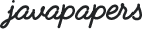
State design pattern provides a mechanism to change behavior of an object based on the object’s state. We can see lot of real world examples for the need of state design pattern. Think of our kitchen mixer, which has a step down motor inside and a control interface. Using that knob we can increase / decrease the speed of the mixer. Based on speed state the behavior changes.
Let us take an example scenario using a mobile. With respect to alerts, a mobile can be in different states. For example, vibration and silent. Based on this alert state, behavior of the mobile changes when an alert is to be done. Which is a suitable scenario for state design pattern. Following class diagram is for this example scenario,
This interface represents the different states involved. All the states should implement this interface.
package com.javapapers.designpatterns.state; public interface MobileAlertState { public void alert(AlertStateContext ctx); }
This class maintains the current state and is the core of the state design pattern. A client should access / run the whole setup through this class.
package com.javapapers.designpatterns.state; public class AlertStateContext { private MobileAlertState currentState; public AlertStateContext() { currentState = new Vibration(); } public void setState(MobileAlertState state) { currentState = state; } public void alert() { currentState.alert(this); } }
Representation of a state implementing the abstract state object.
package com.javapapers.designpatterns.state; public class Vibration implements MobileAlertState { @Override public void alert(AlertStateContext ctx) { System.out.println("vibration..."); } }
Representation of a state implementing the abstract state object.
package com.javapapers.designpatterns.state; public class Silent implements MobileAlertState { @Override public void alert(AlertStateContext ctx) { System.out.println("silent..."); } }
package com.javapapers.designpatterns.state; public class StatePattern { public static void main(String[] args) { AlertStateContext stateContext = new AlertStateContext(); stateContext.alert(); stateContext.alert(); stateContext.setState(new Silent()); stateContext.alert(); stateContext.alert(); stateContext.alert(); } }
vibration... vibration... silent... silent... silent...
Comments are closed for "State Design Pattern".
Good article! Joe, How do you think, is there mathematical abstract model for someone patterns. I mean, for instance the set theory used as abstract model for relational model in RDBMS or something like this.
Great! Thanks a lot for this every nice blog!
really good
Hi Joe,
As usual your posts are very good.
I have side question actually, what tool did you use to draw the class diagram?
Regards,
Kapil
Hi Joe,
State pattern is explained with simplicity, example given is real life and immediately gives us exact thought behind state design pattern after going throuh.
UML notation are awesome and make subject simple to understand.
Regards,
Madan Z
Hi Kapil.
You can add UML designer plugins to eclipse it’s available in marketplace.
Regards
Pratik
Thanks joy, good explanation
I am a beginner and I have a doubt, Why we are taking AlertStateContext obj as parameter of alert method in MobileAlertState interface? Because no way we are using AlertStateContext object in alert method of Vibration or Silent class. Can’t we implement state design pattern by taking alert method without parameter? Kindly reply anyone.
Kindly reply to shaileshsaxena12@gmail.com
Hi Joe,
Nice article. It seems to be similar like that of strategy design pattern. Can you comment on it?
This is in case you need to alter the context’s state from within a MobileAlertState. For instance, you may want the behavior that when the state is silent and 10 alerts are received, the state changes to Vibrate.
In this case you can call
ctx.setState(new Vibrate());
Good example and nice explanation.
Nice as always..
Thanks Joe!!
Thanks for the posts, they are really helpful.
However, if you can incorporate the case-study to accommodate & implement couple of design pattern(at least the ones most commonly used) and also put in latest Java Concepts and learning, that can be extremely helpful at least for those who are intermediate/experts level developers.
This might be little too much but can be really helpful.
Thanks,
Ronak
Why we are taking AlertStateContext obj as parameter of alert method in MobileAlertState interface? Because no way we are using AlertStateContext object in alert method of Vibration or Silent class. Can’t we implement state design pattern by taking alert method without parameter?
This unused parameter passed in to state class from context is just increasing coupling. And if we use it to alter behaviour of context from state class that would increase the coupling manifold as now most of the states will be referring to context + other state classes to set the desired behaviour.
Please comment.