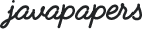
This Java tutorial is to help implement authentication in Java using Facebook OAuth Login API. We will be using Java and a JSON parser API and other than that we will not use any third-party component. Facebook is not providing any sdk for Java client.
Apart from Spring Social I couldn’t find any reputable Java SDK for Facebook integration. With a Json parser we can handle everything ourselves. In this tutorial, we will see how to use Facebook to implement an authentication in a custom Java application and get Facebook profile data like name, email and gender.
We need to create a web application in Facebook. After logging in to https://developers.facebook.com/ under Apps menu click “Create a New App”
We need to specify the application callback url in the FB settings. This will be used by the FB server on authentication to hand back control to our application.
There is a lot of discussion about how Facebook will directly call our “localhost” as it will not be visible to it. Facebook will never call the application URL directly. A request will be sent back as response to the client browser with the callback url. The browser does the request. A sequence diagram given below explains the flow of control in authentication using Facebook OAuth.
Screen shot shown in the start of this tutorial is the login page in our application and following is the code.
<%@page import="com.javapapers.java.social.facebook.FBConnection"%> <%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <% FBConnection fbConnection = new FBConnection(); %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Java Facebook Login</title> </head> <body style="text-align: center; margin: 0 auto;"> <div style="margin: 0 auto; background-image: url(./img/fbloginbckgrnd.jpg); height: 360px; width: 610px;"> <a href="<%=fbConnection.getFBAuthUrl()%>"> <img style="margin-top: 138px;" src="./img/facebookloginbutton.png" /> </a> </div> </body> </html>
Following is the page the Facebook issues a redirect request to the browser.
package com.javapapers.java.social.facebook; import java.io.IOException; import java.util.Map; import javax.servlet.ServletException; import javax.servlet.ServletOutputStream; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class MainMenu extends HttpServlet { private static final long serialVersionUID = 1L; private String code=""; public void service(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException { code = req.getParameter("code"); if (code == null || code.equals("")) { throw new RuntimeException( "ERROR: Didn't get code parameter in callback."); } FBConnection fbConnection = new FBConnection(); String accessToken = fbConnection.getAccessToken(code); FBGraph fbGraph = new FBGraph(accessToken); String graph = fbGraph.getFBGraph(); Map<String, String> fbProfileData = fbGraph.getGraphData(graph); ServletOutputStream out = res.getOutputStream(); out.println("<h1>Facebook Login using Java</h1>"); out.println("<h2>Application Main Menu</h2>"); out.println("<div>Welcome "+fbProfileData.get("first_name")); out.println("<div>Your Email: "+fbProfileData.get("email")); out.println("<div>You are "+fbProfileData.get("gender")); } }
package com.javapapers.java.social.facebook; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.io.UnsupportedEncodingException; import java.net.MalformedURLException; import java.net.URL; import java.net.URLConnection; import java.net.URLEncoder; public class FBConnection { public static final String FB_APP_ID = "234231262685378"; public static final String FB_APP_SECRET = "y2a73dede3n49uid13502f5ab8cdt390"; public static final String REDIRECT_URI = "http://localhost:8080/Facebook_Login/fbhome"; static String accessToken = ""; public String getFBAuthUrl() { String fbLoginUrl = ""; try { fbLoginUrl = "http://www.facebook.com/dialog/oauth?" + "client_id=" + FBConnection.FB_APP_ID + "&redirect_uri=" + URLEncoder.encode(FBConnection.REDIRECT_URI, "UTF-8") + "&scope=email"; } catch (UnsupportedEncodingException e) { e.printStackTrace(); } return fbLoginUrl; } public String getFBGraphUrl(String code) { String fbGraphUrl = ""; try { fbGraphUrl = "https://graph.facebook.com/oauth/access_token?" + "client_id=" + FBConnection.FB_APP_ID + "&redirect_uri=" + URLEncoder.encode(FBConnection.REDIRECT_URI, "UTF-8") + "&client_secret=" + FB_APP_SECRET + "&code=" + code; } catch (UnsupportedEncodingException e) { e.printStackTrace(); } return fbGraphUrl; } public String getAccessToken(String code) { if ("".equals(accessToken)) { URL fbGraphURL; try { fbGraphURL = new URL(getFBGraphUrl(code)); } catch (MalformedURLException e) { e.printStackTrace(); throw new RuntimeException("Invalid code received " + e); } URLConnection fbConnection; StringBuffer b = null; try { fbConnection = fbGraphURL.openConnection(); BufferedReader in; in = new BufferedReader(new InputStreamReader( fbConnection.getInputStream())); String inputLine; b = new StringBuffer(); while ((inputLine = in.readLine()) != null) b.append(inputLine + "\n"); in.close(); } catch (IOException e) { e.printStackTrace(); throw new RuntimeException("Unable to connect with Facebook " + e); } accessToken = b.toString(); if (accessToken.startsWith("{")) { throw new RuntimeException("ERROR: Access Token Invalid: " + accessToken); } } return accessToken; } }
