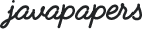
This Hibernate tutorial is part of the “Hibernate Introduction” series. This will help to setup a simple Java project that uses Hibernate ORM and a database to demonstrate insertion of a record. Consider it as a “Hello World” program for Hibernate. Following are the steps,
First two steps are covered in the linked tutorials.
Generally data will be persisted in a database or against some sort of persistence store. Consider the relational database as our persistence store. The application will be using the object oriented programming systems (OOPS). The relational model and the OOPS model are logically different.
We need a mapping process to map the relational schema and object model. For mapping process we need to define mapping rules. That is, for mapping Objects into Relational Tables, we need to define mapping files.
Mapping files can be scattered, where each file represents a single Object or could be all located in one single file. Hibernate requires mapping files to be named like <<file-name>>.hbm.xml. Following sample shows you a simple Message model mapped into Message Table.
We define a new file for mapping all objects that we have and it’s set under resource folder.
<?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD//EN" "http://hibernate.sourceforge.net/hibernate-mapping-2.0.dtd"> <hibernate-mapping> <class name="com.javapapers.data.Message" table="message"> <id name="messageId" column="messageId" type="long"> <generator class="native" /> </id> <property name="message" column="message" type="string" /> </class> </hibernate-mapping>
Download the Eclipse project: Hibernate Example
Now let us consolidate the learning from the “Hibernate Introduction” series.
Hibernation options configuration.
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- Database connection settings --> <property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property> <property name="hibernate.connection.url">jdbc:mysql://192.168.1.102:3306/javapapers</property> <property name="hibernate.connection.username">root</property> <property name="hibernate.connection.password">root</property> <!-- SQL dialect --> <property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property> <!-- Specify session context --> <property name="hibernate.current_session_context_class">org.hibernate.context.internal.ThreadLocalSessionContext</property> <!-- Referring Mapping File --> <mapping resource="domain-classes.hbm.xml"/> </session-factory> </hibernate-configuration>
A simple Java program that
ServiceRegistry
to set options SessionFactory
, Session
package com.javapapers; import java.io.FileNotFoundException; import java.io.IOException; import java.util.Properties; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.boot.registry.StandardServiceRegistryBuilder; import org.hibernate.cfg.Configuration; import org.hibernate.service.ServiceRegistry; import com.javapapers.data.Message; public class App { public static void main(String[] args) throws FileNotFoundException, IOException{ configureUsingHibernateConfigXMLFile(); } public static void configureUsingHibernateConfigXMLFile(){ // Create configuration instance Configuration configuration = new Configuration(); // Pass hibernate configuration file configuration.configure("hibernate.cfg.xml"); // Since version 4.x, service registry is being used ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder(). applySettings(configuration.getProperties()).build(); // Create session factory instance SessionFactory factory = configuration.buildSessionFactory(serviceRegistry); // Get current session Session session = factory.getCurrentSession(); // Begin transaction session.getTransaction().begin(); // Print out all required information System.out.println("Session Is Opened :: "+session.isOpen()); System.out.println("Session Is Connected :: "+session.isConnected()); // Create message object Message message = new Message(); message.setMessage("Hello Javapapers !"); // Save session.save(message); // Commit transaction session.getTransaction().commit(); System.exit(0); } public static void configureUsingHibernatePropertiesFile() throws IOException{ // Create configuration instance Configuration configuration = new Configuration(); // Create properties file Properties properties = new Properties(); properties.load(Thread.currentThread().getContextClassLoader().getResourceAsStream("hibernate.properties")); // Pass hibernate properties file configuration.setProperties(properties); // Since version 4.x, service registry is being used ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder(). applySettings(configuration.getProperties()).build(); // Create session factory instance SessionFactory factory = configuration.buildSessionFactory(serviceRegistry); // Get current session Session session = factory.getCurrentSession(); // Begin transaction session.getTransaction().begin(); // Print out all required information System.out.println("Session Is Opened :: "+session.isOpen()); System.out.println("Session Is Connected :: "+session.isConnected()); // Create message object Message message = new Message(); message.setMessage("Hello Javapapers !"); // Save session.save(message); // Commit transaction session.getTransaction().commit(); System.exit(0); } }
If we wouldn’t define a Transaction boundaries (Begin, Commit) or omit declaring of Hibernate Session Context Tag inside hibernate.cfg.xml, we won’t get Message Object saved.
Executing above code results in insertion of a record in message table.
Download the Eclipse project: Hibernate Example
Comments are closed for "Getting Started With Hibernate".
I Was waiting for Hibernate start up. Thank you sir…
Hi Joe,Thank you very much for sharing knowledge on hibernate.This tutorial is very much helpful.please add few more examples and also please explain about HQL,caching in hibernate,transaction management in hibernate.