log for destroy of old session, but new onle get created.. how solve this
Thanks in advance
sir one request… its too technical. kindly describe in simple words. Thank u so much.
nice article joe, and I am having one doubt y do servlets don’t have constructor. Or if they r having where it vl be useful.??? Can u explain clearly???
Really good
sir please give the differences between
sendRedirect and forward?
Its here,
https://javapapers.com/jsp/difference-between-forward-and-sendredirect/
Nice explanation about session
hi,
i have a scenario in my application where in which I want to explicitly invalidate the session of a user after 12 minutes,if the user has logged in but is not using the application
my doubt is:
1.Is it fine if i will set the session’s max interval time as 12 minutes.But for active user also session is invalidated after 12 minutes and i want only session of inactive user to be invalidated
2.Shall i get the last accessed time and see if exceeds 12 mins and then invalidate
3.if i should follow above point-2,then my doubt is the session.getLastAccesed() returns ‘long’…and is that in ‘minutes’ or ‘seconds’
Please,post a code snippet showing to explicitly invalidate a session by seeting its lifespan also explicitly.
thanks
thanks a lot, sir
Thanks for detailing.
But I am unable to execute servlet file. Pleas help me. It says
File Not Found
Hi the thing you mentioned is the session attribute which has been set in a http session.
very good article.
Thank u very much.
Hi,
i want to write the code for disable the back button.
when , i log out in my application, if i click back button i don’t want to go back page.
So, how i have to write the code.
Can, any one help me. ? ? ?
Nice explanation
Hi,
This article is really helpful.
I’m using ninja kit for cross domain request.Somehow, my session object is not getting persisted in firefox browser. For every request there is new httpsession getting created. Same issue with safari 6. But this works fine with chrome.
Any idea ?
Great Work… Please keep them coming.
pls add struts2 and hibernate……….
Very Useful information for Java Web Application Beginners
please tell the difference between printwriter and System.out
it’s make things go easy ..nice article ..
mainly about the null pointer exception warning
Login.jsp
Login
Error In Login
logout.jsp
Login
am creating one login application with login and logout date and time but dis will no exist a have a error from that like:
java.sql.SQLException: ORA-01006: bind variable does not exist,,,, at server side but the value means datetime will stored.
Hi,
Read all your threads..was excellent ..even about session details..Actually i am doin a web application where i have show the username (session variable)..but the issue is when one user login the details in the session is correct but when concurrent user logs in it displays the new user instead of the old one (Existing user )..how to display the session value correctly…
thank you
what does basically string which is parameter in it session.setAttribute does?
what is the default time span, tat the session will remain open. if the user kept idle for X time.
What is the value of X time?
not setting it by programming of web.xml etc…
Very Nice Blog.
Thanks alot.
:)