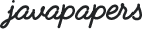
Command design pattern is used to encapsulate a request as an object and pass to an invoker, wherein the invoker does not knows how to service the request but uses the encapsulated command to perform an action.
To understand command design pattern we should understand the associated key terms like client, command, command implementation, invoker, receiver.
It will be easier to understand all the above with an example. Let us think of developing a universal remote. Our UnversalRemote will have only two buttons, one is to power on and another is to mute all associated ConsumerElectronics.
For ConsumerElectronics we will have couple of implementations Television and SoundSystem. Button is a class that is the invoker of an action.
OnCommand is used to switch on a ConsumerElectronics. While instantaiting OnCommand client needs to set the receiver. Here receiver is either Television or SoundSystem. UniversalRemote class will maintain all the receivers and will provide a method to identify the currently active receiver.
When an instance of a invoker is created, a concrete command is passed to it. Invoker does not know how to perform an action. All it does is honoring the agreement with the Command. Invoker calls the execute() method of the set concrete command.
package com.javapapers.designpattern.command; public interface Command { public abstract void execute(); }
package com.javapapers.designpattern.command; public class OnCommand implements Command { private ConsumerElectronics ce; public OnCommand(ConsumerElectronics ce) { this.ce = ce; } public void execute() { ce.on(); } }
package com.javapapers.designpattern.command; import java.util.List; public class MuteAllCommand implements Command { ListceList; public MuteAllCommand(List ceList) { this.ceList = ceList; } @Override public void execute() { for (ConsumerElectronics ce : ceList) { ce.mute(); } } }
package com.javapapers.designpattern.command; public interface ConsumerElectronics { public abstract void on(); public abstract void mute(); }
package com.javapapers.designpattern.command; public class Television implements ConsumerElectronics { public void on() { System.out.println("Television is on!"); } @Override public void mute() { System.out.println("Television is muted!"); } }
package com.javapapers.designpattern.command; public class SoundSystem implements ConsumerElectronics { public void on() { System.out.println("Sound system is on!"); } @Override public void mute() { System.out.println("Sound system is muted!"); } }
package com.javapapers.designpattern.command; public class Button { Command c; public Button(Command c) { this.c = c; } public void click(){ c.execute(); } }
package com.javapapers.designpattern.command; public class UniversalRemote { public static ConsumerElectronics getActiveDevice() { // here we will have a complex electronic circuit :-) // that will maintain current device Television tv = new Television(); return tv; } }
package com.javapapers.designpattern.command; import java.util.ArrayList; import java.util.List; public class DemoCommandPattern { public static void main(String args[]) { // OnCommand is instantiated based on active device supplied by Remote ConsumerElectronics ce = UniversalRemote.getActiveDevice(); OnCommand onCommand = new OnCommand(ce); Button onButton = new Button(onCommand); onButton.click(); Television tv = new Television(); SoundSystem ss = new SoundSystem(); Listall = new ArrayList (); all.add(tv); all.add(ss); MuteAllCommand muteAll = new MuteAllCommand(all); Button muteAllButton = new Button(muteAll); muteAllButton.click(); } }
Implementations of java.lang.Runnable and javax.swing.Action follows command design pattern.
Comments are closed for "Command Design Pattern".
Nice Explanation …………………… Please explain MVP Design Pattern too..
nice
Interesting explanation. Please provide your way explanation to Session Facade. I have red in many articles but not satisfied.
Nice explanation.
Good effort. I appreciate the way you present the content. Well organized.
In my view this article is missing one point that is, its Real Time usage in Projects along with general problems we face while implement it. If it was included in it, then it would be perfect.
I would be more happy to look forward such articles with Real Time situations (when we will choose this pattern) along with general problems.
@Satyprasad,
Thanks.
Sure, your point noted.
Nice Description..
very nice, but does it require to keep the instance variable of the commands same? So that DemoCommandPattern need not write code specific to a command. Please correct me if I am wrong.
Can you explain how Struts Controller(ActionServlet, RequestProcessor) uses the Command Design Pattern
very good description
Great article!!
One doubt..Don’t you think that Button should be part of IniversalRemote class?
Your site has really been a great help.. thanks …
how we can create?
Your tutorials are too good to miss!
I think in the diagram UniversalRemote does not need to have an association for Button.
right?
Rest is excellent. Thanks for sharing
Nice Article
hi,
all
I want to maintain the state of checkboxes across pages.
What happens is when i check one checkbox and then go to say 2nd page then check one checkbox there and when i return to previous page the checked box is again unchecked.
Means the status of the checkbox is not maintained.If it is maintained then it should show checked.
I am using jsps and display tag in jsp for pagination.
