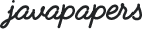
In this tutorial you can learn to write a Java web application to receive emails and display them. Do you know any free or open source email client other than Thunderbird that does an impressive job. Shall we write one? Think about writing our own email client and it will be cool.
This tutorial will help you started. I will write a web client using Java / JSP / HTML / Bootstrap and it will serve as a IMAP client to read emails from IMAP “inbox” folder and display as a list. Along with that, one complete email will be shown in the UI. Have a look at the screen shot of the example application and don’t get deceived from that. The list of emails, one full email is dynamic and other than that is just filler information. You can use this application and enhance it to make a real good email web client.
Already I have written about sending email using GMail SMTP with JavaMail. Use that article and combine it with this application, so that you will get both send and receive email functionality.
I have used a responsive Bootstrap theme for this. You can download this complete example application at the end of this tutorial. Almost all the links are dummy and its just a one page example application and you may enhance it to the next level. May be sometime in future I will try to make this a full fledged email client.
This example project has only two files. One Java file and a JSP page. If you plan to use it in production, you may have to organize and layer it. The UI can be made better with the use of JSP tag libraries. For simplicity, I have used scriplets.
package com.javapapers.java.mail; import java.util.Properties; import javax.mail.Folder; import javax.mail.Message; import javax.mail.MessagingException; import javax.mail.Session; import javax.mail.Store; import javax.mail.URLName; public class MailService { private Session session; private Store store; private Folder folder; // hardcoding protocol and the folder // it can be parameterized and enhanced as required private String protocol = "imaps"; private String file = "INBOX"; public MailService() { } public boolean isLoggedIn() { return store.isConnected(); } /** * to login to the mail host server */ public void login(String host, String username, String password) throws Exception { URLName url = new URLName(protocol, host, 993, file, username, password); if (session == null) { Properties props = null; try { props = System.getProperties(); } catch (SecurityException sex) { props = new Properties(); } session = Session.getInstance(props, null); } store = session.getStore(url); store.connect(); folder = store.getFolder(url); folder.open(Folder.READ_WRITE); } /** * to logout from the mail host server */ public void logout() throws MessagingException { folder.close(false); store.close(); store = null; session = null; } public int getMessageCount() { int messageCount = 0; try { messageCount = folder.getMessageCount(); } catch (MessagingException me) { me.printStackTrace(); } return messageCount; } public Message[] getMessages() throws MessagingException { return folder.getMessages(); } }
I am showing the essential part of the file and you can get the complete file from the download. You need to put your GMail email and password at the place shown. Those things should be parameterized and used accordingly in a serious application.
<% // It is good to Use Tag Library to display dynamic content MailService mailService = new MailService(); mailService.login("imap.gmail.com", "your gmail email here", "your gmail password here"); int messageCount = mailService.getMessageCount(); //just for tutorial purpose if (messageCount > 5) messageCount = 5; Message[] messages = mailService.getMessages(); for (int i = 0; i < messageCount; i++) { String subject = ""; if (messages[i].getSubject() != null) subject = messages[i].getSubject(); Address[] fromAddress = messages[i].getFrom(); %> <div class="mail_list"> <div class="left"> <i class="fa fa-circle"></i> <i class="fa fa-edit"></i> </div> <div class="right"> <h3> <% out.print(fromAddress[0].toString()); %> <small><%=messages[i].getReceivedDate()%></small> </h3> <p><%=subject%> ... </p> </div> </div> <% } %>
Following code displays a mail in the screen. You can choose to display the latest email received.
<% if (messageCount > 0) { String subject = ""; if (messages[0].getSubject() != null) subject = messages[0].getSubject(); Address[] fromAddress = messages[0].getFrom(); Address[] replyToAddress = messages[0].getReplyTo(); %> <div class="inbox-body"> <div class="mail_heading row"> <div class="col-md-8"> <div class="compose-btn"> <a class="btn btn-sm btn-primary" href="mail_compose.html"><i class="fa fa-reply"></i> Reply</a> <button title="" data-placement="top" data-toggle="tooltip" type="button" data-original-title="Print" class="btn btn-sm tooltips"> <i class="fa fa-print"></i> </button> <button title="" data-placement="top" data-toggle="tooltip" data-original-title="Trash" class="btn btn-sm tooltips"> <i class="fa fa-trash-o"></i> </button> </div> </div> <div class="col-md-4 text-right"> <p class="date"><%=messages[0].getReceivedDate()%></p> </div> <div class="col-md-12"> <h4><%=subject%></h4> </div> </div> <div class="sender-info"> <div class="row"> <div class="col-md-12"> <strong> <% out.print(fromAddress[0].toString()); %> </strong> to <strong>me</strong> <a class="sender-dropdown"><i class="fa fa-chevron-down"></i></a> </div> </div> </div> <div class="view-mail"> <% Message message = messages[0]; Object content = message.getContent(); // check for string // then check for multipart if (content instanceof String) { out.println(content); } else if (content instanceof Multipart) { Multipart multiPart = (Multipart) content; int multiPartCount = multiPart.getCount(); for (int i = 0; i < multiPartCount; i++) { BodyPart bodyPart = multiPart.getBodyPart(i); Object o; o = bodyPart.getContent(); if (o instanceof String) { out.println(o); } } } %> <p><%=messages[0].getContent()%></p> </div> <div class="compose-btn pull-left"> <a class="btn btn-sm btn-primary" href="mail_compose.html"><i class="fa fa-reply"></i> Reply</a> <button class="btn btn-sm "> <i class="fa fa-arrow-right"></i> Forward </button> <button title="" data-placement="top" data-toggle="tooltip" type="button" data-original-title="Print" class="btn btn-sm tooltips"> <i class="fa fa-print"></i> </button> <button title="" data-placement="top" data-toggle="tooltip" data-original-title="Trash" class="btn btn-sm tooltips"> <i class="fa fa-trash-o"></i> </button> </div> </div> <% } %>
java-mail-inbox example application
Comments are closed for "Receive Email in Java using JavaMail – GMail IMAP Example".
Hi. This looks pretty simple and straight forward. Thanks you !.
I have one question about mitigating/remediate the Java mail.jar by any other open source jar that should be licencesed by Apache or MPL but not paid one.
I am seeing even Apache Commons email bundle jar also using mail.jar for some dependency classes.
Basically I want get read of mail.jar and place a new bundle and that should not paid version.
[…] API. We will use the email sending part from that article. If you are interested in learning about how to receive email using Java refer the linked […]
This really a nice work. I have tested and get amused. There is a Problem “when i try to retrieve a random email from mail list, it is not working”. Can you please guide how to read random email by clicking on the mail?
Anyway good job.