package com.javapapers.java.social.facebook; import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.URL; import java.net.URLConnection; import java.util.HashMap; import java.util.Map; import org.json.JSONException; import org.json.JSONObject; public class FBGraph { private String accessToken; public FBGraph(String accessToken) { this.accessToken = accessToken; } public String getFBGraph() { String graph = null; try { String g = "https://graph.facebook.com/me?" + accessToken; URL u = new URL(g); URLConnection c = u.openConnection(); BufferedReader in = new BufferedReader(new InputStreamReader( c.getInputStream())); String inputLine; StringBuffer b = new StringBuffer(); while ((inputLine = in.readLine()) != null) b.append(inputLine + "\n"); in.close(); graph = b.toString(); System.out.println(graph); } catch (Exception e) { e.printStackTrace(); throw new RuntimeException("ERROR in getting FB graph data. " + e); } return graph; } public MapgetGraphData(String fbGraph) { Map fbProfile = new HashMap (); try { JSONObject json = new JSONObject(fbGraph); fbProfile.put("id", json.getString("id")); fbProfile.put("first_name", json.getString("first_name")); if (json.has("email")) fbProfile.put("email", json.getString("email")); if (json.has("gender")) fbProfile.put("gender", json.getString("gender")); } catch (JSONException e) { e.printStackTrace(); throw new RuntimeException("ERROR in parsing FB graph data. " + e); } return fbProfile; } }
Comments are closed for "Java Facebook Login with OAuth Authentication".
Sound interesting. But did u check Apache Oltu – oauth protocol implementation in java.
HI Joe, this post is nice but when i am trying to login to facebook by using ur application. I am getting error like –
java.io.IOException: Server returned HTTP response code: 400 for URL: graph.facebook.com/me?access_token=XXXXXX&expires=XXXXXXXXXXX
at sun.net.www.protocol.http.HttpURLConnection.getInputStream(Unknown Source)
at sun.net.www.protocol.https.HttpsURLConnectionImpl.getInputStream(Unknown Source)
…
Jul 17, 2014 4:52:17 PM org.apache.catalina.core.StandardWrapperValve invoke
SEVERE: Servlet.service() for servlet [MainMenu] in context with path [/Facebook_Login] threw exception
java.lang.RuntimeException: ERROR in getting FB graph data. java.io.IOException: Server returned HTTP response code: 400 for URL: graph.facebook.com/me?access_token=XXXXXX&expires=XXXXXXXXXXX
at com.javapapers.java.social.facebook.FBGraph.getFBGraph(FBGraph.java:38)
at com.javapapers.java.social.facebook.MainMenu.service(MainMenu.java:28)
…
can u help me out from this problem.
No, I have tried Apache Oltu yet. Will try soon and post a tutorial on it.
Subash,
You have almost got there. You are getting error at the last step, when you get profile data.
Comment email and gender. Get only the first_name and see if it works.
This is really very nice and in details.
Helped me a lot. Thank you
Thanks Joe .. Good Stuff :)
Nice one sir…
Thanks Firoz.
Welcome Sandeep.
Very nice article on with facebook integration using oauth
Very good article
Hi Joe,
I was trying your application.I have added servlet-api jar and running with java 7 and tomcat 7 .it is giving 404 error for fbhome not available.
sorry joe. that was my mistake.its working.
thanks.
hi Joe,
the app is on my account. i am able to login and get the profile data.
but when i login with other account its showing the blank page.
hi Joe,
how to get friendslist from my account please send me that proper working code for getting friendsist ….
thanks,
Very good article. Just added your solution to my customer’s work.
Thanks
Hi joe,
will this work with the jboss7 server?
Yes Mahesh. This should work in Jboss too. This solution is not dependent on any application server.
joe it is giving 404 error fbhome not found
joe
i have done it thanks
joe,
the appid and appsecret i have created work only for one user
how can i get different user detials.
Thank you so much for beautiful help
Hi joe,
I have implement fb login in my app.first i have make jsp file and implement fb login button cde in that jsp. then when user login thruw that jsp it responce me accesscode and profile details.but i have to reverify user profile details in my java code thruw that accessToken.so for that i have use that “https://graph.facebook.com/me?” + accessToken” call but it will give me 400 error. can you tell me way to get user profile details.
how did u do it joe?
*deepak
its good,Its working fine but how to implement it with fb logout button.please provide solution asap.its urgent
this is a very good solution of fb login button
its good,Its working fine but how to implement session .please provide solution. its urgent
hi joe,
its working, thank you so much..