Any kind of help would be greatly appreciated.
please help me…………………………
Chandan,
You need pass on flag which pass on the state of the check box when you navigate from one page to another page and vise visa if there are any change in the check box status then flag has to be marked accordingly .Hope this clue will help you
thanks for notes
please sir tell me about the j components
thanks u sir for this note
Hi Joe,
Your articals are really just awsome.
I really am able to call back Einsteins Quote:”Everything should be made as simple as possible, but no simpler.”
The way you explain was really cool.
I am still in between reading your blogs on design patterns..
Can u please give a clarification on how to create dynamic objects along with new instace of a class?
If it is explained in any of the design patterns please ping me that link..i’ll go through it.
What am expecting is something like this.
for(;;){
Pavan p=new Pavan();//Pavan is a class
}
At the end what i want to acheive is to find the number of instances of that particular class.
Thank you,
Pavan Karasala
Is there a way to find objects present in one JVM from another JVM?
Via RMI/JMS/Web service we need to either send the object from other vm or something else…but we cannot jst browse through the objects in another JVM..Please correct me about what i am trying to saying is meaningful…
Regards,
Pavan Karasala
Nice article….
Nice Blog
use a filter.and one map which will keep stock of pages in terms of mapping or path info and check box status.
if any changes happen modify the entryset of map retrieving it from session.
instantiate the map on session listener’s activate session.and put that in session.
really learning a lot from you
Hello Joe,How to read any number of lines from the console in java?
It is easy in c/c++
Regards
Samuel
The article was good, but it keeps some questions unanswered like,
Why do we really create this complex structure ? When UniversalRemote already has access to ConsumerElectronics, why not directly invoke ConsumerElectronics.on() and ConsumerElectronics.muteAll() methods, when the appropriate button is clicked, what is the advantage of creating the intermediate classes like Command ?
If aim is to keep the receiver and invoker of command decoupled from each other, intermediary interface is needed.
Most of the design pattern based on this design principal. “Classes should be open for extension, but closed for modification”.
If I have to add ConsumerElectricals to be switched on from the same Button class, then the scenario that have been suggested by you ask me to modify the existing button implementation which violates above rule. However with the Command patters design would be like this.
interface ConsumerElectrical{
public void switchOn();
}
class CeilingFan implements ConsummerElectricals{
public void switchOn{
System.out.println(“Fan is switched on”);
}
}
class ElectricalsSwitchOnCommand implements Command{
ConsumerElectrical CE;
ElectricalsSwitchOnCommand(ConsumerElectrical CE){
this.CE = CE;
}
public void execute(){
CE.swithcon();
}
}
Public class demo{
public static void main(String [] args){
ConsumerElectronics ce = UniversalRemote().getActiveDevice();
Command C = new onCOmmand(cs);
Button b = new Button(c);
b.click();
ConsumerElectricals cel = getActiveDevice();
Command cl = new ElectricalsSwitchOnCommand();
Button b = newbutton(cl)
b.click();
}
}
Your tutorials are just amazing. Relying on your tutorials rather than my text book :)
Welcom Arun. Enjoy :-)
Hi Joe,
This article is really very useful for the beginners in Java. I really appreciate the effort you put in explaining in more diagrammatic way.
I got a question… why @Override annotation is used only for one method (mute()).
Is it a typo or any other reason ?
public class Television implements ConsumerElectronics {
public void on() {
System.out.println(“Sound system is on!”);
}
@Override
public void mute() {
System.out.println(“Sound system is muted!”);
}
Thanks & Regards,
Sadhu
yes it should be as both method are from
ConsumerElectronics interface.
public class Television implements ConsumerElectronics {
@Override
public void on() {
System.out.println(“Sound system is on!”);
}
@Override
public void mute() {
System.out.println(“Sound system is muted!”);
}
Wonderful articles Joe!
Rakesh added extra value with his comments giving some coded explanation.
Both your work is appreciated
Joe, you deserve a Hip, Hip, Hurray!
By default all the methods in an interface is abstract. Then why in command.java you used
public void abstract execute();
?
sorry its
public abstract void execute();
Isn’t MVC pattern part of the observer design pattern?
This pattern is too complex or confusing… The examples given here are very nice and could understand but I was not able to correlate exactly on how it is implemented in JDK – Runnable and Action implementations.
Here’s an open source command framework for Java: github.com/optimaize/command4j (disclaimer: I’m co-author)
Very Nice description Joe. You site helps me a lot.
nice complex circuit… :-)
all your articles for design pattern are very good and useful. I really appreciate your good work .Thanks a lot for your help
Dear Joe, please include some the use cases of this pattern in real programming problems.